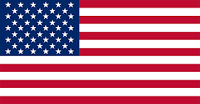

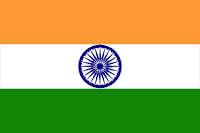
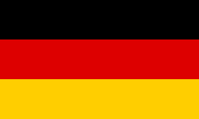
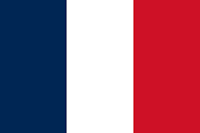

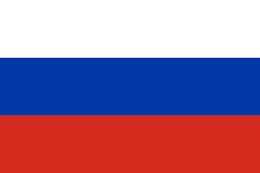
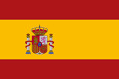



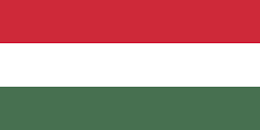

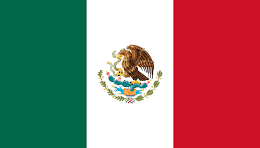
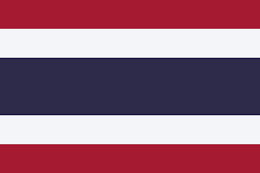
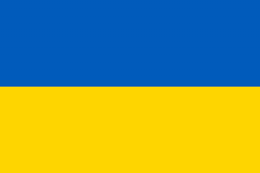
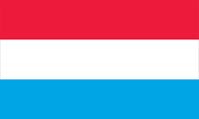

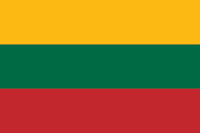

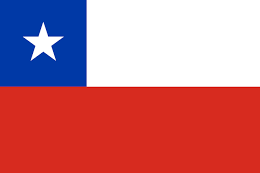

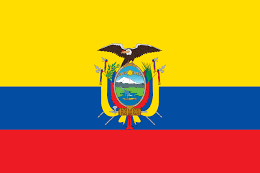

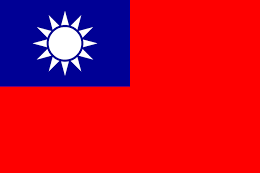

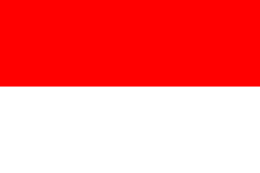
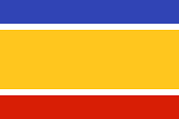
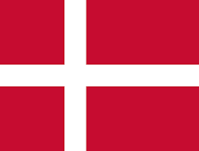
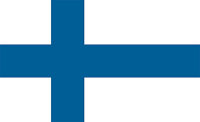


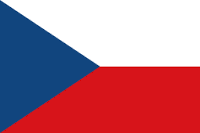
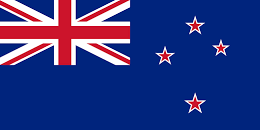
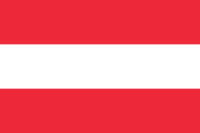
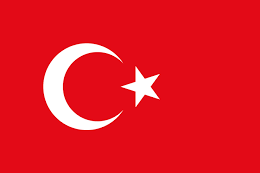
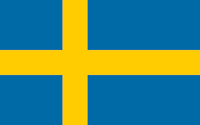
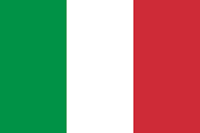
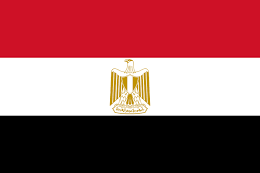

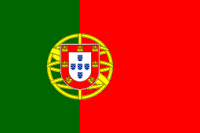
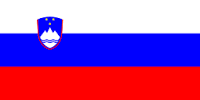

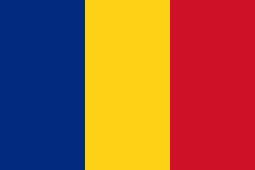
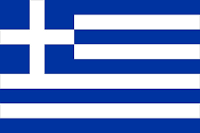

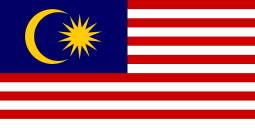
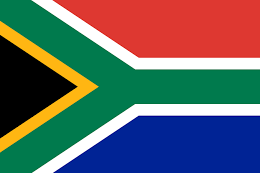

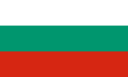
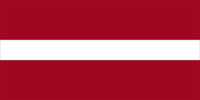


Rumor has it that around 30 million PowerPoint presentations are being created each day. Oh, PowerPoint, the sturdy old friend in the world of presentations. You might ask, why, in this fast-paced, ever-evolving digital world, do people still reach out for PowerPoint? Well, let me break it down for you, because honestly, PowerPoint has still got game! It’s simple, universal (in the realm of presentations), easy to learn and pretty well integrated with the other two Microsoft musketeers (Word & Excel).
So, sure, there are fancier new alternatives out there (like Prezi or Canva), each with their unique features and slick interfaces. But when it comes to a tried-and-true solution for your presentation needs, you might find that, like a well-loved pair of sneakers, PowerPoint fits just right.
And here’s the news: the little AI based automation I am going to show you in this article might actually change your mind about the capabilities of PowerPoint and make you reconsider using much more modern alternatives for your presentations.
Working as a trainer for the last 15 years, I’ve probably seen more PowerPoint than other people do. I used it daily both for studying and teaching, whether for 15-minute presentations or 5-day long courses. I’ve always thought the greatest power of PowerPoint was not in its UI, design features or the number of export formats but rather in the VBA (Visual Basic for Applications) core functionality it shares with the other tools in the Microsoft ecosystem. And yet, so much potential lays unused in this regard.
Oh, did you think that VBA is dead? I’m going to prove you wrong.
A key characteristic for any slide-based presentation is that the viewer only gets a glimpse of the actual knowledge in the slide. It’s more visually appealing that way, but you need a presenter to understand the story and connect the dots. And usually, the presenter needs to use some slide notes for a more cursive and natural presentation. Slide notes are like a secret teleprompter. They give you reminders, help you with the flow of your presentation, and keep you on point. They're your discreet cheat-sheet, making sure you never miss a beat during your presentation.
In this article, I am going to show you how, with just a few lines of code, you can infuse your existing PowerPoint presentations with the ability to automatically generate slide notes based on your slide contents.
My approach might seem a bit crude but it is effective. Especially if your presentation contains general concepts that might fall within the knowledge span of GPT3.5 Turbo from OpenAI (or even GPT-4 if you have an API key). More niche topics, or a slide having just a single bullet of words without too much context, may not produce the best results. Afterall, it’s all automated and no matter how smart the AI model may be, it still needs context to understand the task.
For those who might be unfamiliar with the GPT3.5 Turbo API: this API provides programmatic access to the same advanced language model used by ChatGPT. Its purpose in the script is to explain the concepts in a PowerPoint slide.
So, the basic approach is to create a VBA macro in the presentation that performs the following:
Because it’s a macro, once you’ve created it you can assign a shortcut and repeat the process for each slide.
Alternatively, with a bit of tweaking you could adjust the code to iterate through all the slides and create all slide notes in one go. But keep in mind there is a cost for every API call you make and for a large presentation, the cost might easily spin out of control.
Before
After
Imagine the amount of time you can save by starting with some quick AI-generated draft notes and then reviewing and adding more context instead of having to type everything in from scratch. This productivity hack can save you hours of your time or even help you make sense of presentations created by someone else that lack any explanations or trainer notes.
Some things you need to take care of before you start writing the code:
OpenAI API
key in a local environment variable for secure and efficient access. The code works on the assumption that the API key is stored in your local environment (OPENAI_API_KEY).Developer
tab on your PowerPoint. By default, this tab is not visible. To enable it and be able to create VBA code you need to go to File > Options > Customize Ribbon
and select Main Tabs > Developer > Add
. I know. That’s enough reason to hate PowerPoint. But at least, the good news is you only have to do it once.Developer
Tab and select Visual Basic
.Insert
menu choose Module
.No
, select .PPTM
(Macro enabled presentation) as your file type and save. Make sure you customize the code first to provide the prompt with more context on your specific presentation (see the comments in the code).And that’s it. You’re good to go.
Now, select Developer > Macros
and you should see a new macro called QueryGpt.
Just go to your desired slide and run the macro. Wait for a few seconds and you should have your notes populated with AI’s interpretation of your slide. Be mindful though, the code is simple. There’s no error checking and I haven’t tested it on hundreds of PPTs.
You can download the .BAS source file for this article from here.
Pro Tip: If you want to create an easy shortcut to your macro you can click on the last icon in the very top ribbon menu and select More commands
and then from Choose commands from
select Macros
, choose the QueryGpt macro and Add
. A new button for the macro will be permanently added on your ribbon.
No more talking. Here’s the macro:
Sub QueryGpt()
Dim sld As Slide
Dim shp As Shape
Dim text As String
Dim explanation As String
Dim prompt As String
Dim subject As String
' Extract and format the text from the current slide
Set sld = ActiveWindow.View.Slide
For Each shp In sld.Shapes
If shp.HasTextFrame Then
If shp.TextFrame.HasText Then
text = text & " [" & Replace(shp.TextFrame.TextRange.text, vbCrLf, "/") & "] "
End If
End If
Next shp
text = Replace(Replace(Replace(text, vbCrLf, "\n"), vbCr, "\r"), vbLf, "\n")
' Prepare the prompt and call the function that queries the AI model
subject = "The concept of Public Domain"
prompt = "You are a useful assistant that explains the concepts from a presentation about "
prompt = prompt & subject & ". Explain the following concepts: "
explanation = CallOpenAI(prompt & text)
' Append the AI explanation to the slide notes
sld.NotesPage.Shapes.Placeholders(2).TextFrame.TextRange.InsertAfter vbCrLf & explanation
End Sub
Take note of the variable called subject
.
That is the main topic of your presentation, and you should change that to the subject of your slides.
That way the AI model gets more context about your slides and can provide better descriptions in the notes.
You should be able to achieve better results by adding more details in that variable but consider the maximum context of the model (for GPT 3.5 Turbo that is around 3000 English words). The more you add to your initial input, the more you limit your result’s maximum length because the whole context cannot exceed ~3000 words.
Next, here is the function responsible for making the API call and formatting the resulting answer:
Function CallOpenAI(text As String) As String
Dim httpRequest As Object
Dim responseText As String
Dim contentStartPos As Long
Dim contentEndPos As Long
' Prepare the connection and send the request to the OpenAI API
Set httpRequest = CreateObject("WinHttp.WinHttpRequest.5.1")
httpRequest.Open "POST", "https://api.openai.com/v1/chat/completions", False
httpRequest.setTimeouts 0, 60000, 30000, 120000
httpRequest.setRequestHeader "Content-Type", "application/json"
httpRequest.setRequestHeader "Authorization", "Bearer " & Environ("OPENAI_API_KEY")
httpRequest.Send "{""model"": ""gpt-3.5-turbo"", " _
& """messages"": [{""role"": ""user"", " _
& """content"": """ & text & """}]}"
httpRequest.WaitForResponse
responseText = httpRequest.responseText
' Extract the AI answer from the response string
contentStartPos = InStr(1, responseText, """content"":""") + 11
responseText = Mid(responseText, contentStartPos)
contentEndPos = InStr(1, responseText, """") - 1
responseText = Replace(Mid(responseText, 1, contentEndPos), "\n", vbCrLf)
CallOpenAI = responseText
End Function
All you have to do to add this code to the presentation is to follow steps 3 to 5 above.
Once you’ve created the module and saved the presentation, you’ll have the new macro called QueryGpt.
If you’ve created the shortcut by following the pro tip, you’ll also have a neat way of calling the macro whenever you need to append some slide notes to the current slide.
And there you go: Old-school PowerPoint presentations infused with AI super-powers.
Feels like converting an old Diesel to an EV, doesn’t it?
As with any AI generated content do not forget to check the outputs for accuracy and potential hallucinations. (https://www.packtpub.com/page/4-ways-to-treat-a-hallucinating-AI-with-Prompt-Engineering_). Do not forget that any bias or inaccurate data in the slide content may get amplified in the outputs.
Andrei Gheorghiu is an experienced trainer with a passion for helping learners achieve their maximum potential. He always strives to bring a high level of expertise and empathy to his teaching.
With a background in IT audit, information security, and IT service management, Andrei has delivered training to over 10,000 students across different industries and countries. He is also a Certified Information Systems Security Professional and Certified Information Systems Auditor, with a keen interest in digital domains like Security Management and Artificial Intelligence.
In his free time, Andrei enjoys trail running, photography, video editing and exploring the latest developments in technology.
You can connect with Andrei on: