Writing conditional expressions
When writing the Terraform configuration, we may need to make the code more dynamic by integrating various conditions. In this recipe, we will discuss an example of a conditional expression.
Getting ready
For this recipe, we will use the Terraform configuration we wrote in the previous recipe, the code for which is available at https://github.com/PacktPublishing/Terraform-Cookbook-Second-Edition/tree/main/CHAP02/fct.
We will complete this code by adding a condition to the name of the resource group. This condition is as follows: if the name of the environment is equal to Production
, then the name of the resource group will be in the form RG-<APP NAME>
; otherwise, the name of the resource group will be in the form RG-<APP NAME>-<ENVIRONMENT NAME>
.
How to do it…
In the Terraform configuration of the main.tf
file, modify the code of the resource group as follows:
resource "azurerm_resource_group" "rg-app" {
name = var.environment == "Production" ? upper(format("RG-%s",var.app-name)) : upper(format("RG-%s-%s",var.app-name,var.environment))
location = "westeurope"
}
How it works…
Here, we added the following condition:
condition ? true assert : false assert
The result of executing Terraform commands on this code if the environment
variable is equal to Production
can be seen in the following screenshot:
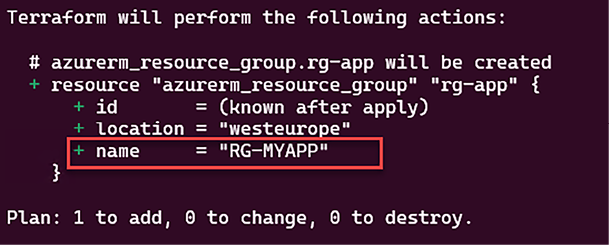
Figure 2.14: Conditional expression first use case
If the environment
variable is not equal to Development
, we’ll get the following output:
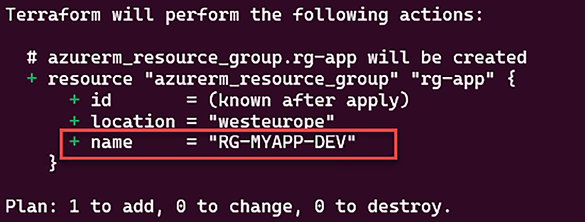
Figure 2.15: Conditional expression second use case
There’s more…
One of the usual use cases for conditional expressions is to implement a pattern of features
flags.
With features
flags, we can make the provisioning of resources optional in a dynamic way, as shown in the following code snippet:
resource "azurerm_application_insights" "appinsight-app" {
count = var.use_appinsight == true ? 1 : 0
....
}
In this code, we have indicated to Terraform that if the use_appinsight
variable is true
, then the count
property is 1
, which will allow us to provision one Azure Application Insights resource. Conversely, where the use_appinsight
variable is false
, the count
property is 0
and in this case, Terraform does not create an Application Insights resource instance.
See also
Documentation on conditional expressions in Terraform can be found at https://www.terraform.io/language/expressions/conditionals.