I assume that you have installed Node.js, npm, and Visual Studio Code and are ready to use them for development. Now let us create an Angular application by cloning the Git repository and performing the following steps:
- Open the Node.Js command prompt and execute the following command:
git clone https://github.com/angular/quickstart my-angular
Open the cloned my-angular application using Visual Studio Code. This command will clone the Angular quickstart repository and creates an Angular application named my-angular for you with all the boilerplate codes required.
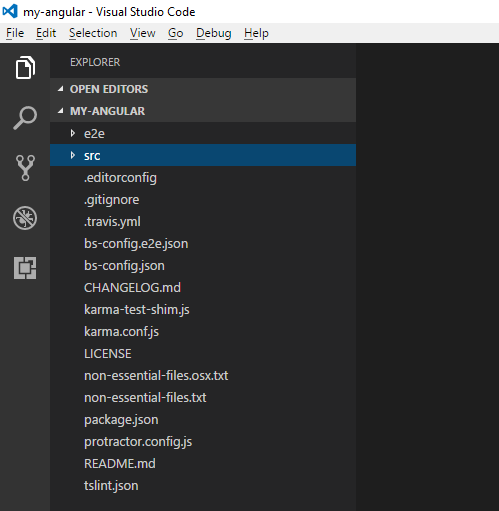
The folder structure and the boilerplate code are organized according to the official style guide in https://angular.io/docs/ts/latest/guide/style-guide.html. The src folder has the code files related to application logic, and the e2e folder has the files related to end-to-end testing. Don't worry about other files in the application now. Let's focus on package.json for now
- Click on the package.json file, and it will have information about the configurations of the metadata and project dependencies. Here is the content of the package.json file:
{ "name":"angular-quickstart", "version":"1.0.0", "description":"QuickStart package.json from the documentation,
supplemented with testing support", "scripts":{ "build":"tsc -p src/", "build:watch":"tsc -p src/ -w", "build:e2e":"tsc -p e2e/", "serve":"lite-server -c=bs-config.json", "serve:e2e":"lite-server -c=bs-config.e2e.json", "prestart":"npm run build", "start":"concurrently \"npm run build:watch\" \"npm run
serve\"", "pree2e":"npm run build:e2e", "e2e":"concurrently \"npm run serve:e2e\" \"npm run
protractor\" --kill-others --success first", "preprotractor":"webdriver-manager update", "protractor":"protractor protractor.config.js", "pretest":"npm run build", "test":"concurrently \"npm run build:watch\" \"karma start
karma.conf.js\"", "pretest:once":"npm run build", "test:once":"karma start karma.conf.js --single-run", "lint":"tslint ./src/**/*.ts -t verbose" }, "keywords":[ ], "author":"", "license":"MIT", "dependencies":{ "@angular/common":"~4.0.0", "@angular/compiler":"~4.0.0", "@angular/core":"~4.0.0", "@angular/forms":"~4.0.0", "@angular/http":"~4.0.0", "@angular/platform-browser":"~4.0.0", "@angular/platform-browser-dynamic":"~4.0.0", "@angular/router":"~4.0.0", "angular-in-memory-web-api":"~0.3.0", "systemjs":"0.19.40", "core-js":"^2.4.1", "rxjs":"5.0.1", "zone.js":"^0.8.4" }, "devDependencies":{ "concurrently":"^3.2.0", "lite-server":"^2.2.2", "typescript":"~2.1.0", "canonical-path":"0.0.2", "tslint":"^3.15.1", "lodash":"^4.16.4", "jasmine-core":"~2.4.1", "karma":"^1.3.0", "karma-chrome-launcher":"^2.0.0", "karma-cli":"^1.0.1", "karma-jasmine":"^1.0.2", "karma-jasmine-html-reporter":"^0.2.2", "protractor":"~4.0.14", "rimraf":"^2.5.4", "@types/node":"^6.0.46", "@types/jasmine":"2.5.36" }, "repository":{ } }
- Now we need to run the npm install command in a command window, navigating to the application folder to install the required dependencies specified in package.json:
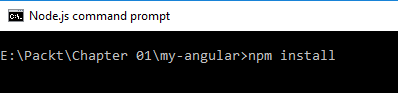
Now, you will have all the dependencies added to the project under the node_modules folder, as shown in this screenshot:
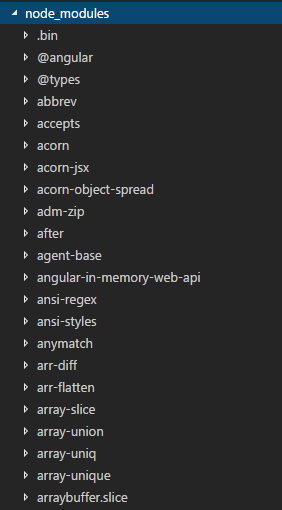
- Now, let's run this application. To run it, execute the following command in the command window:
npm start
Running this command builds the application, starts the lite server, and hosts the application onto it.
Open any browser and navigate to http://localhost:3000/; and you will get the following page displayed, which is rendered through our Angular application:
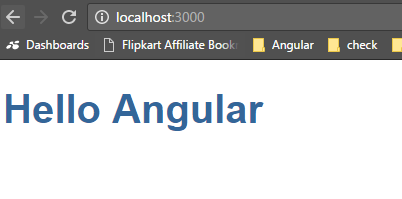
Let's now walk through the content of index.html. Here is the content of index.html:
<!DOCTYPE html>
<html>
<head>
<title>Hello Angular 4</title>
<base href="/">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="styles.css">
<!-- Polyfill(s) for older browsers -->
<script src="node_modules/core- js/client/shim.min.js"> </script>
<script src="node_modules/zone.js/dist/zone.js"> </script>
<script src="node_modules/systemjs/dist/system.src.js"> </script>
<script src="systemjs.config.js"></script>
<script>
System.import('main.js').catch(function(err){
console.error(err); });
</script> </head>
<body>
<my-app>My first Angular 4 app for Packt Publishing...</my-app>
</body>
</html>
Notice that scripts are loaded using System.js. System.js is the module loader that loads modules during runtime.
Voila! Finally, our first Angular app is up-and-running. So far, we have seen how to create an Angular application by cloning the official quickstart repository from GitHub. We ran the application and saw it in the browser successfully.