Chapter 1: Introducing ECMAScript 6
Activity 1 – Implementing Generators
You have been tasked with building a simple app that generates numbers in the Fibonacci sequence upon request. The app generates the next number in the sequence for each request and resets the sequence it is given an input. Use a generator to generate the Fibonacci sequence. If a value is passed into the generator, reset the sequence. You may start the Fibonacci sequence at n=1 for simplicity.
To highlight how the generators can be used to build iterative datasets, follow these steps:
Look up the Fibonacci sequence and understand how the next value is calculated.
Create a generator for the Fibonacci sequence.
Inside the generator, set up the default values for
current
andnext
(0, 1) using variablesn2
andn1
.Create an infinite
while
loop.Inside the
while
loop, use theyield
keyword to provide the current value in the sequence and save the return value of the yield statement into a variable calledinput
.If input contains a value, reset the variables
n2
andn1
to their starting values.Inside the
while
loop, calculate the new next value fromcurrent
+next
and save it into the variable next.Otherwise update
n2
to contain the value fromn1
(thenext
value) and setn1
to thenext
value that we calculated at the top of thewhile
loop.
Code:
index.js
function* fibonacci () { let n2 = 0;x let n1 = 1; while ( true ) { let input = yield n2; if ( input ) { n1 = 1; n2 = 0; } else { let next = n1 + n2; [ n1, n2 ] = [ next, n1 ]; } } } let gen = fibonacci();
Snippet 1.87: Implementing a generator
Outcome:
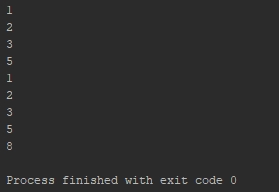
Figure 1.19: Fibonacci sequence with a generator
You have successfully demonstrated how generators can be used to build an iterative data set based on the Fibonacci sequence.