Python doesn't have real private methods, so two underlines at the beginning make a variable and a method private. Let's see a very simple example:
class A:
__amount = 45
def __info(self):
print "I am in Class A"
def hello(self):
print "Amount is ",A.__amount
a = A()
a.hello()
a.__info()
a.__amount
In the preceding program, __info() is the private method and __amount is the private variable. Let's see the output:
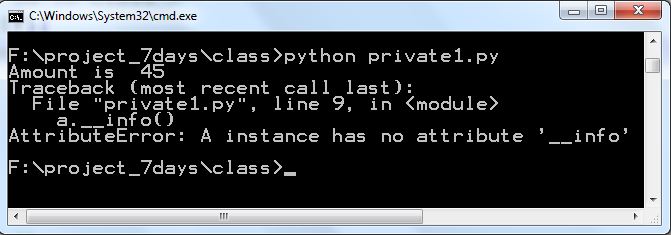
Output of program private1.py
You can see the benefit of the private variable. Outside the class, you cannot access the private method as well as the private variable, but inside the class, you can access the private variables. In the hello() method, the __amount variable can be accessed as shown in the output (Amount is printed).
However, you can access private variables and the private method from outside...