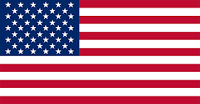

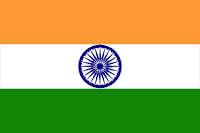
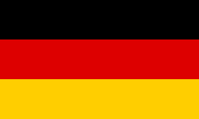
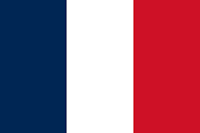

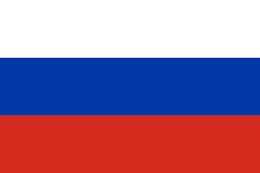
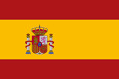



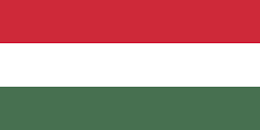
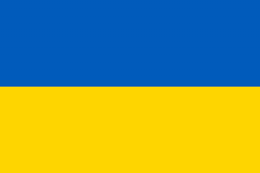
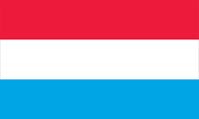

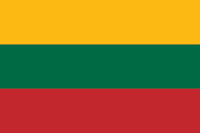

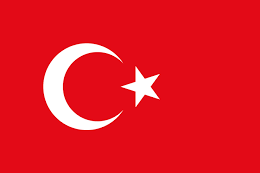


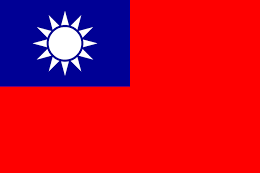
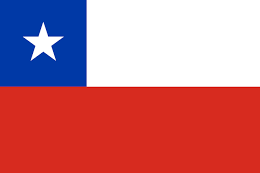

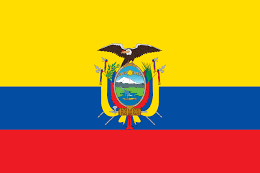
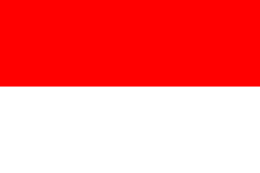
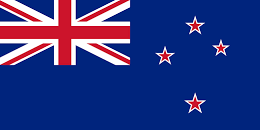
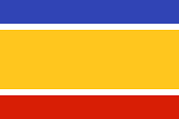
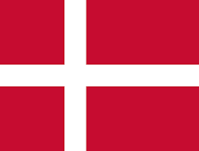
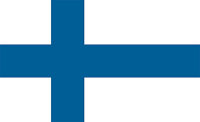


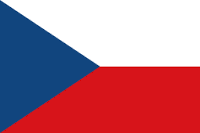
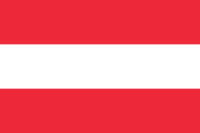
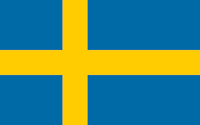
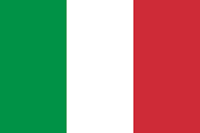
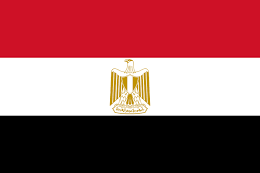

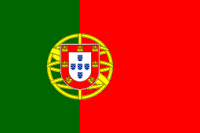
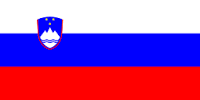

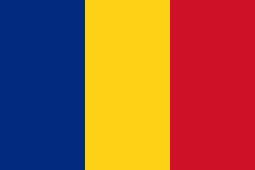
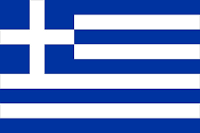


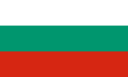
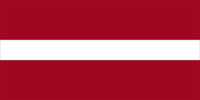
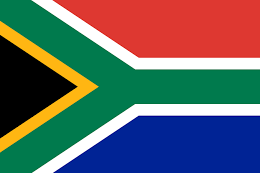
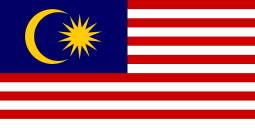



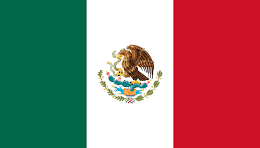
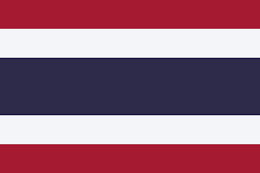
This article is an excerpt from the book, From PHP to Ruby on Rails, by Bernard Pineda. This book will help you adopt the Ruby mindset and get to grips with Ruby-related concepts. You'll learn about setting up your local environment, Ruby syntax, popular frameworks, and more. A language-agnostic approach will help you avoid common pitfalls and start integrating Ruby into your projects.
Just like the libraries we’ve seen so far, Rails is an open source gem. It behaves a little differently than the gems we’ve seen so far as it uses many dependencies and can generate code examples, but at the end of the day, it’s still a gem. This means that we can either install it by itself, or we can include it in a Gemfile. For this section, we will have to divide the process into three separate sections – macOS installation, Windows installation, and Linux installation – as each operating system behaves differently.
The first step of setting up our local environment is to install rbenv. For most Mac installations, brew will simplify this process. Let’s get started with the steps:
1. Let’s open a shell and run the following command:
brew install rbenv
2. This should install the rbenv program. Now, you’ll need to add the following line to your bash profile:
eval "$(rbenv init -)"
3. Once you’ve added this line to your profile, you should activate the change by either opening a new shell or running the following command:
source ~/.bash_profile
Note that this command may differ if you’re using another shell, such as zsh or fish.
4. With rbenv installed, we need to install Ruby 2.6.10 with the following command:
rbenv install 2.6.10
5. Once Ruby 2.6.10 has been installed, we must set the default Ruby version with the following command:
rbenv global 2.6.10
6. Now, we need to install the program to manage gems, called bundler. Let’s install it with the following command:
gem install bundler
With that, our environment is ready for the next steps in this chapter.
If you wish to see more details about this installation, please refer to the following web page: https:// www.digitalocean.com/community/tutorials/how-to-install-ruby-onrails-with-rbenv-on-macos.
Follow these steps to install Ruby on Rails on Windows:
1. To set up our local environment, first, we must install Git for Windows. We can download the package from https://gitforwindows.org/.
Once downloaded, we can run the installer; it should open the installer application:
Figure 7.1 – Git installer
You can safely accept the default options unless you want to change any of the specific behavior from Git. At the end of the installation process, you may just deselect all the options of the wizard and move on to the next step:
Figure 7.2 – Git finalized installation
We will also need the Git SDK installed for some dependencies that Ruby on Rails requires.
We can get the installer from https://github.com/git-for-windows/buildextra/releases/tag/git-sdk-1.0.8.
Be careful and select the correct option for your platform (32 or 64 bits). In my case, I had to choose 64 bits, so I downloaded the git-sdk-installer-1.0.8.0-64.7z.exe binary:
Figure 7.3 – Git SDK download
Once this package has been downloaded, run it; we will be asked where we want the Git SDK to be installed. The default option is fine (C:\git-sdk-64):
Figure 7.4 – Git SDK installation location
This package might take a while to complete as it has to download other additional packages but it will do so on its own. Please be patient. Once this package has finished installing the SDK, it will open a Git Bash console, which looks similar to Windows PowerShell. We can close this Git Bash console window and open another Windows PowerShell.
Once we have the new window open, we must type the following command:
new-item -type file -path $profile -force
This command will help us create a Windows PowerShell profile, which will allow us to execute commands every time we open a Windows PowerShell console. Once we’ve run the previous command, we may also close the Windows PowerShell window, and move on to the next step. At this point, we will install rbenv, which allows us to install multiple versions of Ruby. However, this program wasn’t created for Windows, so its installation is a little different than in other operating systems.
Let’s open a browser and go to the rbenv for Windows web page: https://github.com/ ccmywish/rbenv-for-windows.
On that page, we will find instructions on how to install rbenv, which we will do now.
Let’s open a new Windows PowerShell and type the following command:
$env:RBENV_ROOT = "C:\Ruby-on-Windows "
This command will set a special environment variable that will be used for the rbenv installation.
6. Once we’ve run this command, we must download the rest of the required files with the following command:
iwr -useb "https://github.com/ccmywish/rbenv-for-windows/raw/ main/tools/install.ps1" | iex
7. Once this command has finished downloading the files from GitHub, modify the user’s profile with the following command from within the Windows PowerShell:
notepad $profile
This will open the Notepad application and the profile we previously set.
On the rbenv-for-windows web page, we can see what the content of the file should be. Let’s add it with Notepad so that the profile file now looks like this:
$env:RBENV_ROOT = "C:\Ruby-on-Windows"
& "$env:RBENV_ROOT\rbenv\bin\rbenv.ps1" init
Save and close Notepad, and close all Windows PowerShell windows that we may have open. We should open a new Windows PowerShell to make these changes take effect. As this is the first time rbenv is running, our console will automatically install a default Ruby version. This might take a while and will put our patience to the test. Once the process has finished, we should see an output similar to this one:
Figure 7.5 – rbenv post-installation script
Now, we are ready to install other versions of Ruby. For Ruby on Rails 5, we will install Ruby 2.6.10.
Let’s install it by running the following command on the same Windows Powershell window that we just opened:
rbenv install 2.6.10
The program will ask us whether we want to install the Lite version or the Full version. Choose the Full version. Once again, this might take a while, so please be patient.
Once this command has finished running, we must set this Ruby version for our whole system.
We can do this by running the following command:
rbenv global 2.6.10
To confirm that this version of Ruby has been installed and enabled, use the following command:
ruby --version
This should give us the following output:
ruby 2.6.10-1 (set by C: \Ruby-on-Windows \global.txt)
12. Ruby needs a program called bundler to manage all the dependencies on our system. So, let’s install this program with the following command:
gem install bundler
13. Once this gem has been installed, we must update the RubyGem system with the following command:
gem update –-system 3.2.3
This command will also take a while to compute, but once it’s finished, we will be ready to use Ruby on Rails on Windows.
Next, let’s see the steps for installing Ruby on Rails on Linux.
For Ubuntu and Debian Linux distributions, we must also install rbenv and the dependencies necessary for Ruby on Rails to run correctly:
1. Let’s start by opening a terminal and running the following command:
sudo apt update
2. Once this command has finished updating apt, we must install our dependencies for Ruby, Ruby on Rails, and some gems that require compiling. We’ll do so by running the following command:
sudo apt install git curl libssl-dev libreadline-dev zlib1gdev autoconf bison build-essential libyaml-dev libreadline-dev libncurses5-dev libffi-dev libgdbm-dev pkg-config sqlite3 nodejs
This command might take a while. Once it has finished running, we can install rbenv with the following command:
curl -fsSL https://github.com/rbenv/rbenv-installer/raw/HEAD/ bin/rbenv-installer | bash
We should add rbenv to our $PATH. Let’s do so by running the following command:
echo 'export PATH="$HOME/.rbenv/bin:$PATH"' >> ~/.bashrc
Now, let’s add the initialize command for rbenv to our bash profile with the following command:
echo 'eval "$(rbenv init -)"' >> ~/.bashrc
Next, run the bash profile with the following command:
source ~/.bashrc
7. This command will (among other things) make the rbenv executable available to us. Now, we can install Ruby 2.6.10 on our system with the following command:
rbenv install 2.6.10
This command might take a little while as it installs openssl and that process will take some time. Once this command has finished installing Ruby 2.6.10, we need to set it as the default Ruby version for the whole machine. We can do so by running the following command:
rbenv global 2.6.10
We can confirm that this version of Ruby has been installed by running the following command:
ruby --version
This will result in the following output:
ruby 2.6.10p210 (2022-04-12 revision 67958) [x86_64-linux]
Ruby needs a program called bundler to manage all the dependencies on our system. So, let’s install this program with the following command:
gem install bundler
Once this gem has been installed, we can update the RubyGems system with the following command:
gem update –-system 3.2.3
This command will also take a while to compute, but once it’s finished, we will be ready to use Ruby on Rails on Linux.
For other Linux distributions and other operating systems, please refer to the official Ruby-lang page: https://www.ruby-lang.org/en/documentation/installation/.
In conclusion, installing Ruby on Rails varies across operating systems, but the general process involves setting up a version manager like rbenv, installing Ruby, and then using Bundler to manage gems. Whether you're on macOS, Windows, or Linux, each system has specific steps to ensure Rails and its dependencies run smoothly. By following the detailed instructions for your platform, you'll have your development environment ready for Rails in no time. For further guidance and platform-specific nuances, refer to the official documentation and resources linked throughout this guide.
Bernard Pineda is a seasoned developer with 20 years of web development experience. Proficient in PHP, Ruby, Python, and other backend technologies, he has taught PHP and PHP-based frameworks through video courses on platforms like LinkedIn Learning. His extensive work with Ruby and Ruby on Rails, along with curiosity in frontend development and game development, bring a diverse perspective to this book. Currently working as a Site Reliability Engineer in Silicon Valley, Bernard is always seeking new adventures.