In Kotlin, we can easily add functionality to existing classes through its extension functions. The language provides the ability to add additional functions to classes without modifying the source code. In this section, we will look at how to extend the functionality of classes so that they provide the exact functionality that we are interested in. Kotlin supports extension functions and extension properties.
To create an extension function, we start with the fun keyword, and then we prefix the name of the extension function with the name of the class to be extended (known as the receiver type), followed by the dot operator (represented by the . character).
An example of this is receiverType.customFunctionName().
Consider the following code for 13_ExtensionFunctons.kts:
fun String.myExtendedFunction() = toUpperCase()
println("Kotlin".myExtendedFunction())
Here, myExtendedFunction() is an extension to the existing String class. We added myExtendedFunction() as an extension function and invoked it on a String literal.
The output for the preceding example is as follows:

This can be pictorially represented as follows:
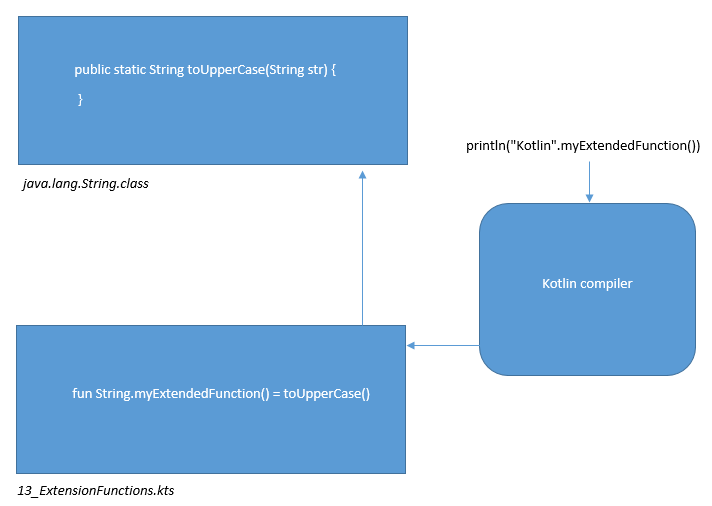
- Extension functions do not get added to the original classes. Instead, new functions are made callable by adding a dot on the receiverType variables.
- Extension functions are resolved during compilation time. They are statically typed. This means that the extension function being invoked is determined by the receiverType of the expression on which the function is invoked, not by the nature of the result of evaluating the expression at runtime.
- The extension functions cannot be overloaded.