API design example
I have talked about a lot of the theory of API design, so now I want to look at how you can use Postman to help you out with practically designing an API. API design does not only apply to new APIs that you create. In fact, using the principles of API design when testing an existing API is a great way to find potential threats to the value of that API, but for the sake of understanding this better, let’s look at how you can design an API from scratch. If you understand the principles through this kind of example, you should be able to use them on existing APIs as well.
Case study – Designing an e-commerce API
Let’s imagine that we want to design an API for a very simple e-commerce application. This application has a few products that you can look at. It also allows users to create a profile that they can use when adding items to their cart and purchasing them. The purpose of this API is to expose the data in a way that can be used by both the web and mobile application user interfaces. Your team has just been given this information and you need to come up with an API that will do this.
So, let’s walk through this and see how to apply the design principles we’ve covered. I will start with a simple RAML definition of the API. The first thing we need is to create a file and tell it what version of RAML we are using. I did this by creating a text file in Visual Studio Code (you can use whatever text editor you prefer) called E-Commerce_API-Design.raml
. I then added a reference to the top of the file to let it know that I want to use the 1.0 version of the RAML specification:
#%RAML 1.0
---
I also needed to give the API a title and set up the base URI for this API, so, next, I defined those in the file:
title: E-Commerce API
baseUri: https://api.ecommerce.com/{version}
version: v1
Defining the endpoints
This is a made-up API so that base URI reference does not point to a real website. Notice also how the version has been specified. Now that I have defined the root or base of the API, I can start to design the actual structure and commands that this API will have. I need to start with the purpose of this API, which is to enable both a website and a mobile app. For this case study, I am not going to dive too deep into things like creating personas. However, we do know that this API will be used by the frontend developers to enable what they can show to the users. With a bit of thought, we can assume that they will need to be able to get product information that they can show the users. They will also need to be able to access a user’s account data and allow users to create or modify that data. Finally, they will need to be able to add and remove items from the cart.
With that information in hand about the purpose of the API, I can start to think about the usability and structure of this API. The frontend developers will probably need a /products
endpoint to enable the display of product information. They will also need a /users
endpoint for reading and maintaining the user data and a /carts
endpoint that will allow the developers to add and remove items from a cart.
These endpoints are not the only way that you could lay out this API. For example, you could fold the carts endpoint into the users one. Each cart needs to belong to a user, so you could choose to have the cart be a property of the user if you wanted. It is exactly because there are different ways to lay out an API that we need to consider things like the purpose of the API. In this case, we know that the workflow will require adding and removing items from a cart regularly.
Developers will be thinking about what they need to do in those terms, and so to make them call a “users” endpoint to modify a cart would cause extra data to be returned that they do not need in that context and could also cause some confusion.
Now that I have picked the endpoints I want to use in this API, I will put them into the RAML specification file. That is simply a matter of typing them into the file with a colon at the end of each one:
/products:
/users:
/carts:
Defining the actions
Of course, we need to be able to do something with these endpoints. What actions do you think each of these endpoints should have? Take a second to think about what actions you would use for each of these endpoints.
My initial thought was that we should have the following actions for each endpoint:
/products:
get:
/users:
get:
post:
put:
/carts:
get:
post:
put:
Think about this for a minute, though. If I only have one endpoint, /carts
, for getting information about the carts, I need to get and update information about every cart in the system every time I want to do something with any cart in the system. I need to take a step back here and define this a little better. The endpoints are plural here and should represent collections or lists of objects. I need some way to interact with individual objects in each of these categories:
/products:
get:
/{productId}:
get:
/users:
post:
get:
/{username}:
get:
put:
/carts:
post:
get:
/{cartId}:
get:
put:
Here, I have defined URI parameters that enable users to get information about a particular product, user, or cart. You will notice that the POST
commands stay with the collection endpoint, as sending a POST
action to a collection will add a new object to that collection. I am also allowing API users to get a full list of each of the collections as well if they want.
Adding query parameters
In Chapter 1, API Terminology and Types, you learned about query parameters. Looking at this from the perspective of the users of the API, I think it would be helpful to use a query parameter in the carts endpoint. When a user clicks on a product and wants to add that product to their cart, the developer will already know the product ID based on the item the user clicked. However, the developer might not have information about the cart ID. In this case, they would have to do some sort of search through the carts collection to find the cart that belonged to the current user. I can make that easier for them by creating a query parameter. Once again, I am using the design principles of usability and purpose to help create a good model of how this API should work.
In RAML, I just need to create a query parameter entry under the action that I want to have a query parameter:
/carts:
post:
get:
queryParameter:
username:
/{cartId}:
get:
put:
I have designed the structure of the API using the principles I laid out, and hopefully, you can see how powerful these principles are in helping you narrow down a broad space into something manageable and useful. When it comes to creating a RAML specification for an API, you would still need to specify the individual attributes or properties of each of the endpoints and query parameters that you want to create. I won’t go into all those details here. You can look at the RAML tutorials (https://raml.org/developers/raml-100-tutorial) to learn more about how to do this. I didn’t give enough information about this imaginary API that we are designing to fill out all the properties. We don’t know what information each product or user has, for example. In real life, you would probably get this information based on what is in the database and then build out the examples and attributes based on that.
Using the RAML specification in Postman
This might not be a fully-fledged API specification, but it is enough for us to use in Postman! Click on the Import button in Postman and browse to the .raml
file from this case study. Before importing it, check the View Import Settings section:
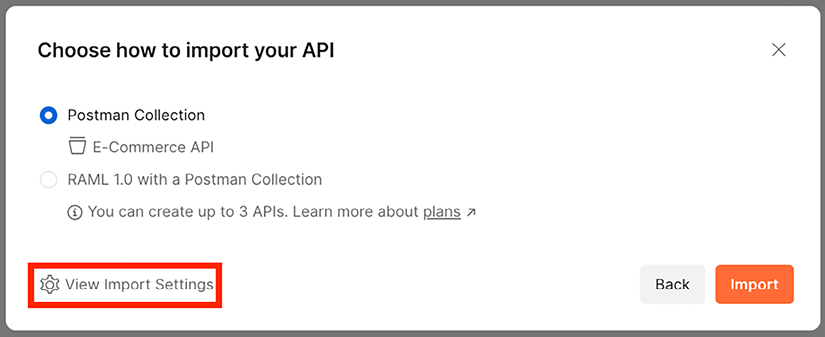
Figure 2.6: Import Settings
Ensure that both the request and response parameter generation options are set to use Schema. Return to the Import panel and click on Import. Postman will parse the specification for you and automatically create a collection that has calls defined for each of the endpoints and action combinations that you created in the specification file. Pretty cool, isn’t it?
Once you have learned about some more Postman features, I will show you how to use these concepts to dive a lot deeper into the design process for APIs. For now, though, I want you to be able to think about how API design intersects with the quality of the API. In fact, I want to give you a little challenge to try out.
Modeling an existing API design
You have been learning how to think through API design. I gave you some principles for this and then walked you through putting the principles into practice in a case study. The case study we just went through involved designing a new API from scratch. Often, though, you will be working with existing APIs. These same design principles can be used to help you find places to improve your existing APIs. In order to learn these principles, you should try this exercise out for yourself. See if you can use these principles on an API that you are currently working on. If the company you are working at does not have an API that you can use, you can, of course, just find a public API to try out this exercise with.
Using the API you have selected, work through the following steps to apply the principles of API design:
- Add each of the endpoints to a RAML file. Make sure to follow the hierarchy of the API in that file.
- Spend a bit of time thinking about what the purpose of the API is and reflecting on whether the structure that you see here fits with that purpose. In what other ways might you design the API? Could you improve the layout to better fit the purpose?
- If you were designing this API, what actions and query parameters would you give to each endpoint? Create a copy of the file and fill it in with what you think you would do with this API.
- In the original file, add the actual actions and query parameters that the API has. How do they compare to the ones that you made in the copy of the file?
If you want, you can import the file into Postman and, as you learn more about testing and other features that you can use in Postman, you will already have a collection of requests ready to use.