Sphere
A sphere is the 3D version of a circle. It is defined by a 3D point in space and a radius. Like a circle in 2D, in 3D the sphere is considered to be one of the simplest shapes we can implement. The simplicity of a sphere makes it very fast for collision detection:
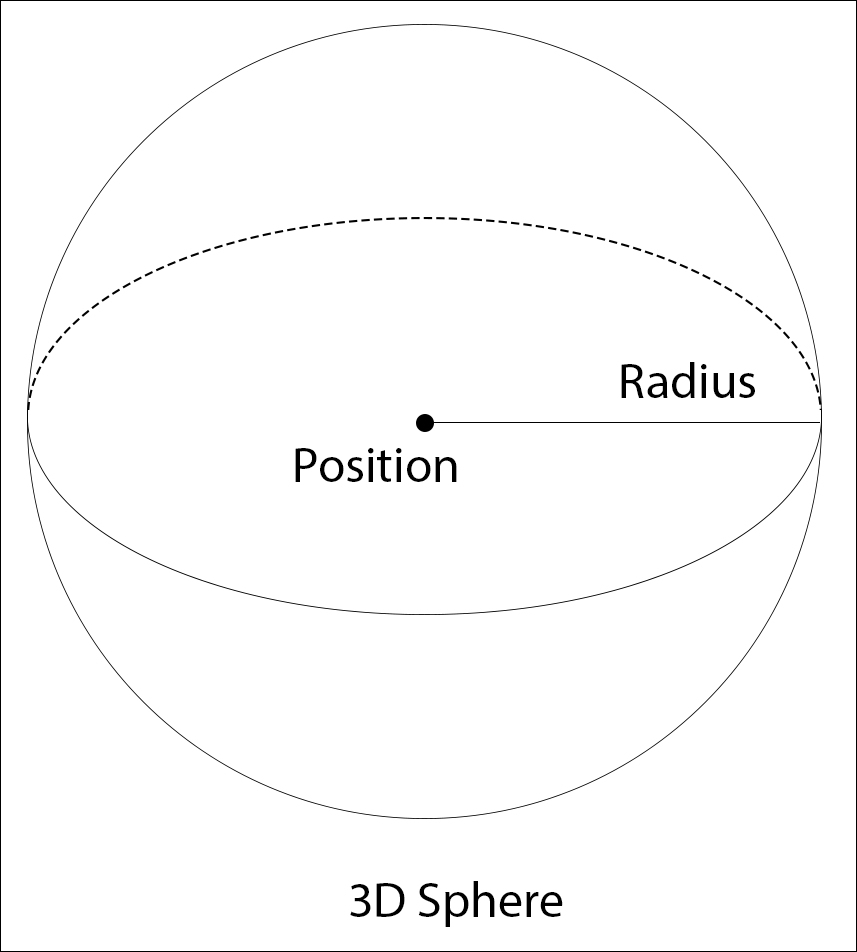
Getting ready
We are going to declare a new Sphere
structure in the Geometry3D.h
header file. This structure will hold a position and a radius.
How to do it…
Follow these steps to implement a 3D sphere:
Declare the
Sphere
structure inGeometry3D.h
:typedef struct Sphere {
Start by declaring the position and radius variables of the Sphere structure:
Point position; float radius;
Finish implementing the structure by adding a default constructor, and one which takes a point and radius to construct a sphere out of:
inline Sphere() : radius(1.0f) { } inline Sphere(const Point& p, float r) : position(p), radius(r) { } } Sphere;
How it works…
The Sphere
structure contains a position and a radius. It has two constructors...