Gradle has now become the de facto build tool for Android, and it is very powerful. It’s great for automating tasks without compromising on maintainability, usability, flexibility, extensibility, or performance. In this recipe, we will see how to use Gradle to run Kotlin code.
How to use Gradle to run Kotlin code
Getting ready
We will be using IntelliJ IDEA because it provides great integration of Gradle with Kotlin, and it is a really great IDE to work on. You can also use Android Studio for it.
How to do it...
In the following steps, we will be creating a Kotlin project with the Gradle build system. First, we will select the Create New Project option from the menu. Then, follow these steps:
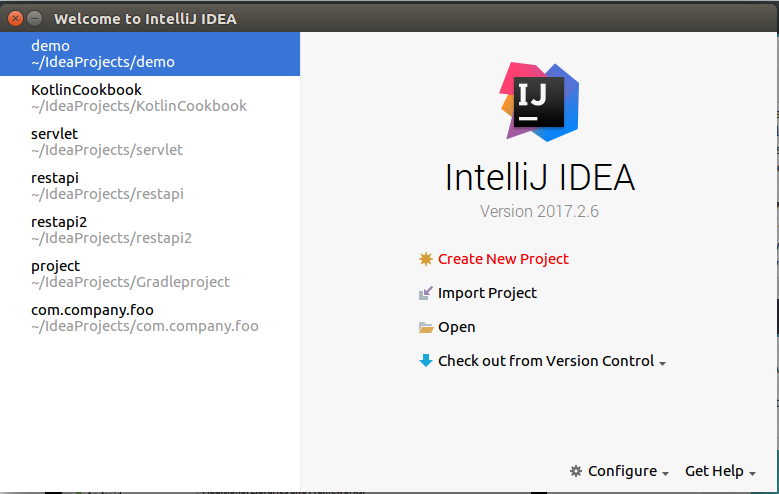
- Create the project with the Gradle build system:
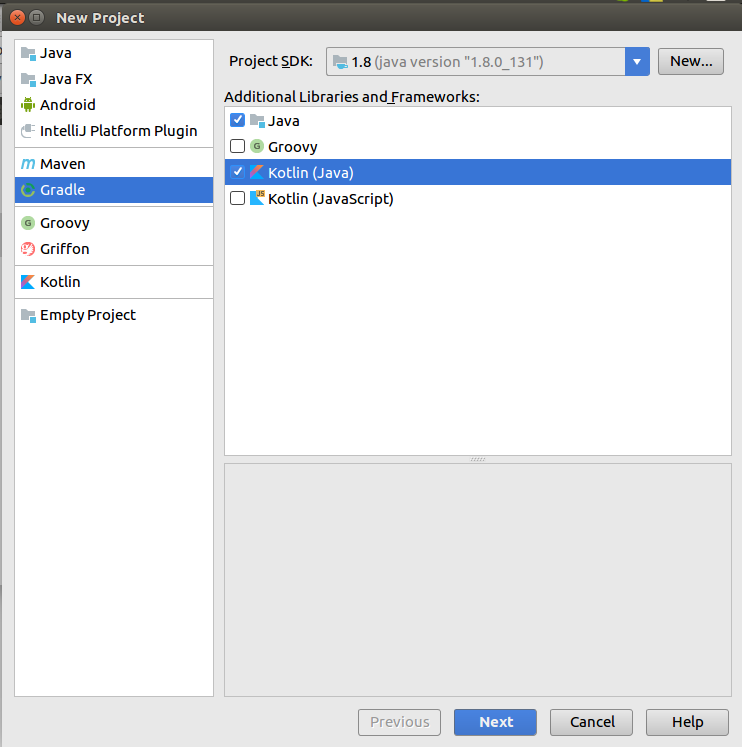
- After you have created the project, you will have the build.gradle file, which will look something like the following:
version '1.0-SNAPSHOT'
buildscript {
ext.kotlin_version = '1.1.4-3'
repositories {
mavenCentral()
}
dependencies {
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
apply plugin: 'java'
apply plugin: 'kotlin'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile "org.jetbrains.kotlin:kotlin-stdlib-jre8:$kotlin_version"
testCompile group: 'junit', name: 'junit', version: '4.12'
}
compileKotlin {
kotlinOptions.jvmTarget = "1.8"
}
compileTestKotlin {
kotlinOptions.jvmTarget = "1.8"
}
- Now we will create a HelloWorld class, which will have a simple main function:
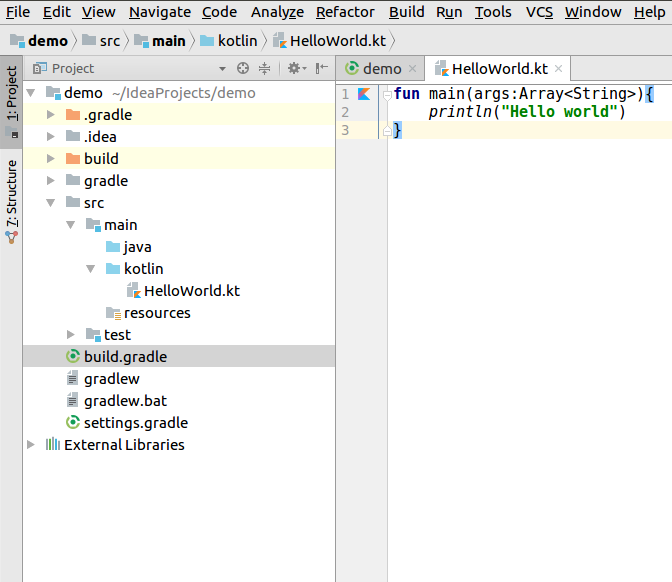
- Now, it would be really cool to run this code directly. To do so, we will use the gradle run command. However, before that, we need to enable the application plugin, which will allow us to directly run this code. We need to add two lines in the build.gradle file to set it up:
apply plugin: 'application'
mainClassName = "HelloWorldKt"
- After this, you can type gradle run in the terminal to execute this file, and you will see the output of the method, as shown:
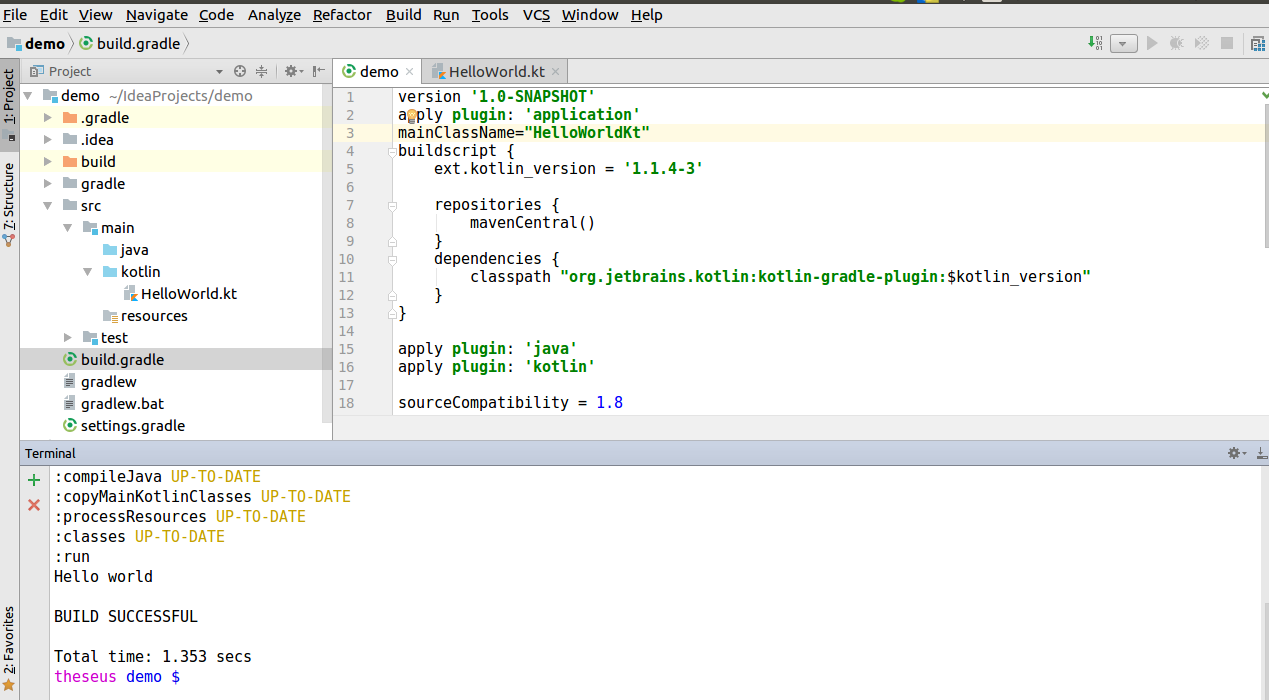
There's more...
The default structure of the project, when you create a new project in IntelliJ, is as illustrated:
project
- src
- main (root)
- kotlin
- java
If you want to have a different structure of the project, you should declare it in build.gradle. You can do it by adding the following lines in build.gradle.
The corresponding sourceSets property should be updated if not using the default convention:
sourceSets {
main.kotlin.srcDirs += 'src/main/myKotlin'
main.java.srcDirs += 'src/main/myJava'
}
Though you can keep Kotlin and Java files under the same package, it’s a good practice to keep them separated.
See also
Check out the How to build a self-executable jar with Gradle and Kotlin recipe in this chapter.