Building a perceptron
Let's start our neural network adventure with a perceptron. A perceptron is a single neuron that performs all the computation. It is a very simple model, but it forms the basis of building up complex neural networks. Here is what it looks like:
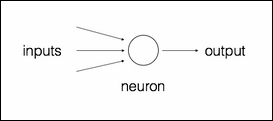
The neuron combines the inputs using different weights, and it then adds a bias value to compute the output. It's a simple linear equation relating input values with the output of the perceptron.
How to do it…
- Create a new Python file, and import the following packages:
import numpy as np import neurolab as nl import matplotlib.pyplot as plt
- Define some input data and their corresponding labels:
# Define input data data = np.array([[0.3, 0.2], [0.1, 0.4], [0.4, 0.6], [0.9, 0.5]]) labels = np.array([[0], [0], [0], [1]])
- Let's plot this data to see where the datapoints are located:
# Plot input data plt.figure() plt.scatter(data[:,0], data[:,1]) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title...