Chapter 6. Creating Animated Movies with Raspberry Pi
In the previous chapter, we learned how to use GPIO pins to control LEDs and get input from buttons. We also learned how to control the PiGlow module with its provided Python API. In Chapter 4, Working with Webcam and Pi Camera, we learned how to operate Pi Camera or a general webcam to capture images and videos. In this chapter, we will combine the two concepts and learn how to create stop-motion animation. In this chapter, we will learn about the following:
- Creating a stop-motion animation using a push button
- Examining the theory behind stop-motion
- Revisiting some GPIO concepts
- Using the
ffmpeg
library to render the shots into a video
Introducing stop-motion animation
A stop-motion animation is basically a video of sequenced images, such as those where an object is physically manipulated so that it appears to move on its own when the video is played. The position of the object is changed incrementally after each shot so that over a course of multiple frames, it appears as though the object is moving. A common subject for stop-motion animations is a clay object, such as a bird, animal, and so on.
Setting up the prerequisites
In addition to the Raspberry Pi, for this chapter we will require the following:
- Pi Camera
- Tactile push button
- Breadboard
- A few jumper cables
- The tactile button can be purchased from your local hardware shop or online from sellers such as Adafruit. It might look something like the following:
- The breadboard is basically a temporary connection mechanism for electronic components. Each pin in the 5-pin row is connected to each other, so they behave as a common terminal for each component connected to the same row. All the pins in the side rail are electrically connected and primarily serve as power lines. A breadboard looks like the following:
Jumper cables are nothing but wires that allow us to connect different rows in a breadboard and look like this:
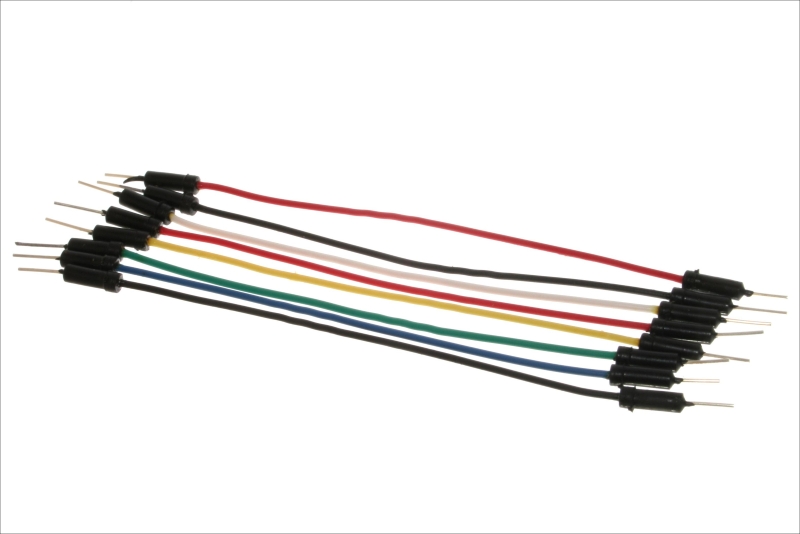
We will require the ffmpeg
library installed on our Pi. This can be installed with the following command:
sudo apt-get install ffmpeg
Once the installation is finished, you can test it by executing the following command:
avconv
If it is installed properly, then you should see some information about the avconv
tool. If not, then try to install it properly and then run the command again.
Setting up and testing the camera
Before booting up the Pi, we need to connect Pi Camera to it. This is as simple as locating the camera port beside the Ethernet port, inserting the camera strip in it, and pushing the tab down to secure it.
You can now test to check whether this works. After booting up the Pi, issue the following command:
raspistill -o image.jpg
If everything went fine, you should now see an image in your home directory. Open it to check whether the camera took a satisfactory picture. Don't worry if it is upside down as we will correct this shortly. It will look something like this:
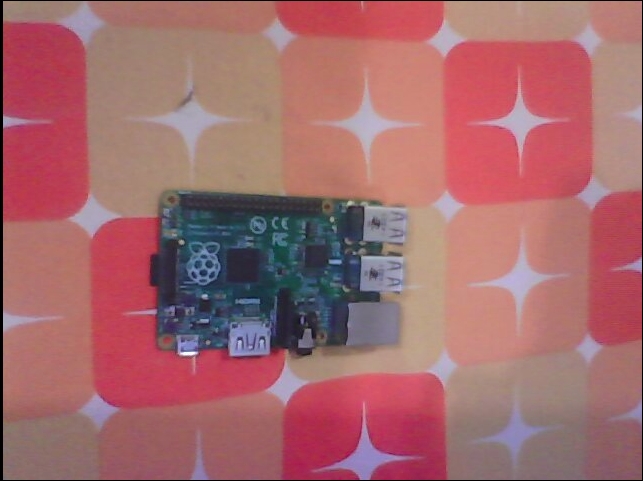
Now, we will look at the code to take a picture with Python. As we did earlier, we will first see the code completely and then learn what it does statement by statement. Here it is:
import picamera from time import sleep with picamera.PiCamera() as camera: camera.start_preview() sleep(3) camera.capture('/home/pi/image.jpg') camera.stop_preview()
We first import the PI camera library, which is required to take pictures with PiCam. We also import the time library to provide delays in taking a picture. The next statement essentially ensures that we can access picamera.PiCamera()
with the same camera. The camera.start_preview()
method starts a preview screen for the camera, and after a delay of 3 seconds the capture()
method takes a picture. The argument to the capture command gives the filename for the image file. Finally, the stop_preview()
method stops the preview.
Save the file as prog1.py
and run it with the following command as this is a python3
program:
python3 prog1.py
Now, double-click on the new image file from the File Explorer and check whether it is upside down. If it is, then add the following two lines before the start_preview()
method to correct it:
camera.vflip = True camera.hflip = True
Adding the hardware button
To our current setup, we will add a hardware input button so that we can take pictures when we press that button. So, connect a tactile button to the Raspberry Pi, as shown in the following image:
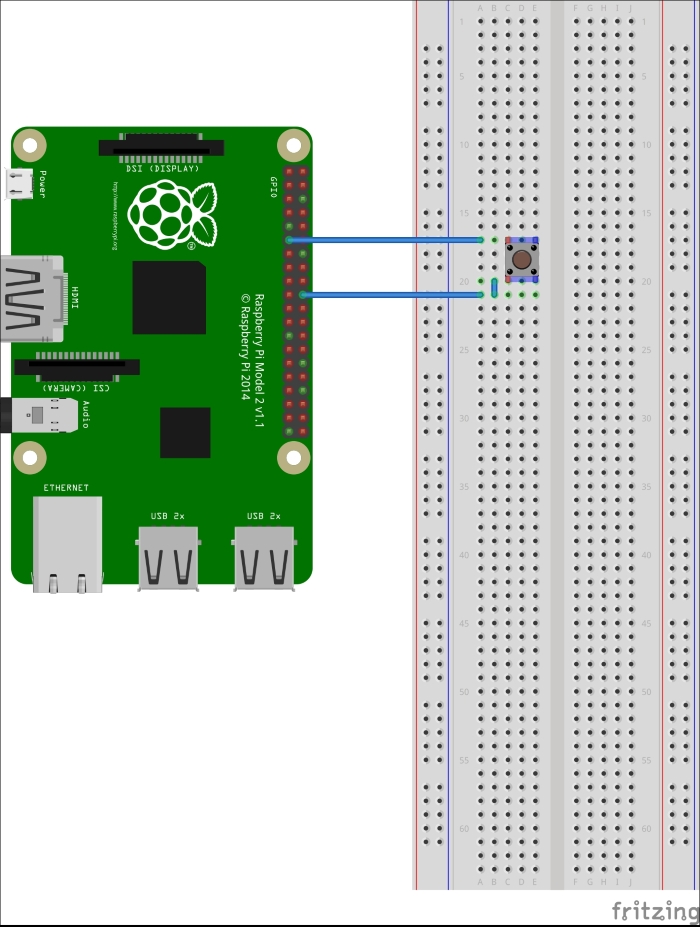
This time, we will use the BCM convention when specifying GPIO pins. We will connect the button to the GPIO17 pin. Then, we will modify the code so that it looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/image.jpg') camera.stop_preview()
Save the preceding program as prog2.py
and run it with the following command:
python3 prog2.py
We will now see what each line of code does, except those that we already saw in the previous program. To use the GPIO pins, we have to import the RPi.GPIO
library, as we did in the previous chapter. Then, we set the GPIO addressing mode as BCM, which uses the Broadcom convention rather than numbering the pins on the board. This means that we can address the GPIO pin by its GPIO number rather than its board number. With the setup()
method, we configure the pin to be an input and set it so that it is pulled up by default.
The function that actually does the magic is wait_for_edge()
. It takes the pin number as the first argument and GPIO_FALLING
as the second. This means that the function will be triggered only when the signal at pin 17 goes from high to low or when it falls. So, it will be triggered when the button goes from the pressed state to the unpressed state; that is, it will be triggered when we leave the button rather than when we press the button. The next statements capture and save the image and then exit the preview. If we want to take a selfie, then we can add a delay after the wait_for_edge()
function in order to enable us to get into position. Also, don't forget to add the two commands to correct an upside down picture if such a need arises.
Now that we have successfully taken individual photos without a camera, it's time to try taking a series of still images and combining them in order to make a stop-motion animation. Just so that your home folder does not just get messy, we can create a new folder and enter it with the following commands:
mkdir stopmotion cd stopmotion
Modify the given code and add a loop to take a picture every time the button is pressed. The new code looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() frame = 1 while True: GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/stopmotion/frame%03d.jpg' % frame) frame += 1 camera.stop_preview()
What we have done is create an infinite while loop that keeps taking photographs as long as the button is pressed. The frame variable keeps track of the current frame and saves the corresponding image in the folder.
Note
But be careful about the space limitations of your SD card. If we hold down the button for long, the Pi could run out of space and crash once it run out of space, causing us to lose all the data and potentially corrupting the SD card.
Now, save the program as prog3.py
and run it with the following command:
python3 prog3.py
Run the program and collect as many images as required for our stop-motion project. Press Ctrl + C to exit the program and open the File Manager to see your images.
Setting up and testing the camera
Before booting up the Pi, we need to connect Pi Camera to it. This is as simple as locating the camera port beside the Ethernet port, inserting the camera strip in it, and pushing the tab down to secure it.
You can now test to check whether this works. After booting up the Pi, issue the following command:
raspistill -o image.jpg
If everything went fine, you should now see an image in your home directory. Open it to check whether the camera took a satisfactory picture. Don't worry if it is upside down as we will correct this shortly. It will look something like this:
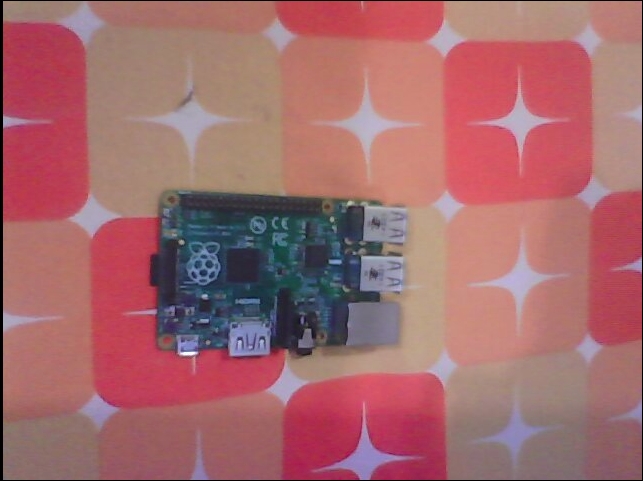
Now, we will look at the code to take a picture with Python. As we did earlier, we will first see the code completely and then learn what it does statement by statement. Here it is:
import picamera from time import sleep with picamera.PiCamera() as camera: camera.start_preview() sleep(3) camera.capture('/home/pi/image.jpg') camera.stop_preview()
We first import the PI camera library, which is required to take pictures with PiCam. We also import the time library to provide delays in taking a picture. The next statement essentially ensures that we can access picamera.PiCamera()
with the same camera. The camera.start_preview()
method starts a preview screen for the camera, and after a delay of 3 seconds the capture()
method takes a picture. The argument to the capture command gives the filename for the image file. Finally, the stop_preview()
method stops the preview.
Save the file as prog1.py
and run it with the following command as this is a python3
program:
python3 prog1.py
Now, double-click on the new image file from the File Explorer and check whether it is upside down. If it is, then add the following two lines before the start_preview()
method to correct it:
camera.vflip = True camera.hflip = True
Adding the hardware button
To our current setup, we will add a hardware input button so that we can take pictures when we press that button. So, connect a tactile button to the Raspberry Pi, as shown in the following image:
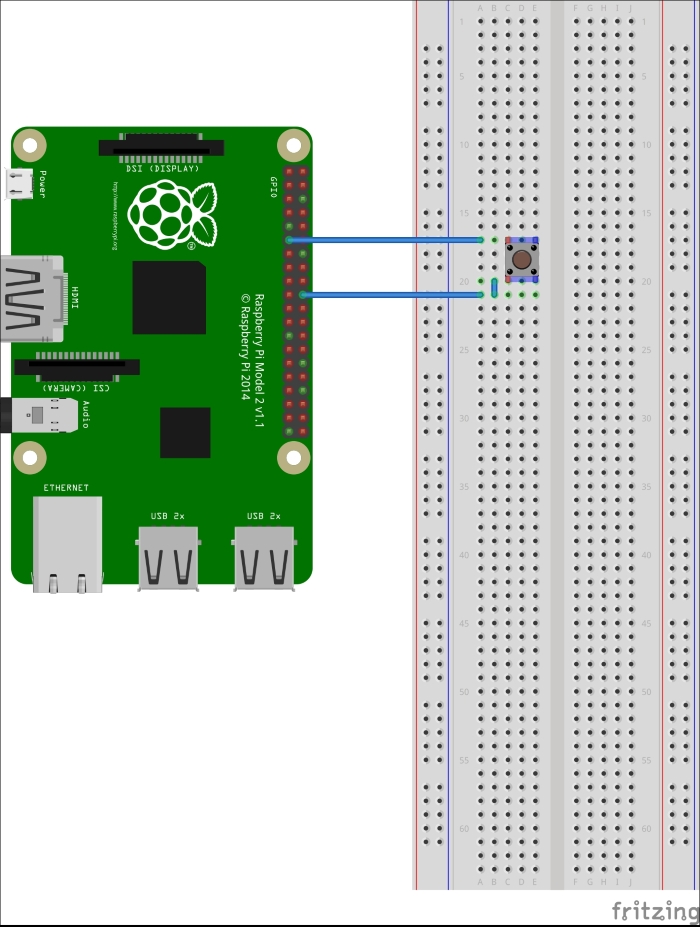
This time, we will use the BCM convention when specifying GPIO pins. We will connect the button to the GPIO17 pin. Then, we will modify the code so that it looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/image.jpg') camera.stop_preview()
Save the preceding program as prog2.py
and run it with the following command:
python3 prog2.py
We will now see what each line of code does, except those that we already saw in the previous program. To use the GPIO pins, we have to import the RPi.GPIO
library, as we did in the previous chapter. Then, we set the GPIO addressing mode as BCM, which uses the Broadcom convention rather than numbering the pins on the board. This means that we can address the GPIO pin by its GPIO number rather than its board number. With the setup()
method, we configure the pin to be an input and set it so that it is pulled up by default.
The function that actually does the magic is wait_for_edge()
. It takes the pin number as the first argument and GPIO_FALLING
as the second. This means that the function will be triggered only when the signal at pin 17 goes from high to low or when it falls. So, it will be triggered when the button goes from the pressed state to the unpressed state; that is, it will be triggered when we leave the button rather than when we press the button. The next statements capture and save the image and then exit the preview. If we want to take a selfie, then we can add a delay after the wait_for_edge()
function in order to enable us to get into position. Also, don't forget to add the two commands to correct an upside down picture if such a need arises.
Now that we have successfully taken individual photos without a camera, it's time to try taking a series of still images and combining them in order to make a stop-motion animation. Just so that your home folder does not just get messy, we can create a new folder and enter it with the following commands:
mkdir stopmotion cd stopmotion
Modify the given code and add a loop to take a picture every time the button is pressed. The new code looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() frame = 1 while True: GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/stopmotion/frame%03d.jpg' % frame) frame += 1 camera.stop_preview()
What we have done is create an infinite while loop that keeps taking photographs as long as the button is pressed. The frame variable keeps track of the current frame and saves the corresponding image in the folder.
Note
But be careful about the space limitations of your SD card. If we hold down the button for long, the Pi could run out of space and crash once it run out of space, causing us to lose all the data and potentially corrupting the SD card.
Now, save the program as prog3.py
and run it with the following command:
python3 prog3.py
Run the program and collect as many images as required for our stop-motion project. Press Ctrl + C to exit the program and open the File Manager to see your images.
Adding the hardware button
To our current setup, we will add a hardware input button so that we can take pictures when we press that button. So, connect a tactile button to the Raspberry Pi, as shown in the following image:
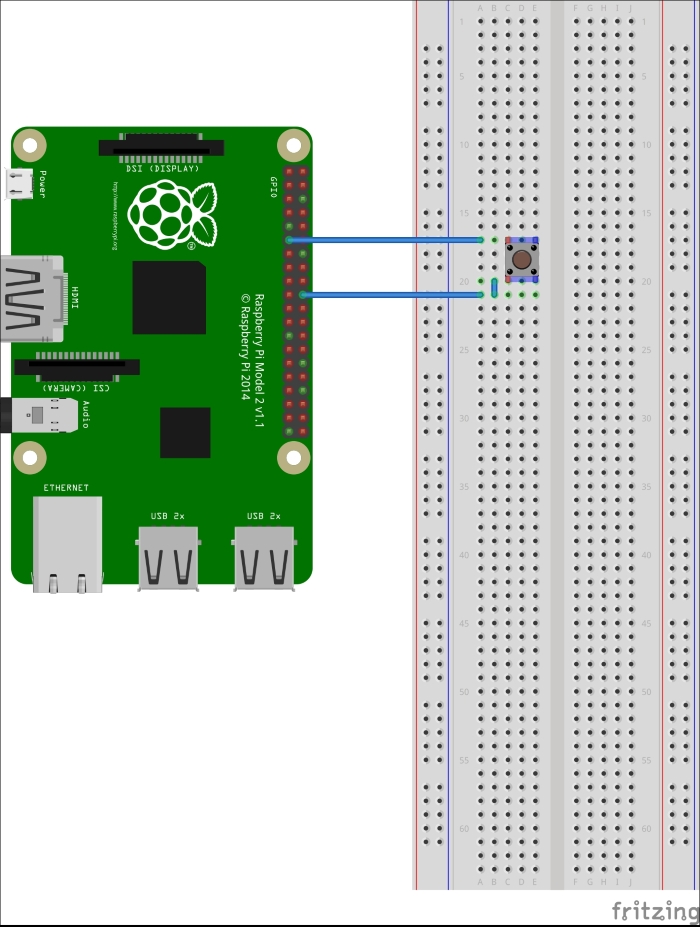
This time, we will use the BCM convention when specifying GPIO pins. We will connect the button to the GPIO17 pin. Then, we will modify the code so that it looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/image.jpg') camera.stop_preview()
Save the preceding program as prog2.py
and run it with the following command:
python3 prog2.py
We will now see what each line of code does, except those that we already saw in the previous program. To use the GPIO pins, we have to import the RPi.GPIO
library, as we did in the previous chapter. Then, we set the GPIO addressing mode as BCM, which uses the Broadcom convention rather than numbering the pins on the board. This means that we can address the GPIO pin by its GPIO number rather than its board number. With the setup()
method, we configure the pin to be an input and set it so that it is pulled up by default.
The function that actually does the magic is wait_for_edge()
. It takes the pin number as the first argument and GPIO_FALLING
as the second. This means that the function will be triggered only when the signal at pin 17 goes from high to low or when it falls. So, it will be triggered when the button goes from the pressed state to the unpressed state; that is, it will be triggered when we leave the button rather than when we press the button. The next statements capture and save the image and then exit the preview. If we want to take a selfie, then we can add a delay after the wait_for_edge()
function in order to enable us to get into position. Also, don't forget to add the two commands to correct an upside down picture if such a need arises.
Now that we have successfully taken individual photos without a camera, it's time to try taking a series of still images and combining them in order to make a stop-motion animation. Just so that your home folder does not just get messy, we can create a new folder and enter it with the following commands:
mkdir stopmotion cd stopmotion
Modify the given code and add a loop to take a picture every time the button is pressed. The new code looks like the following:
import picamera from RPi import GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP) with picamera.PiCamera() as camera: camera.start_preview() frame = 1 while True: GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/stopmotion/frame%03d.jpg' % frame) frame += 1 camera.stop_preview()
What we have done is create an infinite while loop that keeps taking photographs as long as the button is pressed. The frame variable keeps track of the current frame and saves the corresponding image in the folder.
Note
But be careful about the space limitations of your SD card. If we hold down the button for long, the Pi could run out of space and crash once it run out of space, causing us to lose all the data and potentially corrupting the SD card.
Now, save the program as prog3.py
and run it with the following command:
python3 prog3.py
Run the program and collect as many images as required for our stop-motion project. Press Ctrl + C to exit the program and open the File Manager to see your images.
Rendering the video
We are now ready to render the video from the sequence of images we just collected. We will begin rendering the video by entering the terminal window. Navigate to the stopmotion folder with the following command:
cd /home/pi/stopmotion
Then, execute the following command to begin rendering the video:
avconv -r 10 -qscale 2 -i frame%03d.jpg animation.mp4
We will now see what each part of this command does:
-r
specifies the frame rate for the video, which is currently set at 10-qscale
specifies the quality for the video and can range from 2-5-i
specifies the input file
Once the rendering is complete, you can play it with the following command:
omxplayer animation.mp4
Congratulations! You have just created your own stop-motion animation creator! Go ahead and try it out. Build your own interesting animations and upload them on YouTube.
Summary
In this chapter, we revised how to use Pi Camera with the Raspberry Pi and take photos with it. We learned a neat trick to correct our photos if they are upside down. We also learned how to use a GPIO to use a tactile button to act as the trigger for our images.
Once we obtained the images required for our animation, we proceeded to combine those images in a video, where we specified the frame rate and quality. Finally, we rendered the video using the ffmpeg
library.
In the next chapter, we will learn about the popular open source image processing library, OpenCV, and get it set up on the Raspberry Pi. We will also implement some interesting projects, such as video processing, logical operations on an image, colorspace conversions, and much more!