Array Math
You can perform array math very easily on NumPy arrays. Consider the following two rank 2 arrays:
x1 = np.array([[1,2,3],[4,5,6]])
y1 = np.array([[7,8,9],[2,3,4]])
To add these two arrays together, you use the +
operator as follows:
print(x1 + y1)
The result is the addition of each individual element in the two arrays:
[[ 8 10 12]
[ 6 8 10]]
Array math is important, as it can be used to perform vector calculations. A good example is as follows:
x = np.array([2,3])
y = np.array([4,2])
z = x + y
'''
[6 5]
'''
Figure 2.5 shows the use of arrays to represent vectors and uses array addition to perform vector addition.
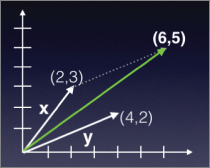
Figure 2.5: Using array addition for vector addition
Besides using the +
operator, you can also use the np.add()
function to add two arrays:
np.add(x1,y1)
Apart from addition, you can also perform subtraction, multiplication, as well as division with NumPy arrays:
print(x1 - y1) # same as np.subtract(x1,y1)
''&apos...