Variable assignment, empty space, and writing our own programs
First, for Python language, an empty space or spaces is very important. For example, if we accidently have a space before typing pv=100
, we will see the following error message:
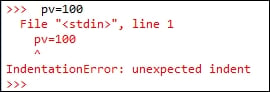
The name of the error is called IndentationError
. The reason is that, for Python, indentation is important. Later in the chapter, we will learn that a proper indentation will regulate/define how we write a function or why a group of codes belongs to a specific topic, function, or loop.
Assume that we deposit $100 in the bank today. What will be the value 3 years later if the bank offers us an annual deposit rate of 1.5%? The related codes is shown here:
>>>pv=100 >>>pv 100 >>>pv*(1+0.015)**3 104.56783749999997 >>>
In the preceding codes, **
means a power function. For example, 2**3
has a value of 8
. To view the value of a variable, we simply type its name; see the previous example. The formula used is given here:

Here, FV is the future value, PV is the present value, R is the period deposit rate while n is the number of periods. In this case, R is the annual rate of 0.015 while n is 3. At the moment, readers should focus on simple Python concepts and operations.
In Chapter 3, Time Value of Money, this formula will be explained in detail. Since Python is case-sensitive, an error message will pop up if we type PV
instead of pv
; see the following code:
>>>PV NameError: name 'PV' is not defined >>>Traceback (most recent call last): File "<stdin>", line 1, in <module>
Unlike some languages, such as C and FORTRAN, for Python a new variable does not need to be defined before a value is assigned to it. To show all variables or function, we use the dir()
function:
>>>dir() ['__builtins__', '__doc__', '__loader__', '__name__', '__package__', '__spec__', 'pv'] >>>
To find out all built-in functions, we type dir(__builtings__)
. The output is shown here:
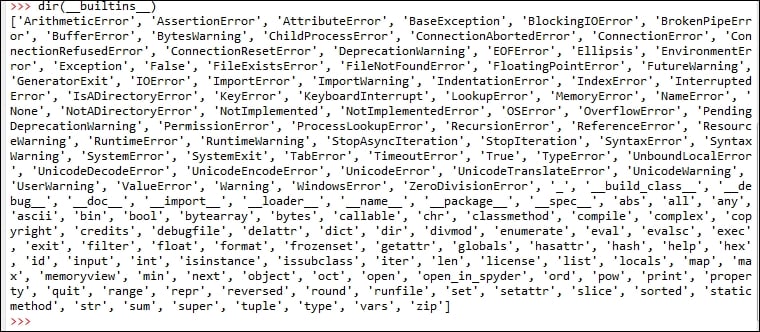