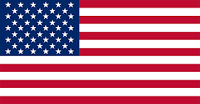

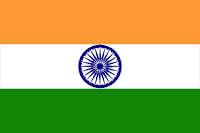
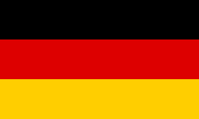
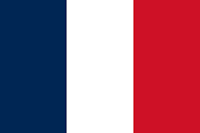

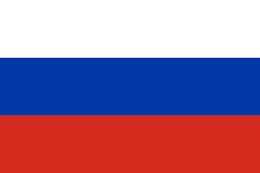
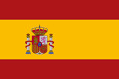



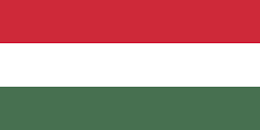
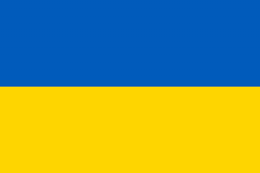
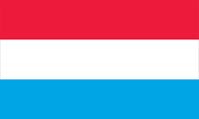

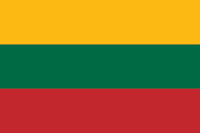

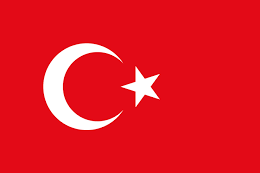


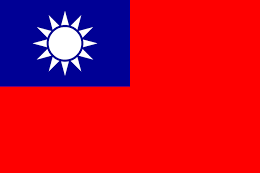
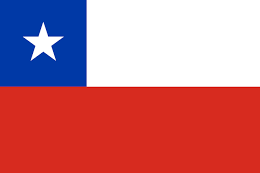

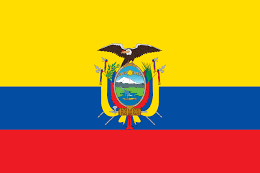
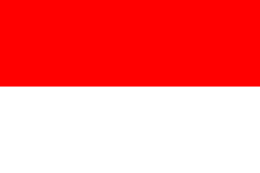
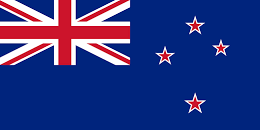
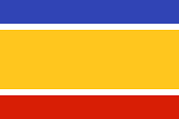
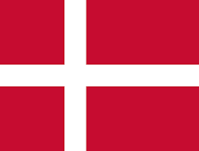
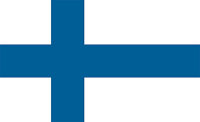


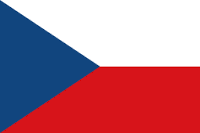
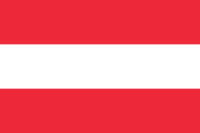
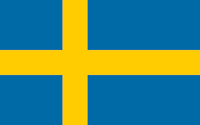
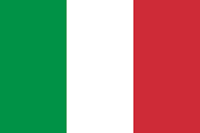
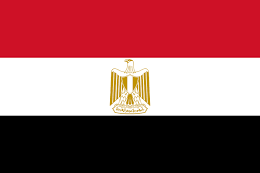

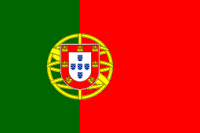
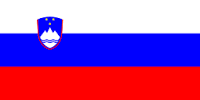

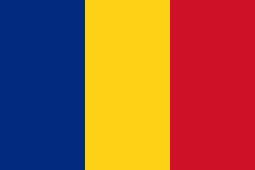
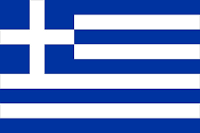


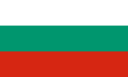
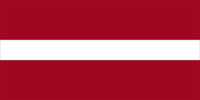
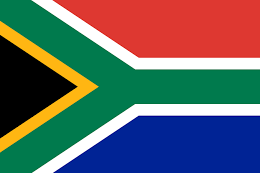
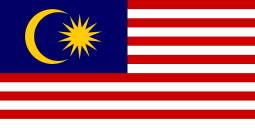



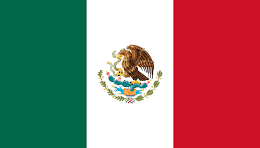
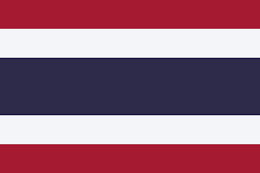
Now that we can invoke Bard from our code using this article, let’s build a news feed application that incorporates Generate AI capability. Not only will it grab the top headlines and stories, but it will also use the Bard API to transform them in a comical way. Note that the Bard API is an unofficial, cutting-edge technology in a rapidly changing area and is not production ready at the time of writing.
The first step is to get the list of news stories. First, let’s use the Bard API to do this.
As seen above, requests to Google Bard yield a natural language response, just like the web interface does. Thus, depending on your use case, you may need to specify a more formal output format so that your program can consume the data.
Our news application could use the prompt, What are the top five news stories today?
This will give us a text response with a numbered or bulleted list of stories. Unless we have a text-only user interface, our application will need to parse this out into individual stories for display.
Thus, to get a computer-readable set of news stories, use a prompt in the form below.
prompt = '''What are the top five news stories today?
Structure the output as a list of xml objects each called story.
Each story element should have child elements called title, content, and url.
'''
Here is an example of what this prompt returned.
Sure, here are the top five news stories today, structured as a list of XML objects with the attributes title, content, and URL:
```xml
<stories>
<story>
<title>US House Votes to Suspend Debt Ceiling and Avoid Default</title>
<content>The US House of Representatives voted on Thursday to suspend the debt ceiling and avoid a default on the country's debt. The vote was 221-212, with all Democrats and 11 Republicans voting in favor. The Senate is expected to vote on the measure later this week.</content>
<url>https://www.cnn.com/2023/06/01/politics/us-debt-ceiling-vote/index.html</url>
</story>
…
</stories>
```
Note the pre-canned introduction at the beginning of the response. Multiple attempts to instruct Bard to omit this and only return the XML data were unsuccessful. However, Bard used a markdown format so extracting the substring from the response is fairly easy. Here is the code we used that leverages the prompt shown earlier.
def get_bard_headlines():
answer = call_bard(prompt)
# We need to filter out the precanned response and get just the xml
# Bard's response beings with something like:
#
# Sure, here are the top five news stories today, structured as a
# list of XML objects with the attributes title, content, and url:
# ```xml
# <news>
# ...
# </news>
# ```
# Get just the json text from the response
delimeter = "```xml"
idx1 = answer.index(delimeter)
idx2 = answer.index("```", idx1 + 6)
# length of substring 1 is added to
# get string from next character
xml_data = answer[idx1 + len(delimeter) + 1: idx2]
article_list = []
# Parse the XML data
root = ET.fromstring(xml_data)
# Iterate over each 'item' element
for item in root.iter('story'):
title = item.find('title').text
content = item.find('content').text
url = item.find('url').text
article_dict = {'title': title, 'content': content, 'url': url}
article_list.append(article_dict)
return article_list
Why use an XML format and not JSON? In our testing with Bard, it did not always create correctly formatted JSON for this use case. It had trouble when the content included single or double quotation marks. See the example below where it incorrectly wrapped text outside of the quotes, resulting in an invalid JSON that caused an exception during parsing.
For this news use case, XML provided reliable results that our application code could easily parse. For other use cases, JSON may work just fine. Be sure to perform testing on different data sets to see what is best for your requirements.
Even if you are not familiar with parsing XML using Python, you can ask Bard to write the code for you:
At this point, we have the basic news stories, but we want to transform them in a comic way. We can either combine the two steps into a single prompt, or we can use a second step to convert the headlines.
One thing to keep in mind is that calls to the Bard API are often slower than typical services. If you use the output from Bard in a high-volume scenario, it may be advantageous to use a background process to get content from Bard and cache that for your web application.
Another option to get fast, reliable headlines is to use the GNews API. It allows up to 100 free queries per day. Create an account to get your API token. The documentation on the site is very good, or you can ask Bard to write the code to call the API. It has a top headlines endpoint with several categories to choose from (world, business, technology, etc.), or you can perform a query to retrieve headlines using arbitrary keyword(s). Here is the code to get the top headlines.
def get_gnews_headlines(category):
url = f"https://gnews.io/api/v4/top-headlines?category={category}&lang=en&country=us&max=10&apikey={gnews_api_key}"
with urllib.request.urlopen(url) as response:
data = json.loads(response.read().decode("utf-8"))
articles = data["articles"]
return articles
Bottle is a lightweight web server framework for Python. It is quick and easy to create web applications. We use the following prompt to have Bard write code for a news feed web page built using the Bottle framework.
Here is a snapshot of the resulting page. It can use some CSS styling, but not bad for a start. The links take you to the underlying news source and the full article.
With the basic news structure in place, Bard can be used for the creative part of transforming the headlines. The prompt and Python functions to do this are shown below. Bard sometimes returns multiple lines of output, so this function only returns the first line of actual output.
reword_prompt = '''Rewrite the following news headline to have a comic twist to it.
Use the comic style of Jerry Seinfeld.
If the story is about really bad news, then do not attempt to rewrite it and simply echo back the headline.
'''
def bard_reword_headline(headline):
answer = call_bard(reword_prompt + headline)
# Split response into lines
lines = answer.split('\n')
# Ignore the first line which is Bard’s canned response
lines = lines[1:]
for line in lines:
if len(line) > 0:
return line.strip("\"")
return "No headlines available"
We then incorporate this into our web server code:
import bottle
import requests
from gnews import get_gnews_headlines
from bard import bard_reword_headline
# Define the Bottle application.
app = bottle.Bottle()
# Define the route to display the news stories.
@app.route("/")
def index():
# Get the news articles from the gnews.io API.
# Category choices are: general, world, nation, business, technology, entertainment,
# sports, science, and health
articles = get_gnews_headlines("technology")
# Reword each headline
for article in articles:
reworded_headline = bard_reword_headline(article['title'])
article['comic_title'] = reworded_headline
# Render the template with the news stories.
return bottle.template("news_template.html", articles=articles)
# Run the Bottle application.
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8080)
Here is the result. I think Bard did a pretty good job.
In conclusion, building an irreverent news feed using Python and the Bard API is a practical and engaging project. By harnessing the power of Python, developers can extract relevant news data and employ creative techniques to inject humor and satire into the feed. The Bard API provides a vast collection of literary quotes that can be seamlessly integrated into the news content, adding a unique and entertaining touch. This project not only demonstrates the versatility of Python but also showcases the potential for innovative and humorous news delivery in the digital age.
Darren Broemmer is an author and software engineer with extensive experience in Big Tech and Fortune 500. He writes on topics at the intersection of technology, science, and innovation.