In this section, we will look at the architecture and requirements of building a serverless microservice. The rest of the chapter is heavily hands-on in terms of configuration in the AWS Management Console, but also Python code. The Python code follows basic design patterns and is kept simple, so that you can understand and easily adapt it for your own use cases.
Building a serverless microservice data API
Serverless microservice data API requirements
We want to create a microservice that is able to serve a web visits count, for a specific event, of the total users browsing your website.
Query string
For a specific EventId passed into the request, we want to retrieve a daily web visits count (already collected by another system) as a response. We are going to be querying by EventId and startDate; where we want to retrieve all web visit counts after the startDate. The URL, with its parameter, will look as follows:
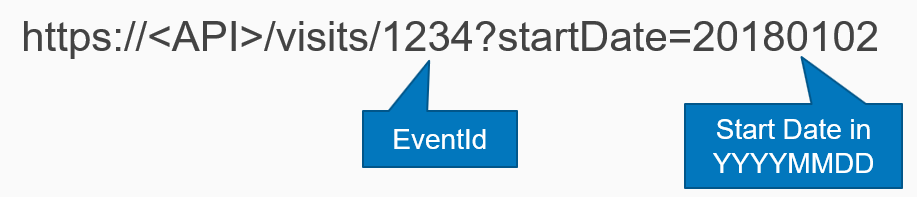
Here, you can see that we have EventId as resource 1234, and a startDate parameter formatted in the YYYYMMDD format. In this case, it's 20180102. That is the request.
We can either enter this request in the browser or programmatically, and the response that we want to get is from the NoSQL database, where the live data is stored. The actual response format will be in JSON coming back from the database, through a Lambda function, to be presented to either the user, or the other service that has queried the API programmatically. We also want this API to scale very easily and be very cost-effective; that is, we don't want to have a machine running all the time, for example, as that would cost money and need to be maintained. We only want to pay when actual requests are made.
Now the following diagram:
Here is an example of the time-series data that we're interested in, where we have EventId that is for event 324, and we have the date in the format EventDay, which is in October 2017, and we have a total EventCount of web events in the right-hand column in the table. You can see that on the October 10, 2017, EventId with 324 had an EventCount of 2, which means there was a total daily count of visits equal to 2 on that specific day for that event. The next day it's 0, as there is no entry for the 11th. Then, it increased to 10 on the 12th, 10 on the 13th, then it went down to 6, 0, 6, and 2. Note that when we have no data in the table for a specific date, then it's 0.
This is the data we want the API to provide as a response in JSON, so another client application can chart it, as shown in the following chart:
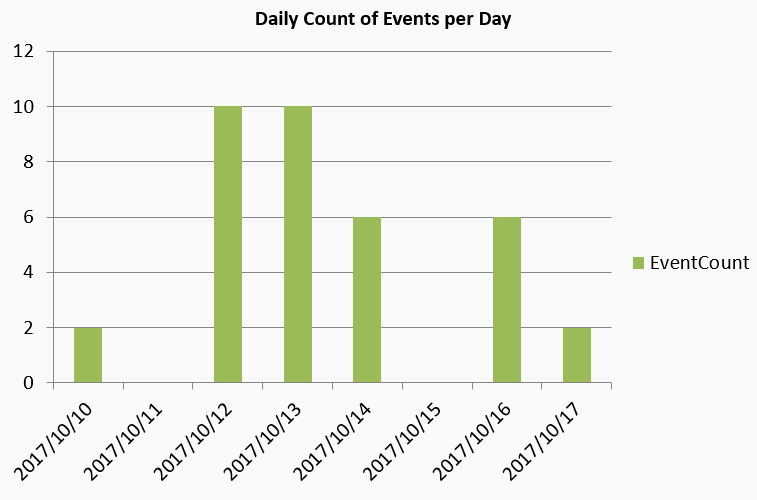
For example, if we plot the data in Excel, you would see this sort of chart, with the EventCount beginning at 2, with a gap on the October 11, where there was no visits for this specific user, then an increase to 10, 10, 6, then a gap on October 15, then up to 6 again on October 16.
Data API architecture
Now that we know the requirements and shape of the data that we want to return, we will talk about the overall architecture. Again, the whole stack will rely on the JSON format for data exchange between all the services.
The following diagram shows what we have in the the request:
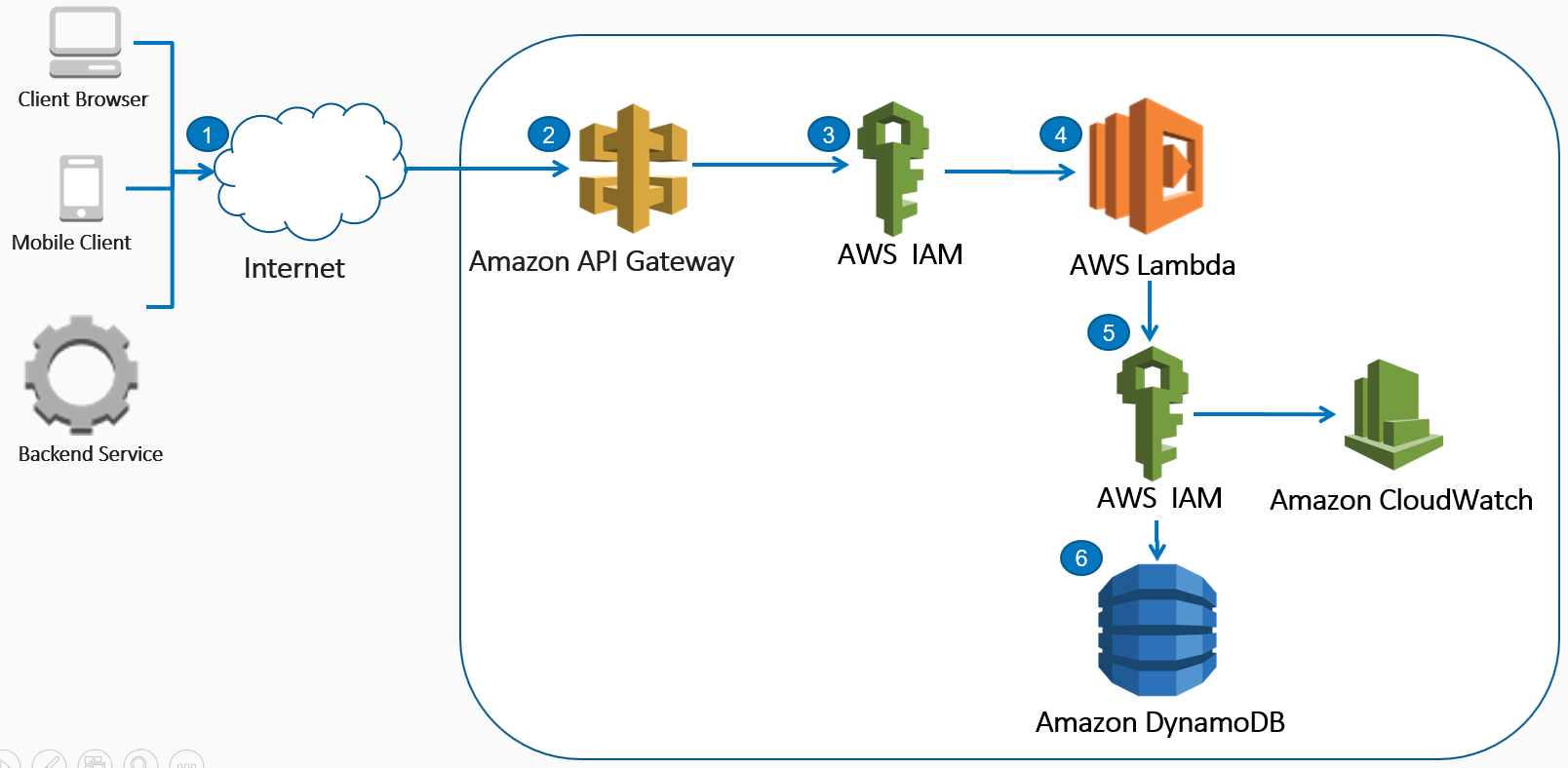
The request flow is as follows:
- We have a client browser, mobile client, or a backend service on the internet.
- That will query our API Gateway, passing the request with the EventId and the startDate as an optional URL parameter.
- This will get authenticated through an AWS IAM role.
- That will then launch the Lambda function.
- The Lambda function will then use a role to access DynamoDB.
- The Lambda will query Dynamo to search for that specific EventId. Optionally, the query will also include a specific startDate that is compared with EventDay. If EventDay is greater than startDate, then the records will be returned.
The following diagram shows what we have in the the response:
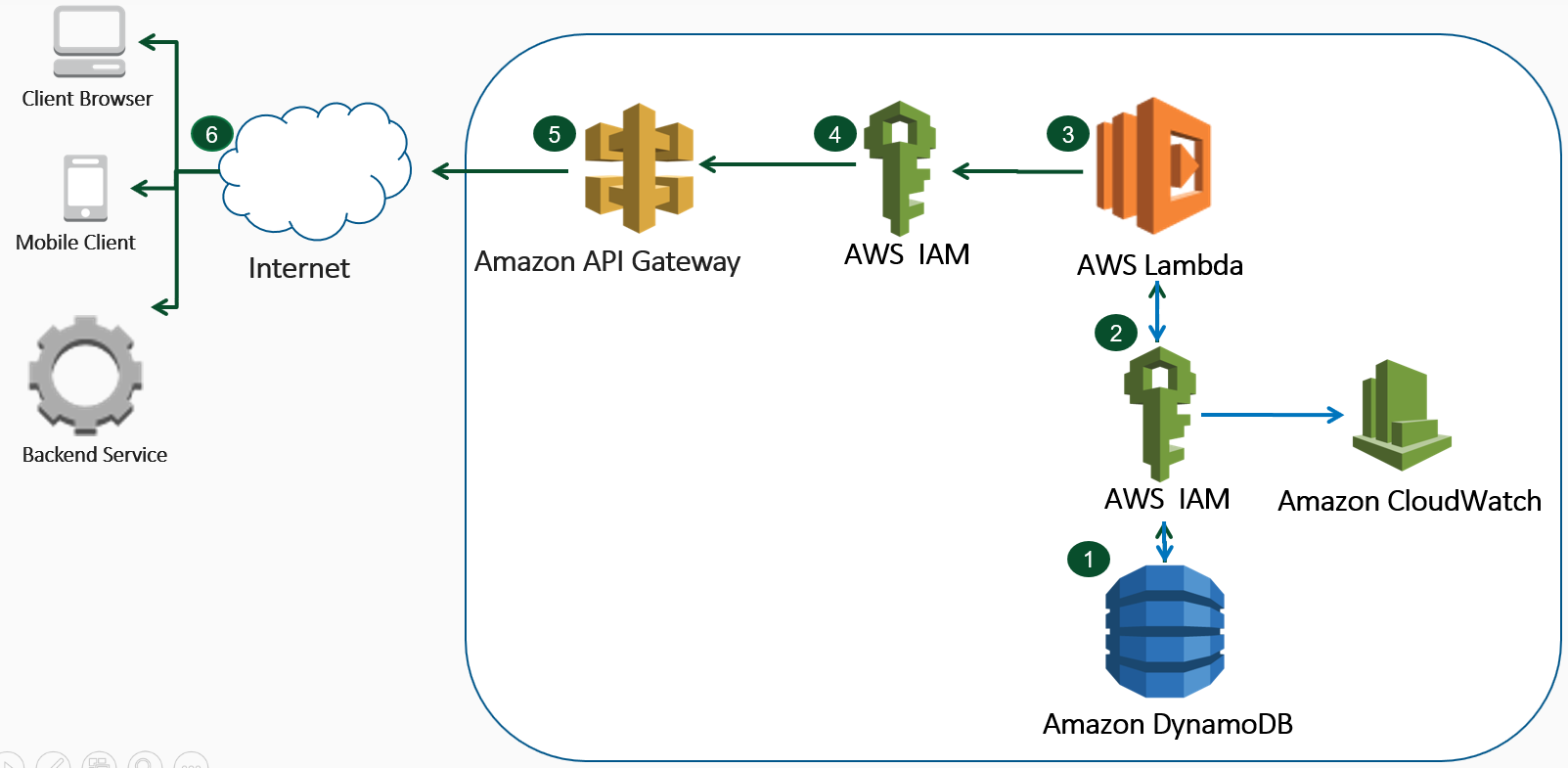
The response flow is as follows:
- The data will be returned from DynamoDB, as shown at the bottom-right of the preceding diagram
- This will be via the same IAM role associated with the Lambda function
- The JSON records from DynamoDB are returned to the Lambda function, which parses it into a JSON response
- That will get passed via the API Gateway role by the Lambda function
- It is passed back into API Gateway
- Eventually, it is returned to the client browser mobile client, or the backend service that made the initial request, so that it can be charted
We also have Amazon CloudWatch configured for monitoring requests, by providing dashboards for metrics and logs.