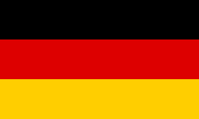




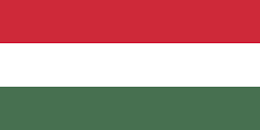

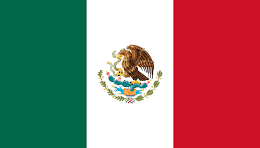
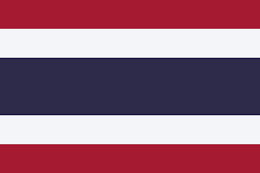
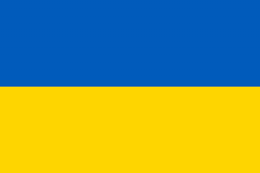
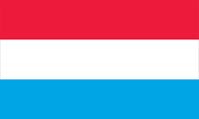

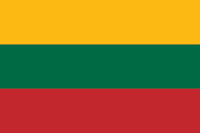

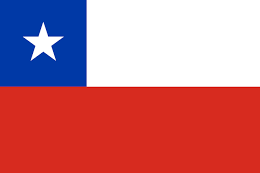
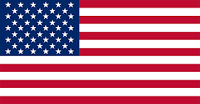

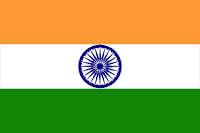
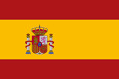

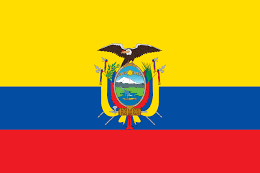

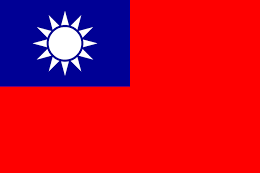

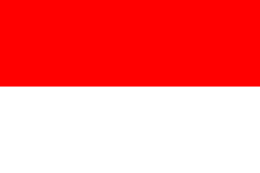
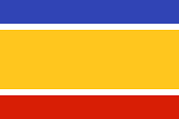
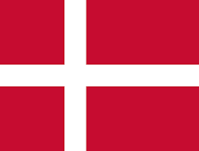
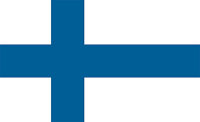


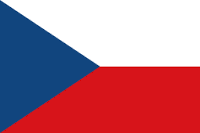
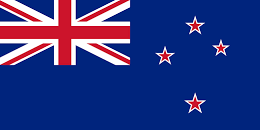
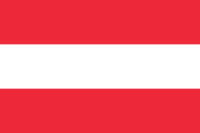
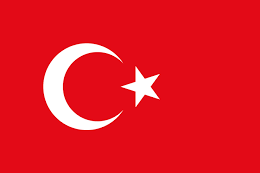
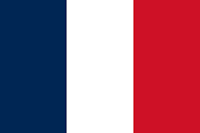
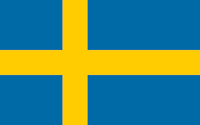
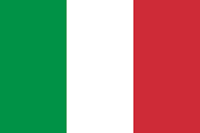
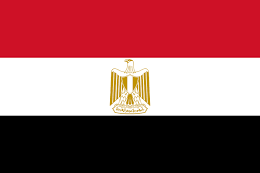

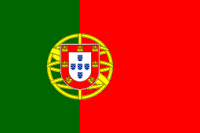
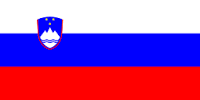

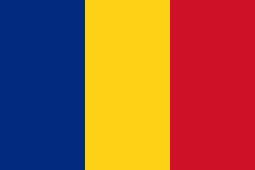
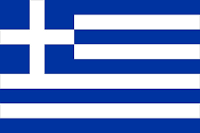

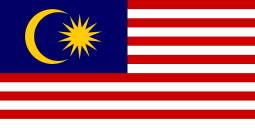
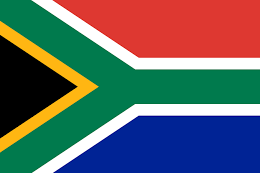

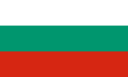
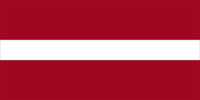


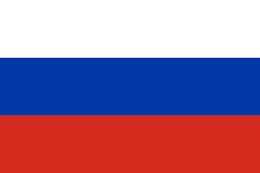
This article is an excerpt from the book, The Ultimate iOS Interview Playbook, by Avi Tsadok. The iOS Interview Guide is an essential book for iOS developers who want to maximize their skills and prepare for the competitive world of interviews on their way to getting their dream job. The book covers all the crucial aspects, from writing a resume to reviewing interview questions, and passing the architecture interview successfully.
In iOS development, data structures are fundamental tools for managing and organizing data. Whether you are preparing for a technical interview or building robust iOS applications, mastering data structures like arrays, dictionaries, and sets is essential. This tutorial will guide you through the essential data structures in Swift, explaining their importance and providing practical examples of how to use them effectively. By the end of this tutorial, you will have a solid understanding of how to work with these data structures, making your code more efficient, modular, and reusable.
Before diving into the tutorial, make sure you have the following prerequisites:
Familiarity with Swift Programming Language: A basic understanding of Swift syntax, including variables, functions, and control flow, is essential for this tutorial.
Xcode Installed: Ensure you have Xcode installed on your Mac. You can download it from the Mac App Store if you haven’t done so already.
Basic Understanding of Object-Oriented Programming: Knowing concepts such as classes and objects will help you better understand the examples provided in this tutorial.
Data structures play a crucial role in iOS development. They allow you to store, manage, and manipulate data efficiently, which is especially important in performance-sensitive applications. Whether you're handling user data, managing app state, or working with APIs, choosing the right data structure can significantly impact your app's performance and scalability.
Swift provides several built-in data structures, including arrays, dictionaries, and sets. Each of these data structures offers unique advantages and is suitable for different use cases. Understanding when and how to use each of them is a key skill for any iOS developer.
Arrays are one of the most commonly used data structures in Swift. They allow you to store ordered collections of elements, making them ideal for tasks that require sequential access to data.
var numbers: [Int] = [1, 2, 3, 4, 5]
This creates an array of integers containing the values 1 through 5. Arrays in Swift are type-safe, meaning you can only store elements of the specified type (in this case, Int).
let arrayWithDuplicates = [1, 2, 3, 3, 4, 5, 5]
let arrayWithNoDuplicates = Array(Set(arrayWithDuplicates))
This approach is efficient and concise, leveraging the unique properties of sets to remove duplicates.
Iterating Over an Array Arrays provide several methods for iterating over their elements. The most common approach is to use a for-in loop:
for number in numbers {
print(number)
}
This loop prints each element in the array to the console. You can also use methods like map, filter, and reduce for more advanced operations on arrays.
A queue is a data structure that follows the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed. Queues are commonly used in scenarios like task scheduling, breadth-first search algorithms, and managing requests in networking.
In Swift, you can implement a basic queue using an array. Here’s an example:
struct Queue<Element> {
private var array: [Element] = []
var isEmpty: Bool {
return array.isEmpty
}
var count: Int {
return array.count
}
mutating func enqueue(_ element: Element) {
array.append(element)
}
mutating func dequeue() -> Element? {
return array.isEmpty ? nil : array.removeFirst()
}
}
In this implementation:
Queues are useful in many scenarios, such as managing tasks in a multi-threaded environment or implementing a breadth-first search algorithm.
Dictionaries are another powerful data structure in Swift. They store data in key-value pairs, allowing you to quickly look up values based on their associated keys. Dictionaries are ideal for tasks where you need fast access to data based on a unique identifier.
var userAges: [String: Int] = ["Alice": 25, "Bob": 30]
In this example, the keys are strings representing user names, and the values are integers representing their ages.
if let age = userAges["Alice"] {
print("Alice is \(age) years old.")
}
userAges["Alice"] = 26
This code snippet retrieves Alice's age and updates it to 26. Dictionaries are highly efficient for lookups, making them a valuable tool when working with large datasets.
userAges["Charlie"] = 22
To remove a key-value pair, use the removeValue(forKey:) method:
userAges.removeValue(forKey: "Bob")
5. Exploring Sets
Sets in Swift are similar to arrays, but with one key difference: they do not allow duplicate elements. Sets are unordered collections of unique elements, making them ideal for tasks like checking membership, ensuring uniqueness, and performing set operations (e.g., union, intersection).
let uniqueNumbers: Set = [1, 2, 3, 4, 5]
Unlike arrays, sets do not maintain the order of elements. However, they are more efficient for operations like checking if an element exists.
let evenNumbers: Set = [2, 4, 6, 8]
let oddNumbers: Set = [1, 3, 5, 7]
let union = evenNumbers.union(oddNumbers) // All unique elements from both sets
let intersection = evenNumbers.intersection([4, 5, 6]) // Elements common to both sets
let difference = evenNumbers.subtracting([4, 6]) // Elements in evenNumbers but not in the other set
6. Understanding the Codable Protocol
The Codable protocol in Swift simplifies encoding and decoding data, making it easier to work with JSON and other data formats. This is especially useful when interacting with web APIs or saving data to disk.
struct Person: Codable {
var name: String
var age: Int
var address: String
}
With Codable, you can easily encode and decode instances of Person using JSONEncoder and JSONDecoder:
let person = Person(name: "Alice", age: 25, address: "123 Main St")
let jsonData = try JSONEncoder().encode(person)
let decodedPerson = try JSONDecoder().decode(Person.self, from: jsonData)
For each code snippet, you should test and verify that the output matches the expected results. For example, when implementing the queue structure, enqueue and dequeue elements to ensure the correct order of processing. Similarly, when working with dictionaries, confirm that you can retrieve, add, and remove key-value pairs correctly.
This tutorial has covered fundamental data structures in Swift, including arrays, dictionaries, and sets, and their practical applications in iOS development. Understanding these data structures will make you a better Swift developer and prepare you for technical interviews and real-world projects.
Avi Tsadok, seasoned iOS developer with a 13-year career, has proven his expertise leading projects for notable companies like Any.do, a top productivity app, and currently at Melio Payments, where he steers the mobile team. Known for his ability to simplify complex tech concepts, Avi has written four books and published 40+ tutorials and articles that enlighten and empower aspiring iOS developers. His voice resonates beyond the page, as he's a recognized public speaker and has conducted numerous interviews with fellow iOS professionals, furthering the field's discourse and development.