Adding flexibility to the rendering pipeline using dynamic rendering
In this recipe, we will delve into the practical application of dynamic rendering in Vulkan to enhance the flexibility of the rendering pipeline. We will guide you through the process of creating pipelines without the need for render passes and framebuffers and discuss how to ensure synchronization. By the end of this section, you will have learned how to implement this feature in your projects, thereby simplifying your rendering process by eliminating the need for render passes and framebuffers and giving you more direct control over synchronization.
Getting ready
To enable the feature, we must have access to the VK_KHR_get_physical_device_properties2
instance extension, instantiate a structure of type VkPhysicalDeviceDynamicRenderingFeatures
, and set its dynamicRendering
member to true
:
const VkPhysicalDeviceDynamicRenderingFeatures dynamicRenderingFeatures = { .sType = VK_STRUCTURE_TYPE_PHYSICAL_DEVICE_DYNAMIC_RENDERING_FEATURES, .dynamicRendering = VK_TRUE, };
This structure needs to be plugged into the VkDeviceCreateInfo::pNext
member when creating a Vulkan device:
const VkDeviceCreateInfo dci = { .sType = VK_STRUCTURE_TYPE_DEVICE_CREATE_INFO, .pNext = &dynamicRenderingFeatures, ... };
Having grasped the concept of enabling dynamic rendering, we will now move forward and explore its implementation using the Vulkan API.
How to do it…
Instead of creating render passes and framebuffers, we must call the vkCmdBeginRendering
command and provide the attachments and their load and store operations using the VkRenderingInfo
structure. Each attachment (colors, depth, and stencil) must be specified with instances of the VkRenderingAttachmentInfo
structure. Figure 2.13 presents a diagram of the structure participating in a call to vkCmdBeginRendering
:
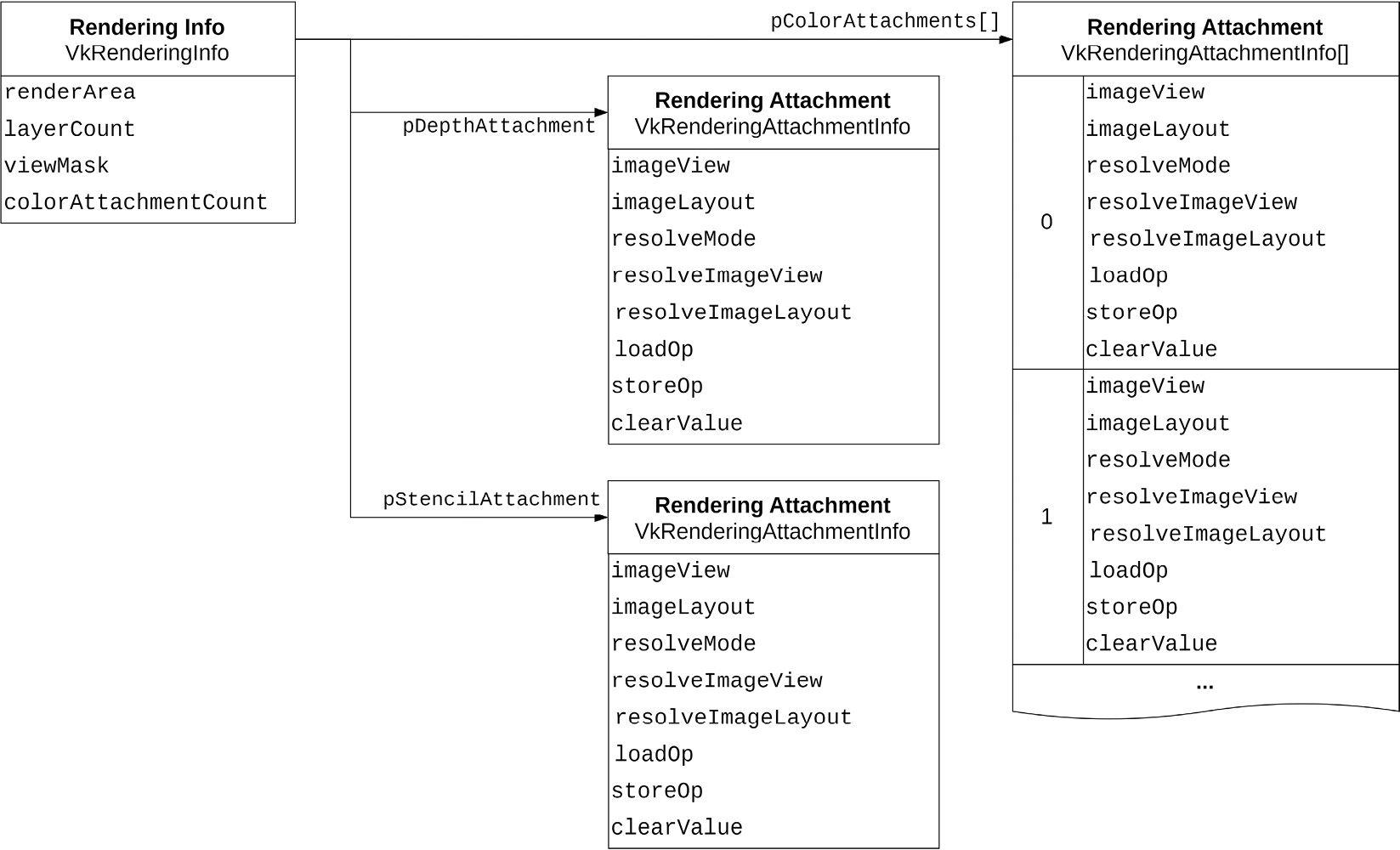
Figure 2.13 – Dynamic rendering structure diagram
Any one of the attachments, pColorAttachments
, pDepthAttachment
, and pStencilAttachment
, can be null
. Shader output written to location x
is written to the color attachment at pColorAttachment[x]
.