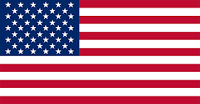

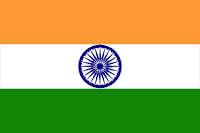
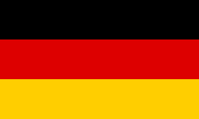
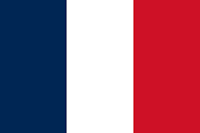

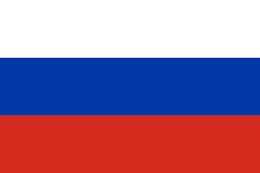
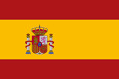



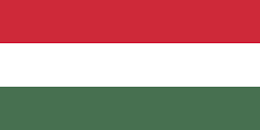
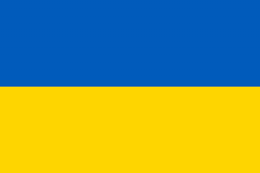
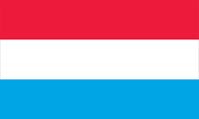

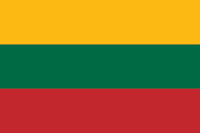

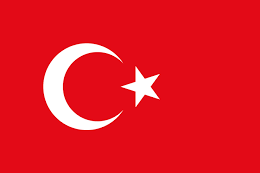


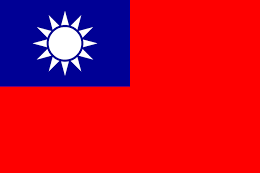
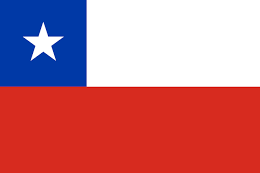

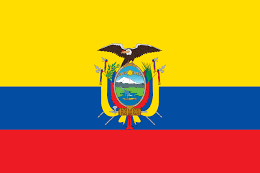
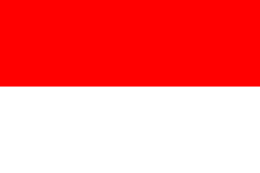
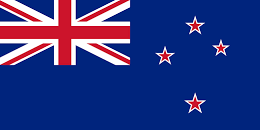
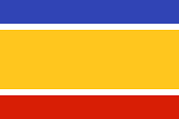
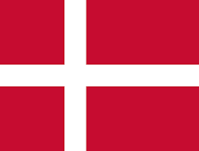
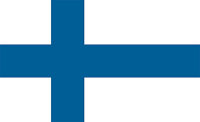


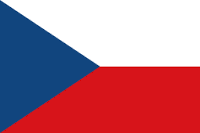
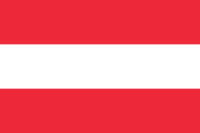
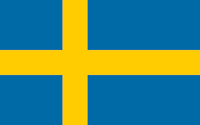
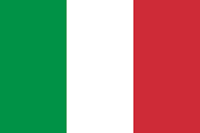
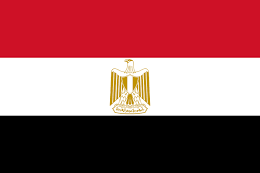

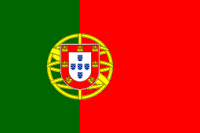
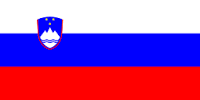

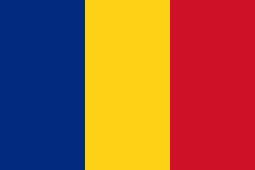
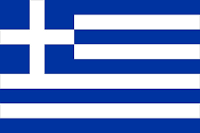


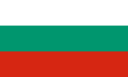
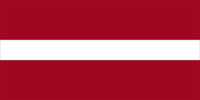
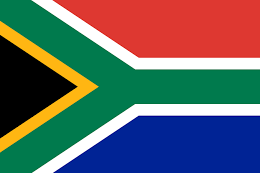
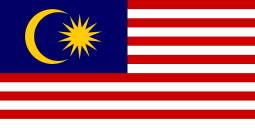



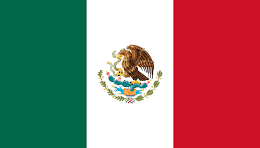
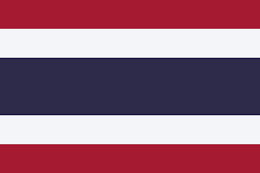
[box type="note" align="" class="" width=""]This is an excerpt from a book written by Alberto Paro, titled Elasticsearch 5.x Cookbook. This book is your one-stop guide to mastering the complete ElasticSearch ecosystem with comprehensive recipes on what’s new in Elasticsearch 5.x.[/box]
In this article we see how to create a standard Java HTTP Client in ElasticSearch. All the codes used in this article are available on GitHub. There are scripts to initialize all the required data.
An HTTP client is one of the easiest clients to create. It's very handy because it allows for the calling, not only of the internal methods as the native protocol does, but also of third- party calls implemented in plugins that can be only called via HTTP.
You need an up-and-running Elasticsearch installation. You will also need a Maven tool, or an IDE that natively supports it for Java programming such as Eclipse or IntelliJ IDEA, must be installed. The code for this recipe is in the chapter_14/http_java_client directory
.
For creating a HTTP client, we will perform the following steps:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.2</version>
</dependency>
import org.apache.http.*;
Import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils; import java.io.*;
public class App {
private static String wsUrl = "http://127.0.0.1:9200"; public static void main(String[] args) {
CloseableHttpClient client = HttpClients.custom()
.setRetryHandler(new MyRequestRetryHandler()).build();
HttpGet method = new HttpGet(wsUrl+"/test-index/test- type/1");
// Execute the method.
try {
CloseableHttpResponse response = client.execute(method);
if (response.getStatusLine().getStatusCode() != HttpStatus.SC_OK) {
System.err.println("Method failed: " +
response.getStatusLine());
}else{
HttpEntity entity = response.getEntity();
String responseBody = EntityUtils.toString(entity); System.out.println(responseBody);
}
} catch (IOException e) { System.err.println("Fatal transport error: " + e.getMessage());
e.printStackTrace();
} finally {
// Release the connection. method.releaseConnection();
}
}
}
{"_index":"test-index","_type":"test- type","_id":"1","_version":1,"exists":true, "_source" : {...}}
We perform the previous steps to create and use an HTTP client:
CloseableHttpClient client = HttpClients.custom().setRetryHandler(new MyRequestRetryHandler()).build();
HttpRequestRetryHandler
, which monitors the execution and repeats it three times before raising an error.HttpGet
and the URL will be item index/type/id. To initialize the method, the code is: HttpGet method = new HttpGet(wsUrl+"/test-index/test-type/1
basicAuth
, but works very well for non-complex deployments:HttpHost targetHost = new HttpHost("localhost", 9200, "http"); CredentialsProvider credsProvider = new BasicCredentialsProvider();
credsProvider.setCredentials(
new AuthScope(targetHost.getHostName(), targetHost.getPort()), new UsernamePasswordCredentials("username", "password"));
// Create AuthCache instance
AuthCache authCache = new BasicAuthCache();
// Generate BASIC scheme object and add it to local auth cache BasicScheme basicAuth = new BasicScheme(); authCache.put(targetHost, basicAuth);
// Add AuthCache to the execution context HttpClientContext context = HttpClientContext.create(); context.setCredentialsProvider(credsProvider);
response = client.execute(method, context);
request.addHeader("Accept-Encoding", "gzip");
response = client.execute(method, context);
if (response.getStatusLine().getStatusCode() != HttpStatus.SC_OK)
HttpEntity entity = response.getEntity();
String responseBody = EntityUtils.toString(entity);
The response is wrapped in HttpEntity
, which is a stream.
The HTTP client library provides a helper method EntityUtils.toString
that reads all the content of HttpEntity
as a string. Otherwise we'd need to create some code to read from the string and build the string. Obviously, all the read parts of the call are wrapped in a try-catch
block to collect all possible errors due to networking errors.
We saw a simple recipe to create a standard Java HTTP client in Elasticsearch.
If you enjoyed this excerpt, check out the book Elasticsearch 5.x Cookbook to learn how to create an HTTP Elasticsearch client, a native client and perform other operations in ElasticSearch.