Creating a project
The first thing to do is to start Qt Creator.
In Qt Creator, you can create a new Qt project via File | New File or Project | Application | Qt Widgets Application | Choose.
The wizard will then guide you through four steps:
- Location: You must choose a project name and a location.
- Kits: Target platforms that your project aims at (Desktop, Android, and so on).
- Details: Base class information and name for the generated class.
- Summary: Allows you to configure your new project as a subproject and automatically add it to a version control system.
Even if all default values can be kept, please at least set a useful project name such as "todo" or "TodoApp." We won't blame you if you want to call it "Untitled" or "Hello world."
Once done, Qt Creator will generate several files that you can see in the Projects hierarchy view:
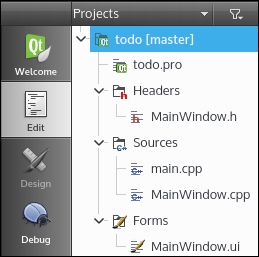
The .pro
file is Qt's configuration project file. As Qt adds specific file formats and C++ keywords, an intermediate build step is performed, parsing all files to generate final files. This process is done by qmake
, an executable from the Qt SDK. It will also generate the final Makefiles for your project.
A basic .pro
file generally contains:
- Qt modules used (
core
,gui
, and so on) - Target name (
todo
,todo.exe
, and so on) - Project template (
app
,lib
, and so on) - Sources, headers, and forms
There are some great features that come with Qt and C++14. This book will showcase them in all its projects. For GCC
and CLANG
compilers, you must add CONFIG += c++14
to the .pro
file to enable C++14 on a Qt project, as shown in the following code:
QT += core gui CONFIG += c++14 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets TARGET = todo TEMPLATE = app SOURCES += main.cpp \ MainWindow.cpp HEADERS += MainWindow.h \ FORMS += MainWindow.ui \
The MainWindow.h
and MainWindow.cpp
files are the headers/sources for the MainWindow
class. These files contain the default GUI generated by the wizard.
The MainWindow.ui
file is your UI design file in XML format. It can be edited more easily with Qt Designer. This tool is a WYSIWYG (What You See Is What You Get) editor that helps you to add and adjust your graphical components (widgets).
Here is the main.cpp
file, with its well-known function:
#include "MainWindow.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; w.show(); return a.exec(); }
As usual, the main.cpp
file contains the program entry point. It will, by default, perform two actions:
- Instantiate and show your main window
- Instantiate a
QApplication
and execute the blocking main event loop
This is the bottom-left toolbar for Qt Creator:
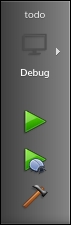
Use it to build and start your todo
application in debug mode:
- Check that the project is in Debug build mode.
- Use the hammer button to build your project.
- Start debugging using the green Play button with a little blue bug.
You will discover a wonderful and beautifully empty window. We will rectify this after explaining how this MainWindow
is constructed:
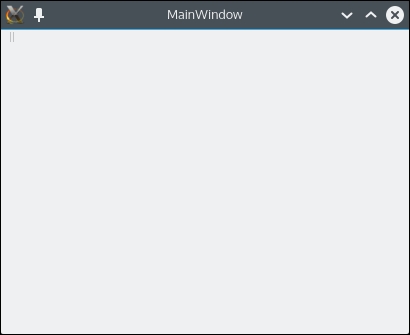
An empty MainWindow screenshot
Tip
Qt tip
- Press Ctrl + B (for Windows/Linux) or Command + B (for Mac) to build your project
- Press F5 (for Windows / Linux) or Command +R (for Mac) to run your application in debug mode