Adding rows to a list
Lists are usually used to add, edit, remove, or display content from an existing dataset. In this section, we will go over the process of adding items to an already existing list.
Getting ready
Let's start by creating a new SwiftUI app called AddRowsToList
.
How to do it…
To implement the add functionality, we will enclose the List
view in NavigationView
, and add a button to navigationBarItems
that triggers the add function we will create. The steps are as follows:
- Create a state variable in the
ContentView
struct that holds an array of integers:@State var numbers = [1,2,3,4]
- Add a
NavigationView
component containing aList
view to theContentView
body:NavigationView{ List{ ForEach(self.numbers, id:\.self){ number in Text("\(number)") } } }
- Add a
navigationBarItems
modifier to the list closing brace that contains a button that triggers theaddItemToRow()
function:.navigationBarItems(trailing: Button(action: { self.addItemToRow() }){ Text("Add") })
- Implement the
addItemToRow()
function, which appends a random integer to the numbers array. Place the function within theContentView
struct, immediately after the body variable's closing brace:private func addItemToRow() { self.numbers.append(Int.random(in: 0 ..< 100)) }
- For the beauty and aesthetics, add a
navigationBarTitle
modifier to the end of the list so as to make it display a title at the top of the list:.navigationBarTitle("Number List", displayMode: .inline)
- The resulting code should be as follows:
struct ContentView: View { @State var numbers = [1,2,3,4] var body: some View { NavigationView{ List{ ForEach(self.numbers, id:\.self){ number in Text("\(number)") } }.navigationBarTitle("Number List", displayMode: .inline) .navigationBarItems(trailing: Button("Add", action: addItemToRow)) } } private func addItemToRow() { self.numbers.append(Int.random(in: 0 ..< 100)) } }
The resulting preview should be as follows:
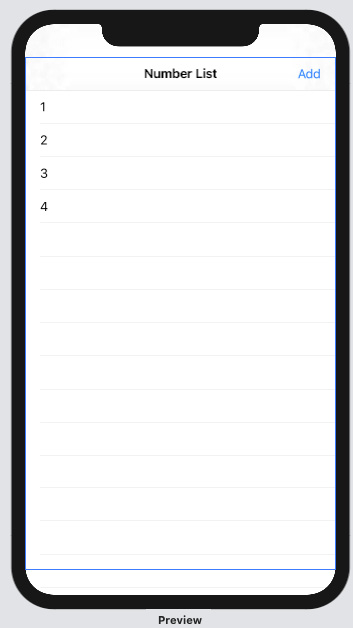
Figure 2.5 – AddRowToList preview
Run the app live preview and admire the work of your own hands!
How it works…
The array of numbers is declared as a @State
variable because we want the view to be refreshed each time the value of the items in the array changes – in this case, each time we add an item to the numbers array.
The .navigationBarTitle("Number List", displayMode: .inline)
modifier adds a title to the list using the .inline display mode
parameter.
The .navigationBarItems(trailing: Button(…)…)
modifier adds a button to the trailing end of the display, which triggers the addItemToRow
function when clicked.
The addItemToRow
function generates a random number in the range 0–99 and appends it to the numbers array.