Writing a custom HTTP handler
You can create a handler function that takes *gin.Context
as an argument and serves a JSON response with a status code of 200. Then, you can register the handler using the router.Get()
function:
package main import "github.com/gin-gonic/gin" func IndexHandler(c *gin.Context){ Â Â Â c.JSON(200, gin.H{ Â Â Â Â Â Â Â "message": "hello world", Â Â Â }) } func main() { Â Â Â router := gin.Default() Â Â Â router.GET("/", IndexHandler) Â Â Â router.Run() }
Important note
Separating the handler function from the router will be useful in the advanced chapters of this book, when unit testing is tackled.
The biggest strength of the Gin framework is its ability to extract segments from the request URL. Consider the following example:
/users/john /hello/mark
This URL has a dynamic segment:
- Username: Mark, John, Jessica, and so on
You can implement dynamic segments with the following :variable
pattern:
func main() { Â Â Â router := gin.Default() Â Â Â router.GET("/:name", IndexHandler) Â Â Â router.Run() }
The last thing we must do is get the data from the variable. The gin
package comes with the c.Params.ByName()
function, which takes the name of the parameter and returns the value:
func IndexHandler(c *gin.Context) { Â Â Â name := c.Params.ByName("name") Â Â Â c.JSON(200, gin.H{ Â Â Â Â Â Â Â "message": "hello " + name, Â Â Â }) }
Rerun the app with the go run
command. Hit the http://localhost:8080/mohamed
link on your browser; the user will be returned:
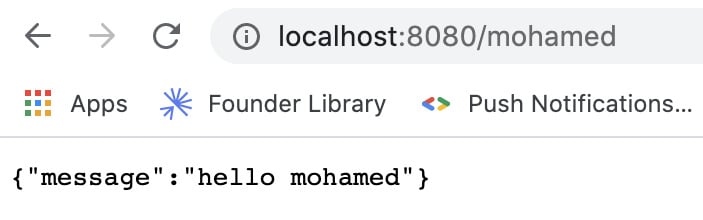
Figure 1.28 – Example of the path parameter
Now, we know that every time we hit the GET /user
route, we get a response of "hello user." If we hit any other route, it should respond with a 404 error message:
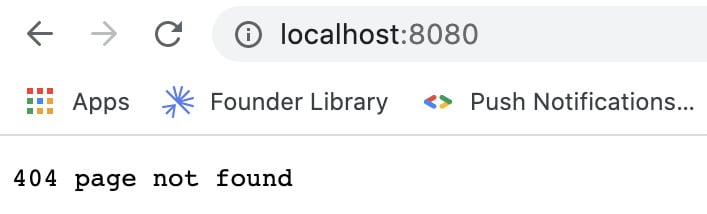
Figure 1.29 – Error handling in Gin
Gin can also handle HTTP requests and responses in XML format. To do so, define a user struct with firstName
and lastName
as attributes. Then, use the c.XML()
method to render XML:
func main() {    router := gin.Default()    router.GET("/", IndexHandler)    router.Run() } type Person struct {      XMLName xml.Name `xml:"person"`      FirstName     string   `xml:"firstName,attr"`      LastName     string   `xml:"lastName,attr"` } func IndexHandler(c *gin.Context) {      c.XML(200, Person{FirstName: "Mohamed",                        LastName: "Labouardy"}) }
Now, rerun the application. If you navigate to http://localhost:8080, the server will return an XML response, as follows:

Figure 1.30 – XML response
Congratulations! At this point, you have a Go programming workspace set up on your local machine, as well as Gin configured. Now, you can begin a coding project!