Debugging in the browser
We can also use our code directly in the browser. There may be times when we want to see the effects of a change, without a build step. In such cases, we can try something like this:
$ npm install --save babel-core $ npm install --save systemjs
These will give us access to a browser-based dependency manager and cross-compiler; that is, we can use unbundled source code in an example HTML file:
<!DOCTYPE html> <html> <head> <script src="/node_modules/babel-core/browser.js"></script> <script src="/node_modules/systemjs/dist/system.js"></script> </head> <body> <div class="react"></div> <script> System.config({ "transpiler": "babel", "map": { "react": "/examples/react/react", "react-dom": "/examples/react/react-dom", "page": "/src/page" }, "defaultJSExtensions": true }); System.import("main"); </script> </body> </html>
This uses the same unprocessed main.js
file as before, but we no longer need to rebuild it after each change to the source code. The System
is a reference to the SystemJS library we just installed through NPM. It takes care of the import statements, loading those dependencies with Ajax requests.
You may notice the references to react
and react-dom
. We import these in main.js
, but where do they come from? Browserify fetches them out of the node_modules
folder. When we skip the Browserify step, we need to let SystemJS know where to find them.
The easiest place to find these files is at https://facebook.github.io/react. Click on the download button, extract the archive, and copy the JS
files in the build
folder to where they are referenced in the HTML page.
The ReactJS website is a great place to download ReactJS, and find documentation about how you can use it:
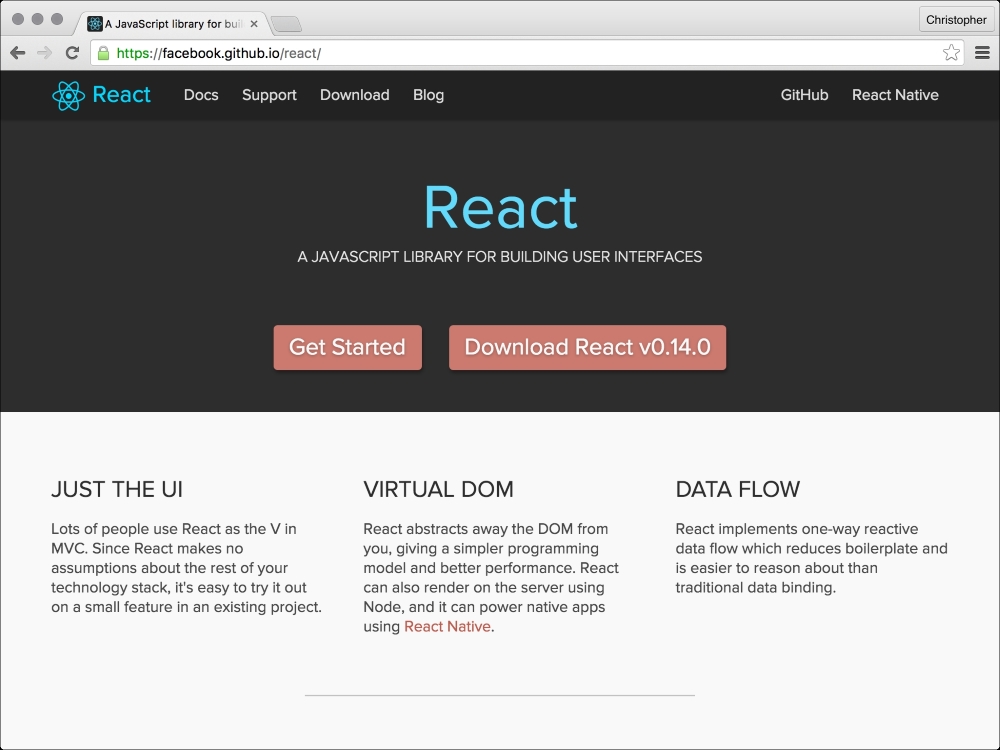