Creating cutscenes with Timeline
We have our intro camera, but that’s not enough to create a cutscene. A proper cutscene is a sequence of actions happening at the exact moment that they should happen, coordinating several objects to act as intended. We can have actions such as enabling and disabling objects, switching cameras, playing sounds, moving objects, and so on. To do this, Unity offers Timeline, which is a sequencer of actions to coordinate those kinds of cutscenes. We will use Timeline to create an intro cutscene for our scene, showing the level before starting the game.
In this section, we will examine the following Timeline concepts:
- Creating animation clips
- Sequencing our intro cutscene
We are going to see how to create our own animation clips in Unity to animate our GameObjects and then place them inside a cutscene to coordinate their activation using the Timeline sequencer tool. Let’s start by creating a camera animation to use later in Timeline.
Creating animation clips
This is actually not a Timeline-specific feature but rather a Unity feature that works great with Timeline. When we downloaded the character, it came with animation clips that were created using external software, but you can create custom animation clips using Unity’s Animation window. Don’t confuse it with the Animator window, which allows us to create animation transitions that react to the game situation. This is useful to create small object-specific animations that you will coordinate later in Timeline with other objects’ animations.
These animations can control any value of an object’s component properties, such as the positions, colors, and so on. In our case, we want to animate the dolly track’s Position property to make it go from start to finish in a given time. In order to do this, do the following:
- Select the
DollyCart1
object. - Open the Animation (not Animator) window by going to Window | Animation | Animation.
- Click on the Create button at the center of the Animation window. Remember to do this while the dolly cart (not track) is selected:
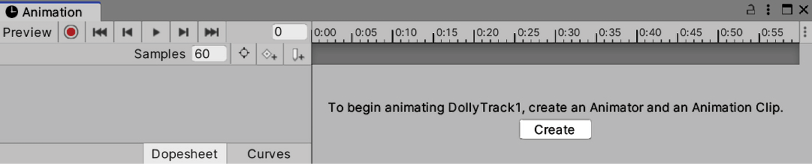
Figure 17.61: Creating a custom animation clip
- After doing this, you will be prompted to save the animation clip somewhere. I recommend you create an
Animations
folder in the project (inside theAssets
folder) and call itIntroDollyTrack
.
If you pay attention, the dolly cart now has an Animator component with an Animator Controller created, which contains the animation we just created. As with any animation clip, you need to apply it to your object with an Animator Controller; custom animations are no exception. So, the Animation window created them for you.
Animating in this window consists of specifying the value of its properties at given moments. In our case, we want Position to have a value of 0
at the beginning of the animation, at 0 seconds on the timeline, and have a value of 254
at the end of the animation, at 5 seconds. I chose 254
because that’s the last possible position in my cart, but that depends on the length of your dolly track. Just test which is the last possible position in yours. Also, I chose 5
seconds because that’s what I feel is the correct length for the animation, but feel free to change it as you wish. Now, whatever happens between the animation’s 0 and 5 seconds is an interpolation of the 0
and 254
values, meaning that in 2.5 seconds, the value of Position
will be 127
. Animating always consists of interpolating different states of our object at different moments.
In order to do this, follow these steps:
- In the Animation window, click on the record button (the red circle in the top-left section). This will make Unity detect any changes in our object and save them to the animation. Remember to do this while you have selected the dolly cart.
- Set the Position setting of the dolly cart to
1
and then0
. Changing this to any value and then to0
again will create a keyframe, which is a point in the animation that says that at0
seconds, we want the Position value to be0
. We need to set it first to any other value if the value is already at0
.You will notice that the Position property has been added to the animation:
Figure 17.62: The animation in Record mode after changing the Position value to 0
- Using the mouse scroll wheel, zoom out the timeline to the right of the Animation window until you see 5:00 seconds in the top bar:
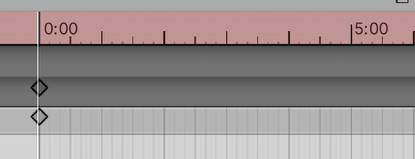
Figure 17.63: The timeline of the Animation window seeing 5 seconds
- Click on the 5:00-second label in the top bar of the timeline to position the playback header at that moment. This will locate the next change we make at that moment.
- Set the Position value of the dolly track to the highest value you can get; in my case, this is
240
. Remember to have the Animation window in Record mode:
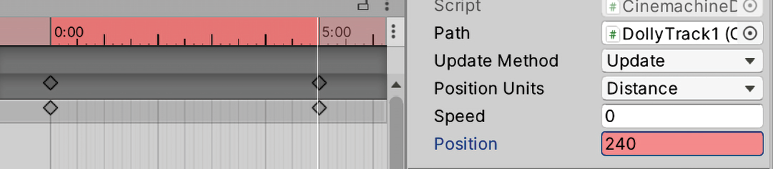
Figure 17.64: Creating a keyframe with the 240 value 5 seconds into the animation
- Hit the play button in the top-left section of the Animation window to see the animation playing. Remember to view it in the Game view while
CM vcam1
is disabled.
Sometimes, you will need to synchronize gameplay code with animations. One classic example is an attack animation, where you want the player to damage the attacked object when the sword hits the ground instead of as soon as the animation starts. To do this, you can use animation events: https://docs.unity3d.com/Manual/script-AnimationWindowEvent.html. You can also add animation events to imported animations: https://docs.unity3d.com/Manual/class-AnimationClip.html.
Now, if we hit Play, the animation will start playing, but that’s something we don’t want. In this scenario, the idea is to give control of the cutscene to the cutscene system, Timeline, because this animation won’t be the only thing that needs to be sequenced in our cutscene. One way to prevent the Animator component from automatically playing the animation we created is to create an empty animation state in the Controller and set it as the default state by following these steps:
- Search the Animator Controller that we created at the same time as the animation and open it. If you can’t find it, just select the dolly cart and double-click on the Controller property of the Animator component on our GameObject to open the asset.
- Right-click on an empty state in the Controller and select Create State | Empty. This will create a new state in the state machine as if we created a new animation, but it is empty this time:
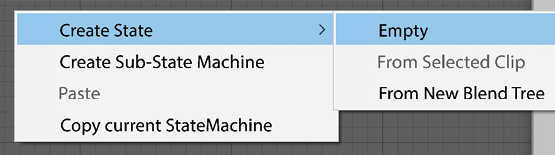
Figure 17.65: Creating an empty state in the Animator Controller
- Right-click on New State and click on Set as Layer Default State. The state should become orange:
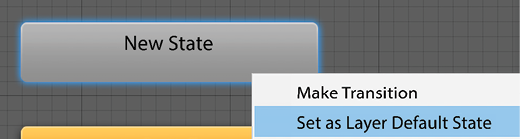
Figure 17.66: Changing the default animation of the Controller to an empty state
- Now, if you hit Play, no animation will play as the default state of our dolly cart is empty. No transition will be required in this case.
Now that we have created our camera animation, let’s start creating a cutscene that switches from the intro cutscene camera to the player camera by using Timeline.
Sequencing our intro cutscene
Timeline is already installed in your project, but if you go to the Package Manager of Timeline, you may see an Update button to get the latest version if you need some of the new features. In our case, we will keep the default version included in our project (1.5.2, at the time of writing this book).
The first thing we will do is create a cutscene asset and an object in the scene responsible for playing it. To do this, follow these steps:
- Create an empty GameObject using the GameObject | Create Empty option.
- Select the empty object and call it
Director
. - Go to Window | Sequencing | Timeline to open the Timeline editor.
- Click the Create button in the middle of the Timeline window while the Director object is selected to convert that object into the cutscene player (or director).
- After doing this, a window will pop up asking you to save a file. This file will be the cutscene or timeline; each cutscene will be saved in its own file. Save it in a
Cutscenes
folder in your project (theAssets
folder). - Now, you can see that the Director object has a Playable Director component with the Intro cutscene asset saved in the previous step set for the Playable property, meaning this cutscene will be played by the Director:
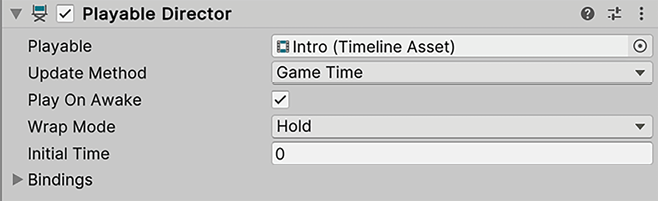
Figure 17.67: Playable Director prepared to play the Intro Timeline asset
Now that we have the Timeline asset ready to work with, let’s make it sequence actions. To start, we need to sequence two things—first, the cart position animation we did in the last step and then the camera swap between the dolly track camera (CM vcam2
) and the player cameras (CM vcam1
). As we said before, a cutscene is a sequence of actions executing at given moments, and in order to schedule actions, you will need tracks. In Timeline, we have different kinds of tracks, each one allowing you to execute certain actions on certain objects. We will start with the animation track.
The animation track will control which animation a specific object will play; we need one track per object to animate. In our case, we want the dolly track to play the Intro animation that we created, so let’s do that by following these steps:
- Add an Animation track by clicking the plus button (+) and then Animation Track:
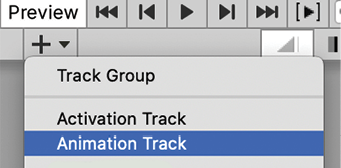
Figure 17.68: Creating an animation track
- Select the Director object and check the Bindings list of the Playable Director component in the Inspector window.
- Drag the Cart object to specify that we want the animation track to control its animation:
Figure 17.69: Making the animation track control the dolly cart animation in this Director
Timeline is a generic asset that can be applied to any scene, but as the tracks control specific objects, you need to manually bind them in every scene. In our case, we have an animation track that expects to control a single animator, so in every scene, if we want to apply this cutscene, we need to drag the specific animator to control it in the Bindings list.
- Drag the Intro animation asset that we created to the animation track in the Timeline window. This will create a clip in the track showing when and for how long the animation will play. You can drag as many animations as possible that the cart can play into the track to sequence different animations at different moments, but right now, we want just that one:

Figure 17.70: Making the animator track play the intro clip
- You can drag the animation to change the exact moment you want it to play. Drag it to the beginning of the track.
- Hit the Play button in the top-left part of the Timeline window to see it in action. You can also manually drag the white arrow in the Timeline window to view the cutscene at different moments. If that doesn’t work, try playing the game and then stopping:
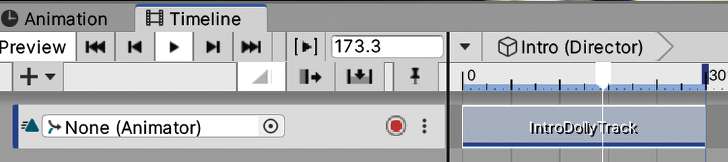
Figure 17.71: Playing a timeline and dragging the playback header
Now, we will make our Intro timeline asset tell the CinemachineBrain
component (the main camera) which camera will be active during each part of the cutscene, switching to the player camera once the camera animation is over. We will create a second track—a Cinemachine track—which specializes in making a specific CinemachineBrain
component to switch between different virtual cameras. To do this, follow these steps:
- Click the + button again and click on Cinemachine Track. Note that you can install Timeline without Cinemachine, but this kind of track won’t appear in that case:
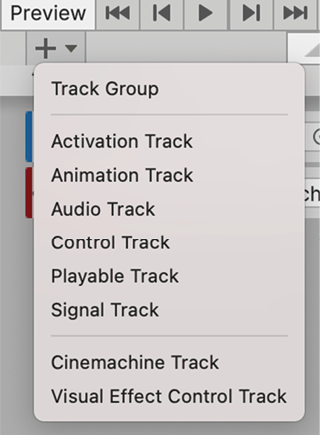
Figure 17.72: Creating a new Cinemachine track
- In the Playable Director component’s Bindings list, drag the main camera to Cinemachine Track to make it track control which virtual camera will control the main camera at different moments of the cutscene:
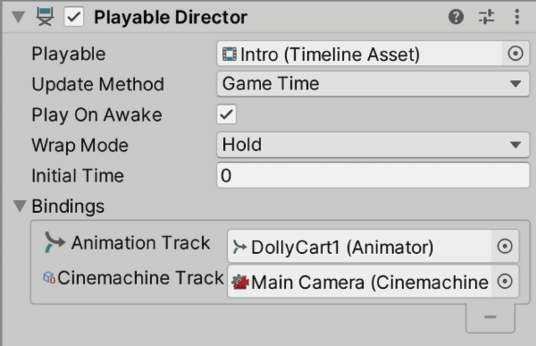
Figure 17.73: Binding the main camera to the Cinemachine track
- The next step indicates which virtual camera will be active during specific moments of the timeline. To do so, our Cinemachine track allows us to drag virtual cameras to it, which will create virtual camera clips. Drag both CM vcam2 and CM vcam1, in that order, to the Cinemachine track:

Figure 17.74: Dragging virtual cameras to the Cinemachine track
- If you hit the Play button or just drag the Timeline Playback header, you can see how the active virtual camera changes when the playback header reaches the second virtual camera clip. Remember to view this in the Game view.
- If you place the mouse near the ends of the clips, a resize cursor will appear. If you drag them, you can resize the clips to specify their duration. In our case, we will need to match the length of the
CM vcam2
clip to the Cart animation clip and then putCM vcam1
at the end of it by dragging it so that the camera will be active when the dolly cart animation ends. In my case, they were already the same length, but just try to change it anyway to practice. Also, you can make theCM vcam1
clip shorter; we just need to play it for a few moments to execute the camera swap. - You can also overlap the clips a little bit to make a smooth transition between the two cameras, instead of a hard switch, which will look odd:
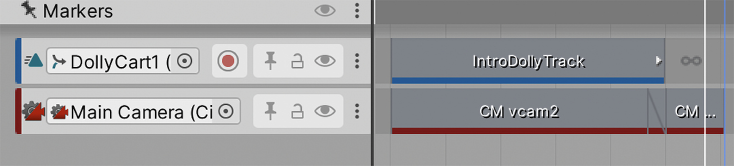
Figure 17.75: Resizing and overlapping clips to interpolate them
- Increase the Start Time property of the WaveSpawners to prevent the enemies from being spawned before the cutscene begins.
If you wait for the full cutscene to end, you will notice how, at the very end, CM vcam2
becomes active again. You can configure how Timeline will deal with the end of the cutscene, as, by default, it does nothing. This can cause different behavior according to the type of track—in our case, again giving control to pick the virtual camera to the CinemachineBrain
component, which will pick the virtual camera with the highest Priority value. We can change the Priority property of the virtual cameras to be sure that CM vcam1 (the player camera) is always the more important one or set the Wrap Mode of the Playable Director component to Hold, which will keep everything as the last frame of the timeline specifies. In our case, we will use the latter option to test the Timeline-specific features:
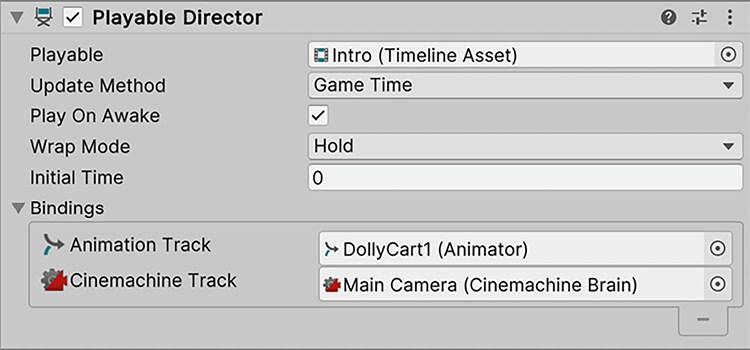
Figure 17.76: Wrap Mode set to Hold mode
Most of the different kinds of tracks work under the same logic; each one will control a specific aspect of a specific object using clips that will execute during a set time. I encourage you to test different tracks to see what they do, such as Activation, which enables and disables objects during the cutscene. Remember, you can check out the documentation of the Timeline package in the Package Manager.