Building a binary tree ADT
A binary tree is a hierarchical data structure whose behavior is similar to a tree, as it contains root and leaves (a node that has no child). The root of a binary tree is the topmost node. Each node can have at most two children, which are referred to as the left child and the right child. A node that has at least one child becomes a parent of its child. A node that has no child is a leaf. Please take a look at the following binary tree:
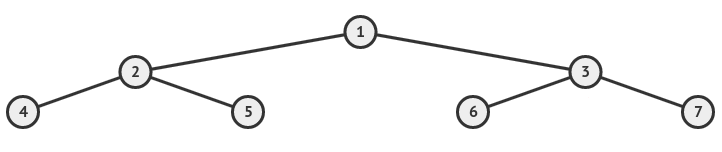
From the preceding binary tree diagram, we can conclude the following:
- The root of the tree is the node of element 1 since it's the topmost node
- The children of element 1 are element 2 and element 3
- The parent of elements 2 and 3 is 1
- There are four leaves in the tree, and they are element 4, element 5, element 6, and element 7 since they have no child
This hierarchical data structure is usually used to store information that forms a hierarchy, such as a file system of a computer.
To implement the binary in code, we need a data structure...