Simple and complex shapes
Sometimes a containing circle or a containing rectangle alone is not accurate enough for collision detection. When this happens we can use several simple shapes to approximate a complex shape:
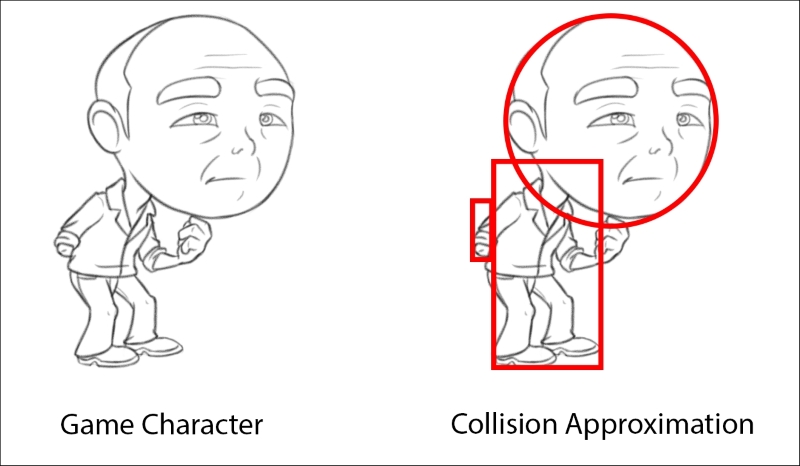
Getting ready
We are going to create a new structure called BoundingShape
. This new structure will hold an array of circles and an array of rectangles. It's assumed that the structure does not own the memory it is referencing. We can implement several primitive tests by looping through all the primitives that BoundingShape
contains.
How to do it…
Follow these steps to create a class which represents a complex shape. A complex shape is made out of many simple shapes:
Declare the
BoundingShape
primitive inGeometry2D.h
:typedef struct BoundingShape { int numCircles; Circle* circles; int numRectangles; Rectangle2D* rectangles; inline BoundingShape() : numCircles(0), circles(0), numRectangles(0), rectangles(0) { } };
Define a new
PointInShape
function inGeometry2D...