Detecting pupils
We are going to take a different approach here. Pupils are too generic to take the Haar cascade approach. We will also get a sense of how to detect things based on their shape. The following is what the output will look like:
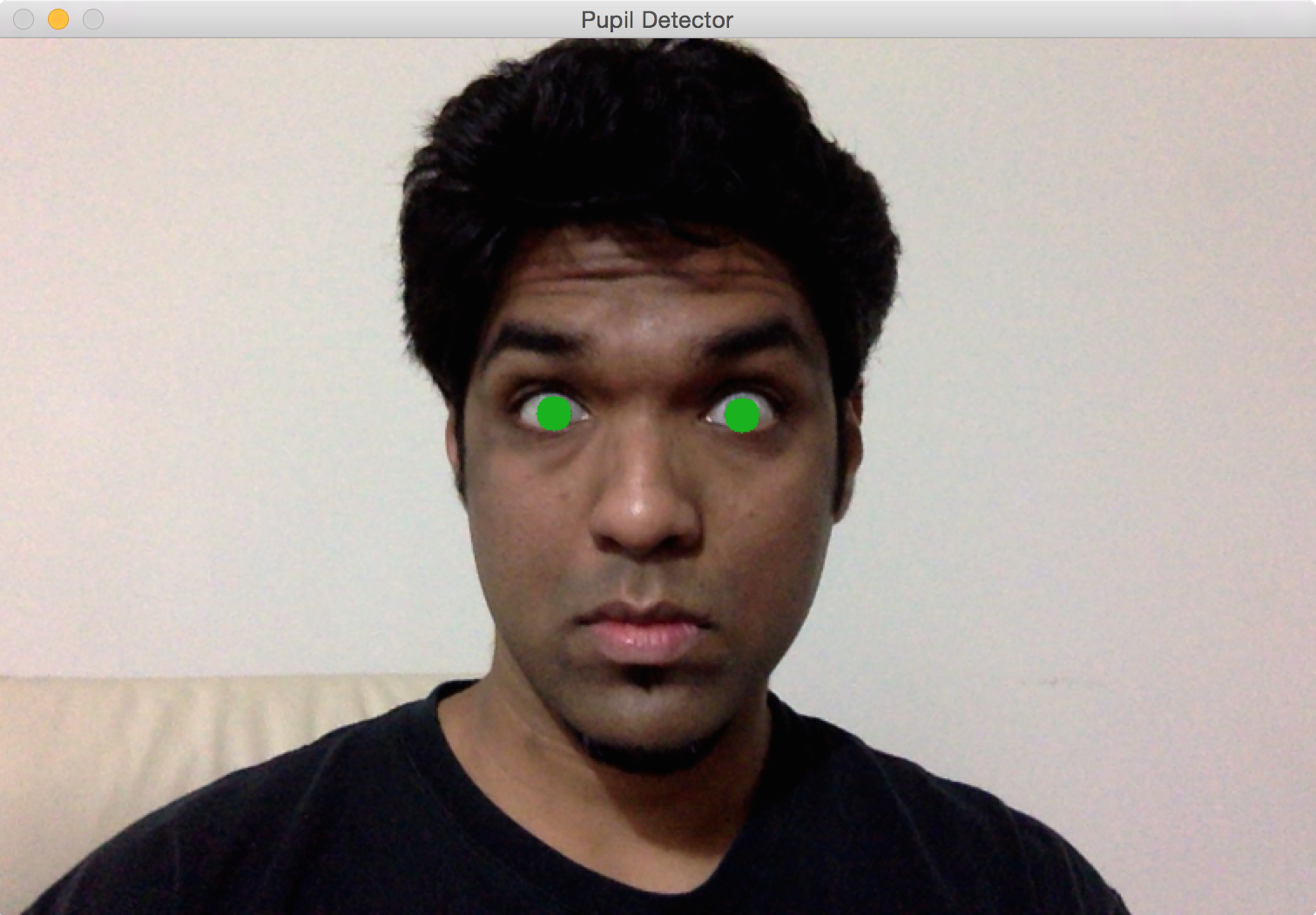
Let's see how to build the pupil detector:
import math import cv2 eye_cascade = cv2.CascadeClassifier('./cascade_files/haarcascade_eye.xml') if eye_cascade.empty(): raise IOError('Unable to load the eye cascade classifier xml file') cap = cv2.VideoCapture(0) ds_factor = 0.5 ret, frame = cap.read() contours = [] while True: ret, frame = cap.read() frame = cv2.resize(frame, None, fx=ds_factor, fy=ds_factor, interpolation=cv2.INTER_AREA) gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) eyes = eye_cascade.detectMultiScale(gray, scaleFactor=1.3, minNeighbors=1) for (x_eye, y_eye, w_eye, h_eye) in eyes: pupil_frame = gray[y_eye:y_eye + h_eye, x_eye:x_eye + w_eye] ret, thresh = cv2.threshold(pupil_frame, 80, 255, cv2.THRESH_BINARY)...