Integrating the PayPal API
In this section, we will set up the Webhooks that will enable us to receive a notification related to the payment in the ESP32. To set up the Webhooks, we will complete the following steps:
- Firstly, we will go to https://www.webhook.site and get a unique URL, as highlighted in the following figure:
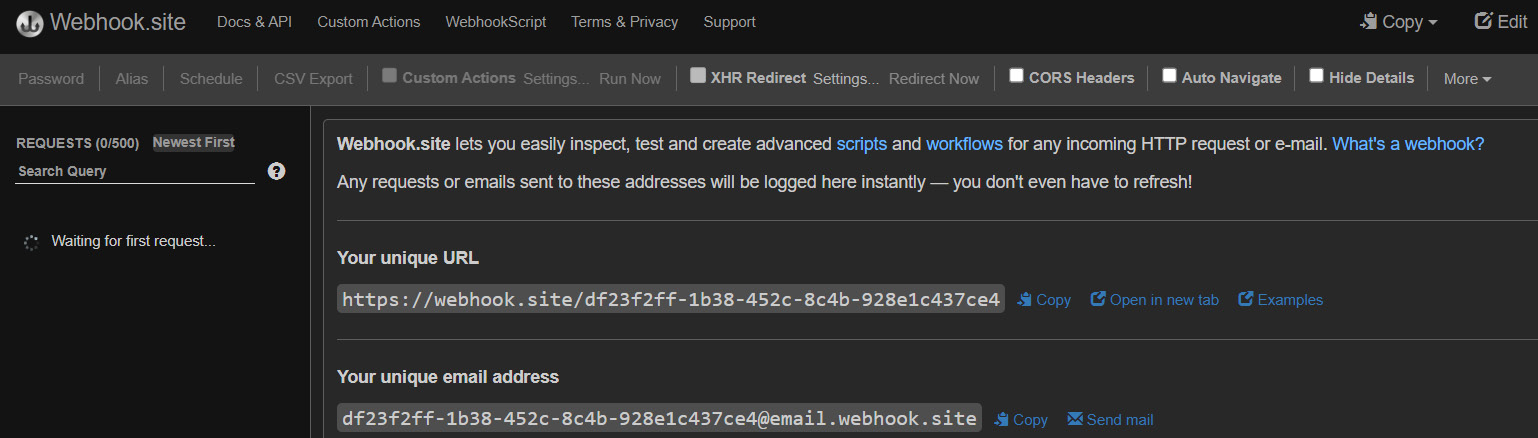
Figure 7.4 – A unique URL on Webhook.site
- Then, to test our Webhook, we will use the PayPal Instant Payment Notification (IPN) simulator. It will help us to simulate the payment notification for testing. To access the IPN simulator, go to https://developer.paypal.com/dashboard/ipnsimulator using your web browser.
- Paste the unique URL into the IPL URL handler, and select Web Accept as the transaction type.
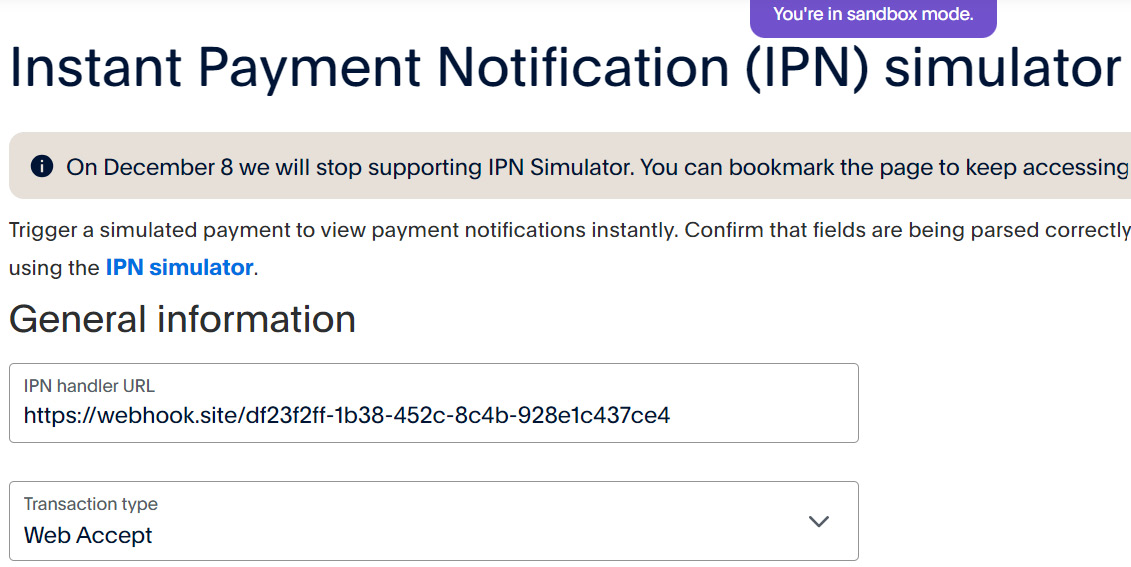
Figure 7.5 – The PayPal IPN simulator
- For testing, leave all other values to default, and then go to the bottom of the page and click on Send IPN.
...