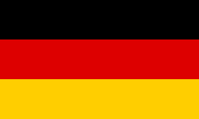




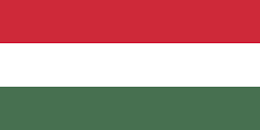

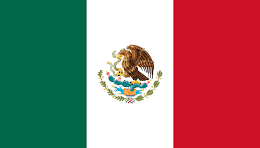
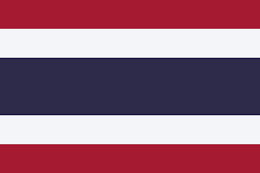
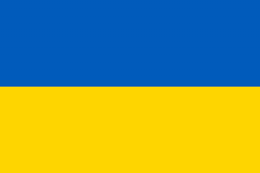
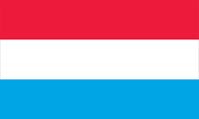

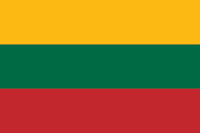

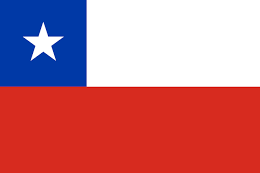
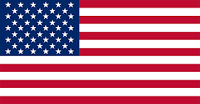

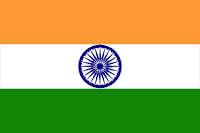
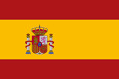

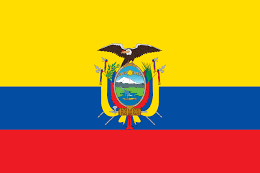

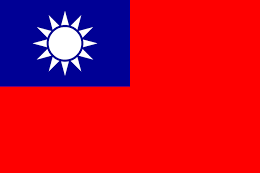

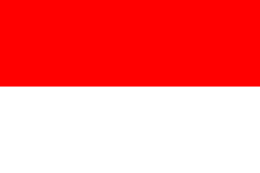
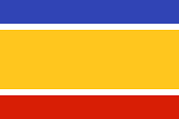
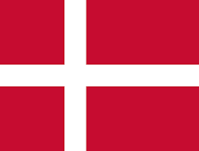
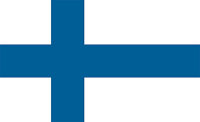


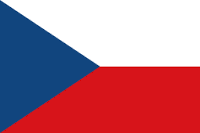
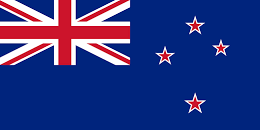
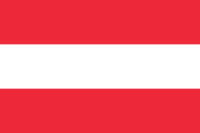
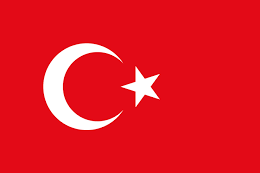
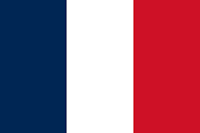
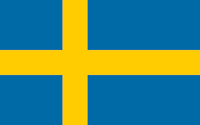
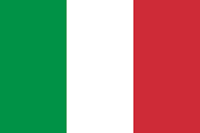
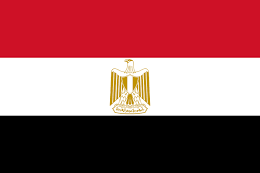

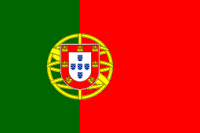
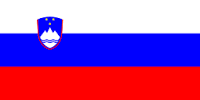

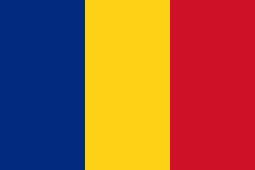
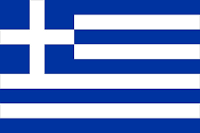

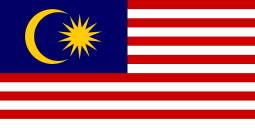
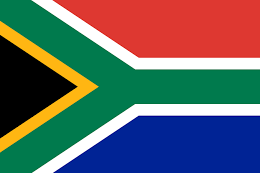

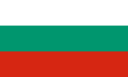
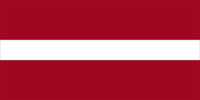


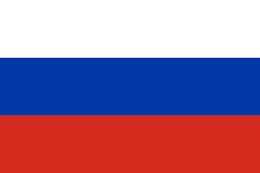
Dive deeper into the world of AI innovation and stay ahead of the AI curve! Subscribe to our AI_Distilled newsletter for the latest insights. Don't miss out – sign up today!
Virtual Personal Assistants are emerging as an important aspect of the rapidly developing Artificial Intelligence landscape. These intelligent, Artificial Intelligence assistants are capable of carrying out a wide range of tasks such as answering questions and providing advice on how to make process more efficient.
You're more easily getting your personal assistant built using the ChatGPT service from OpenAI. We'll explore the creation of virtual personal assistants using ChatGPT, complete with hands-on code examples and projected outputs in this advanced guide. Use ChatGPT, the world's most advanced language model created by OpenAI to create a virtual assistant that you can use.
There are certain prerequisites that need to be met before we embark on this journey:
pip install openai
Let's have a look at the following steps for creating a Virtual Personal Assistant with ChatGPT
To begin, we shall import the required libraries and set up an API key.
import openai
openai.api_key = "YOUR_OPENAI_API_KEY"
We're going to build an easy interaction based on text with our assistant. We will ask ChatGPT a question, and we shall receive an answer.
Input code:
def chat_with_gpt(prompt):
response = openai.Completion.create(
engine="davinci-codex",
prompt=prompt,
max_tokens=50 # Adjust as needed
)
return response.choices[0].text
# Interact with the assistant
user_input = input("You: ")
response = chat_with_gpt(f"You: {user_input}\nAssistant:")
print(f"Assistant: {response}")
Output:
You: What's the weather like today?
Assistant: The weather today is sunny with a high of 25°C and a low of 15°C.
We used ‘chat_with_gpt’, for interacting with ChatGPT to generate responses from user input. Users can input questions or comments and the function will send a request to ChatGPT. In the output, the assistant's answer is shown in a conversational format.
By making it a language translation tool, we can improve the assistant's abilities. Users can type a word in one language and an assistant will translate it to another.
Input Code:
def translate_text(input_text, target_language="fr"):
response = chat_with_gpt(f"Translate the following text from English to {target_language}: {input_text}")
return response
# Interact with the translation feature
user_input = input("Enter the text to translate: ")
target_language = input("Translate to (e.g., 'fr' for French): ")
translation = translate_text(user_input, target_language)
print(f"Translation: {translation}")
Output:
Enter the text to translate: Hello, how are you?
Translate to (e.g., 'fr' for French): fr
Translation: Bonjour, comment ça va?
To translate English text to the target language using ChatGPT, we are defining a function, ‘translate_text’. Users input text and the target language, which is returned in translation by this function. It uses the ability of ChatGPT to process natural languages in order to carry out accurate translation.
The creation of code fragments may also be assisted by our virtual assistant. It is especially useful for developers and programmers who want to quickly solve code problems.
Input Code:
def generate_code(question):
response = chat_with_gpt(f"Generate Python code to: {question}")
return response
# Interact with the code generation feature
user_input = input("You: ")
generated_code = generate_code(user_input)
print("Generated Python Code:")
print(generated_code)
Output:
You: Create a function to calculate the factorial of a number.
Generated Python Code:
def calculate_factorial(n):
if n == 0:
return 1
else:
return n * calculate_factorial(n - 1)
The user provides a question and the function sends a request to ChatGPT to generate code to answer it. In the output, a Python code is displayed.
It's even possible to make use of our Virtual Assistant as an organizer. A reminder of tasks or events can be set by users, which will be handled by an assistant.
Input code:
def set_reminder(task, time):
response = chat_with_gpt(f"Set a reminder: {task} at {time}.")
return response
# Interact with the reminder feature
task = input("Task: ")
time = input("Time (e.g., 3:00 PM): ")
reminder_response = set_reminder(task, time)
print(f"Assistant: {reminder_response}")
Output:
Task: Meeting with the client
Time (e.g., 3:00 PM): 2:30 PM
Assistant: Reminder set: Meeting with the client at 2:30 PM.
The code defines a function, ‘set_reminder’, which can be used to generate reminders based on the task and time. Users input their tasks and time, and the function requests a reminder to be sent to ChatGPT. The output will be printed with the assistant's answer and confirmation of this reminder.
In conclusion, we got to know the evolution of Virtual Personal Assistant using ChatGPT throughout this advanced guide. We've started with a basic text-based interaction, followed by three advanced examples: language translation, code generation, and setting reminders. There is no limit to the potential of Virtual Personal Assistants.
Integrating your assistant into various APIs, enhancing the ability to understand languages and making it useful for a variety of tasks will allow you to further expand its capabilities. Creating a tailored virtual assistant is now even easier to create and adapted to your individual needs, given the advancement of AI technologies.
Sangita Mahala is a passionate IT professional with an outstanding track record, having an impressive array of certifications, including 12x Microsoft, 11x GCP, 2x Oracle, and LinkedIn Marketing Insider Certified. She is a Google Crowdsource Influencer and IBM champion learner gold. She also possesses extensive experience as a technical content writer and accomplished book blogger. She is always Committed to staying with emerging trends and technologies in the IT sector.