In this section, you'll create the map where all the action will take place. As the name implies, you'll probably want to make a maze-like level with lots of twists and turns.
Here is a sample level:
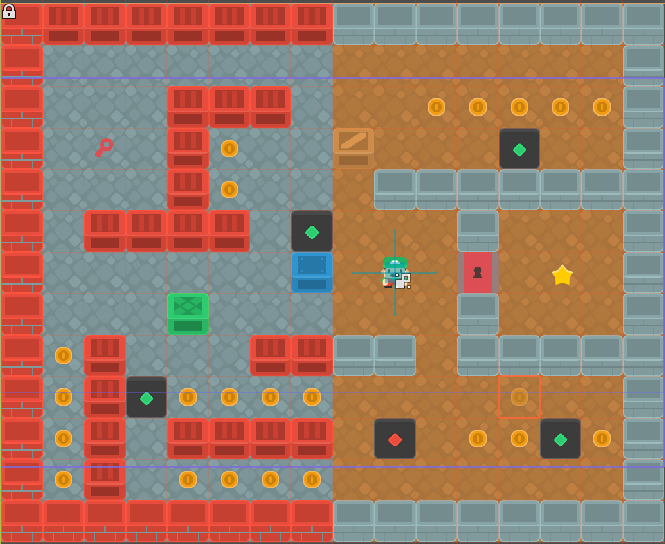
The player's goal is to reach the star. Locked doors can only be opened by picking up the key. The green dots mark the spawn locations of enemies, while the red dot marks the player's start location. The coins are extra items that can be picked up along the way for bonus points. Note that the entire level is larger than the display window. The Camera will scroll the map as the player moves around it.
You'll use the TileMap node to create the map. There are several benefits to using a TileMap for your level design. First, they make it possible to draw the level's layout by painting the tiles onto a grid, which is much faster than placing...