Getting help
Now that you’ve installed PowerShell and can start it, you need to do stuff with it. You’re going to need help with that. Happily, PowerShell comes with three useful cmdlets built in: Get-Command
, Get-Help
, and Get-Member
. Each of these cmdlets will tell you useful things and give you guidance. Let’s start with Get-Command
.
Get-Command
Get-Command
will give you a list of cmdlets. If you type it in just like that, it will give you a list of around 1,500 cmdlets. When you start installing and writing modules, that list will grow significantly. Scrolling through a list of thousands looking for a likely cmdlet is not that efficient. What you need to do is search the list.
Imagine you need to interrogate a particular process that is running on your client. It is likely that a cmdlet for doing that would include the word process
somewhere. Go ahead and try typing the following into your shell:
Get-Command *process
You should see something like this:
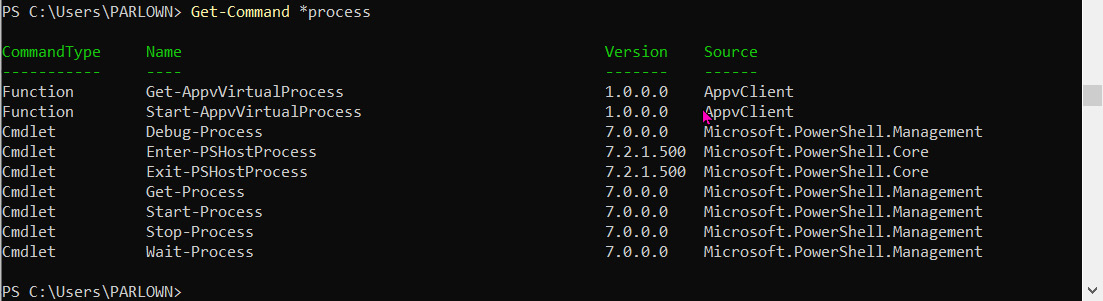
Figure 1.13 – Searching for relevant cmdlets
The cmdlet interprets *process
as a string and searches for cmdlets that end in process
. The *
is a wildcard character. Try running it like this:
Get-Command process
You’ll probably get an error in red.
Some of those cmdlets look a bit cryptic, but there are a few that really stand out – Get-Process
especially. Try running that. You should see quite a long list of processes and some information about them. Let’s look at a process I know you’re currently running: pwsh
. Type the following:
Get-Process pwsh
You should see information for your PowerShell processes:
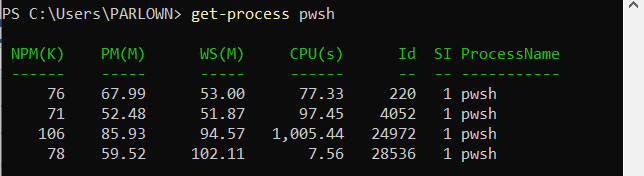
Figure 1.14 – My PowerShell processes
That’s very nice, but what does it all mean? Let’s look at the next of our three helpful cmdlets: Get-Help
.
Get-Help
Running the Get-Help
cmdlet is easy; type Get-Help
followed by the name of the cmdlet you would like help with:
Get-Help Get-Process
You should then see something like this:
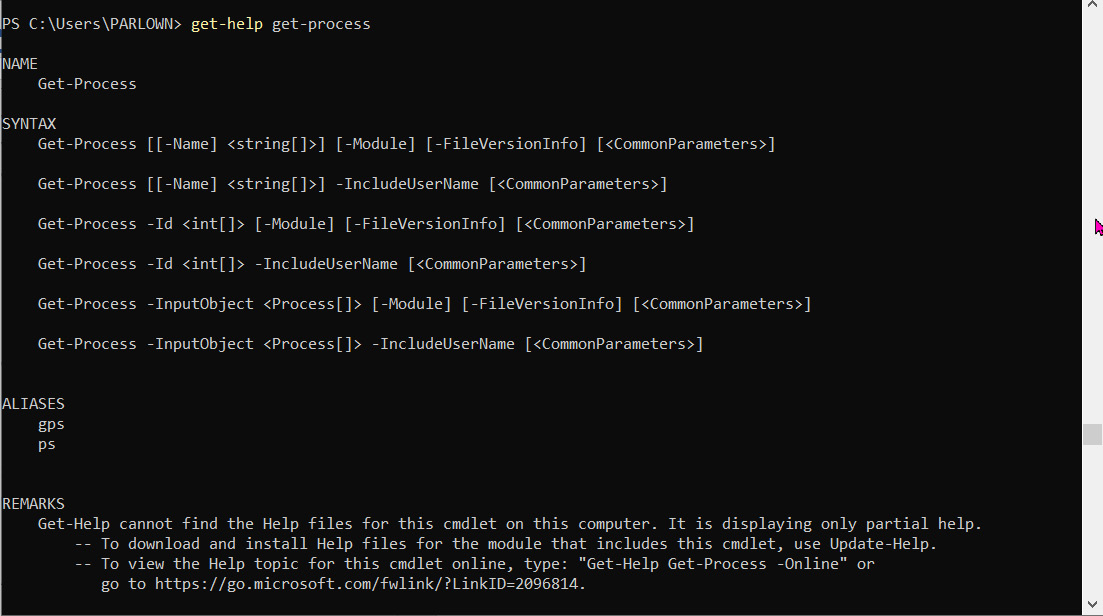
Figure 1.15 – Running Get-Help for the first time
That doesn’t look very helpful. However, if you read the REMARKS
section, there’s an explanation. PowerShell doesn’t ship with full help; you need to download and update it. To update the help files, run the following:
Update-Help
It will take a little while to run, and if you have installed some modules, help files may not be available online for all of them, so you will see red error messages, but after a minute or two, it should finish, and you can then try getting help for Get-Process
again.
Get-Help Get-Process
PowerShell is quite biased toward the en-US culture. Culture here refers to a specific meaning within .NET and associated programs such as PowerShell; it’s equivalent to locale in other systems and refers to the settings specific to a language, number format, and date-time format. If your environment is not set to en-US
, then it may not download all of the relevant help files. If you find you’re not getting everything, try running this line:
Update-Help -UICulture en-US
Then, try again. This is something that particularly affects Linux installations.
You should see a lot more information, including a one-line synopsis and a detailed description. If that’s not enough, then in the REMARKS
section, there will be some other ways of getting even more information about the cmdlet. Try running this:
Get-Help Get-Process -Detailed
You will see more detailed information, including examples of how to use the cmdlet. To see all the information available, use this:
Get-Help Get-Process -Full
You will see everything in the help file, including the extremely useful NOTES
section, which, for this cmdlet, will tell you how to interpret some of the values in the output.
There is one other useful way to run Get-Help
for a cmdlet, using the -
online
parameter:
Get-Help Get-Process -Online
This will produce the web page for the cmdlet in your default browser; it gives the same information as when you use the -
Full
parameter.
About files
Get-Help
doesn’t just help you with cmdlets; you can also get lots of useful information about PowerShell concepts in a special set of files called ABOUT TOPICS
. At the time of writing, there are over 140 of them. There’s lots of information in these files about programming concepts, constructs, and common queries such as logging for both Windows and non-Windows environments. Have a look yourself by running the following:
Get-Help about*
Let’s have a look at one of the files:
Get-Help about_Variables
You should see lots of interesting information about how variables are used in PowerShell.
You can also use full-text search with Get-Help
. If the word you are looking for is not in the help file’s name, then the text of the files will be searched. This takes a little longer but can often be worth it. Try entering the following:
Get-Help *certificate*
Make a mental note of the results you get. Now, try entering certificates
, plural:
Get-Help *certificates*
You’ll get a different set of results. The first set finds help files with certificate
in the filename. When Get-Help
produces the second set, it can’t find any files with certificates
in the name, so it does a full text search. Note that if the search term does occur in a filename, then the full text search won’t be carried out.
The only downside I find with these files is that there is some expectation for you to be knowledgeable about everything in PowerShell except the topic in question. For example, ABOUT_VARIABLES
mentions the scope
variable in the first few paragraphs. Nonetheless, if you need to know how something works quickly, then these files are a great resource.
Get-Member
The final helpful cmdlet we’re going to look at in this chapter is Get-Member
. Earlier in the chapter, we discussed how PowerShell produces objects rather than text output like some shells and scripting languages. Get-Member
allows you to see the members of those objects, their properties, and the methods that may be used on them. It’s easier to show rather than tell, so go ahead and type the following into your shell:
Get-Process | Get-Member
Note
The vertical line between the two cmdlets is called the pipeline character, |. It’s not a lower case L – on my en-GB standard PC keyboard, it’s on the lower left, next to the Z key, and on a standard en-US keyboard, it’s between the Enter and Backspace keys. If your keyboard doesn’t have a solid vertical bar (|), then the broken vertical bar (¦) will work.
What you’re doing here is piping the output of the Get-Process
cmdlet into the next cmdlet as input, which in this case is Get-Member
. We’ll be doing plenty of work on the pipeline in later chapters. Get-Member
will tell you the type of object you’ve given it, in this case a System.Diagnostics.Process
object, and the methods, properties, alias properties, and events associated with that object, like this:
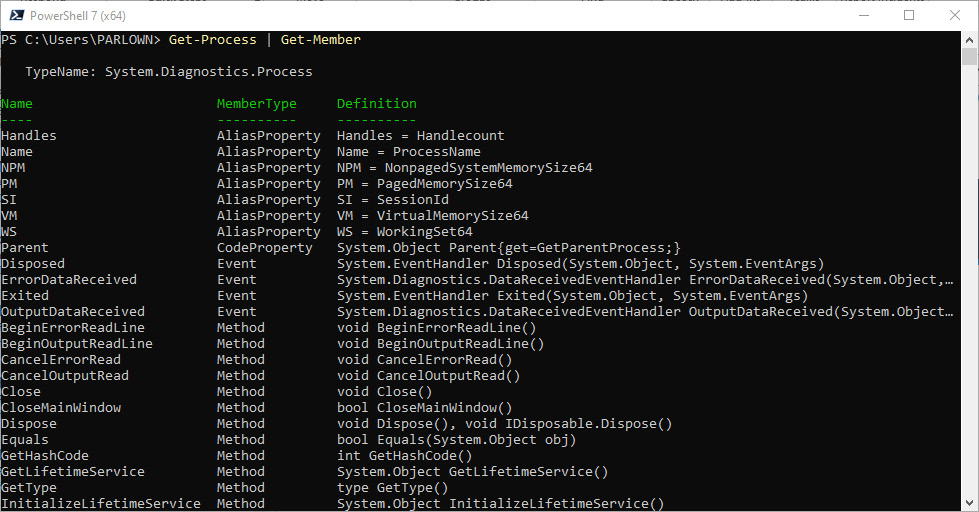
Figure 1.16 – Some of the members of System.Diagnostics.Process
A few pages earlier, in Figure 1.14, we looked at the properties of the pwsh
processes running on your machine. These are the properties that were listed: NPM(K)
, PM(M)
, WS(M)
, CPU(s)
, ID
, SI
, and ProcessName
. As you can now see, that’s Non-Paged Memory (K)
, Paged Memory (M)
, Working Set (M)
, and Session ID
, which are all aliases, so that they can fit nicely into a table on the screen. The CPU(s)
alias is derived in a slightly different way – it’s not set on the object. The ID and the process name are not aliases. M and K are abbreviations for Megabytes and Kilobytes, respectively. That’s a really small subset of all the properties available on the object. As you can see, there are also methods available that can be used to perform operations on the object.
Activity 2
Have a look at the methods. What method might you use to forcibly and immediately stop a process? If you get stuck, have a look at the methods here: https://docs.microsoft.com/en-us/dotnet/api/system.diagnostics.process.
We’ll be returning to Get-Member
more than once in the rest of the book, as it’s such a useful cmdlet.