With the Radiobutton widget, you can let the user select among several options. This pattern works well for a relatively small number of mutually exclusive choices.
Creating selections with radio buttons
How to do it...
You can connect multiple Radiobutton instances using a Tkinter variable so that when you click on a non-selected option, it will deselect whatever other option was previously selected.
In the following program, we created three radio buttons for the Red, Green, and Blue options. Each time you click on a radio button, it prints the lowercase name of the corresponding color:
import tkinter as tk COLORS = [("Red", "red"), ("Green", "green"), ("Blue", "blue")] class ChoiceApp(tk.Tk): def __init__(self): super().__init__() self.var = tk.StringVar() self.var.set("red") self.buttons = [self.create_radio(c) for c in COLORS] for button in self.buttons: button.pack(anchor=tk.W, padx=10, pady=5) def create_radio(self, option): text, value = option return tk.Radiobutton(self, text=text, value=value, command=self.print_option, variable=self.var) def print_option(self): print(self.var.get()) if __name__ == "__main__": app = ChoiceApp() app.mainloop()
If you run this script, it will display the application with the Red radio button already selected:
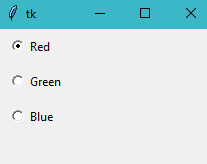
How it works...
To avoid repeating the code of the Radiobutton initialization, we defined a utility method that is called from a list comprehension. We unpacked the values of each tuple of the COLORS list and then passed these local variables as options to Radiobutton. Remember to try to not repeat yourself whenever possible.
Since StringVar is shared among all the Radiobutton instances, they are automatically connected, and we force the user to select only one choice.
There's more...
We set a default value of "red" in our program; however, what would happen if we omit this line, and the value of StringVar does not match any of the radio button values? It will match the default value of the tristatevalue option, which is the empty string. This causes the widget to display in a special "tri-state" or indeterminate mode. Although this option can be modified with the config() method, a better practice is to set a sensible default value so the variable is initialized in a valid state.