150. Allocating arrays into memory segments
Now that we know how to create memory segments for storing single values, let’s take it a step further and try to store an array of integers. For instance, let’s define a memory segment for storing the following array: [11, 21, 12, 7, 33, 1, 3, 6].
A Java int
needs 4 bytes (32 bits) and we have 8 integers, so we need a memory segment of 4 bytes x 8 = 32 bytes = 256 bits. If we try to represent this memory segment, then we can do it as in the following figure:
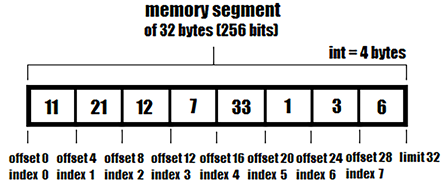
Figure 7.10: A memory segment of 8 integers
In code lines, we can allocate this memory segment via any of the following approaches (arena
is an instance of Arena
):
MemorySegment segment = arena.allocate(32);
MemorySegment segment = arena.allocate(4 * 8);
MemorySegment segment = arena.allocate(
ValueLayout.JAVA_INT.byteSize() * 8);
MemorySegment segment = arena.allocate(Integer.SIZE/8 * 8);
MemorySegment segment = arena.allocate(Integer.BYTES * 8...