Adding sections to a list
In this section, we will create an app that implements a static list with sections. The app will display a partial list of countries grouped by continent.
Getting ready
Let's start by creating a new SwiftUI app in Xcode and name it ListWithSections
.
How to do it…
We will add section views to our list view. We will then add a few countries to each of the sections. Proceed as follows:
- (Optional) open the
ContentView.swift
file and replace theText
view in the body with a navigation view. Wrapping the list in a navigation view allows you to add a title and navigation items to the view:NavigationView { }
- Add a list and section to the navigation view:
List { Section(header: Text("North America")){ Text("USA") Text("Canada") Text("Mexico") Text("Panama") Text("Anguilla") } }
- Add a
listStyle(..)
modifier to the end of the list to change its style from the default plain style toGroupedListStyle()
:List { . . . } .listStyle(GroupedListStyle()) .navigationBarTitle("Continents and Countries", displayMode: .inline)
- (Optional) add a
navigationBarTitle(..)
modifier to the list, just below the list style. Add this section if you included the navigation view in step 1. - Add more sections representing various continents to the navigation view. The resulting
ContentView
struct should look as follows:struct ContentView: View { var body: some View { NavigationView{ List { Section(header: Text("North America")){ Text("USA") Text("Canada") Text("Mexico") Text("Panama") Text("Anguilla") } Section(header: Text("Africa")){ Text("Nigeria") Text("Ghana") Text("Kenya") Text("Senegal") } Section(header: Text("Europe")){ Text("Spain") Text("France") Text("Sweden") Text("Finland") Text("UK") } } .listStyle(GroupedListStyle()) .navigationBarTitle("Continents and Countries", displayMode: .inline) } } }
The canvas preview should now show a list with sections, as follows:
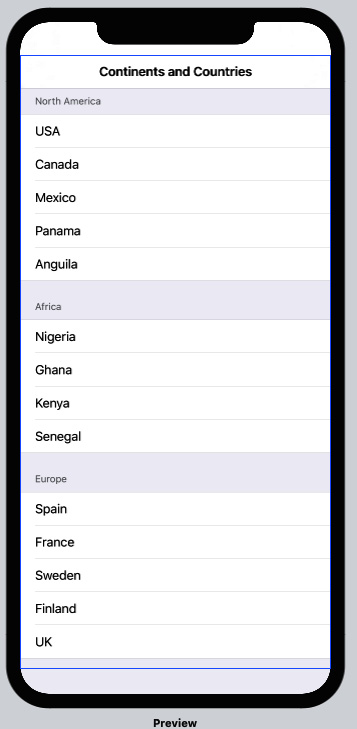
Figure 2.9 – ListWithSections preview
Good work! Now, run the app live preview and admire the work of your own hands.
How it works…
SwiftUI's sections are used to separate items into groups. In this recipe, we used sections to visually group countries into different continents. Sections can be used with a header, as follows:
Section(header: Text("Europe")){ Text("Spain") Text("France") Text("Sweden") Text("Finland") Text("UK") }
They can also be used without a header:
Section { Text("USA") Text("Canada") Text("Mexico") Text("Panama") Text("Anguilla") }
When using a section embedded in a list, we can add a .listStyle()
modifier to change the styling of the whole list.