Building images with Docker Compose
To demonstrate how to build a Docker image using Docker Compose, we need a small application. Proceed as follows:
- In the chapter’s folder (
ch11
), create a subfolder,step2
, and navigate to it:mkdir step2 && cd step2
- From the previous exercise, copy the
db
folder containing the database initialization script to thestep2
folder and also copy thedocker-compose.yml
file:$ cp -r ../step1/db .$ cp ../docker-compose.yml .
- Create a folder called
web
in thestep2
folder. This folder will contain a simple Express.js web application. - Add a file called
package.json
to the folder with this content:
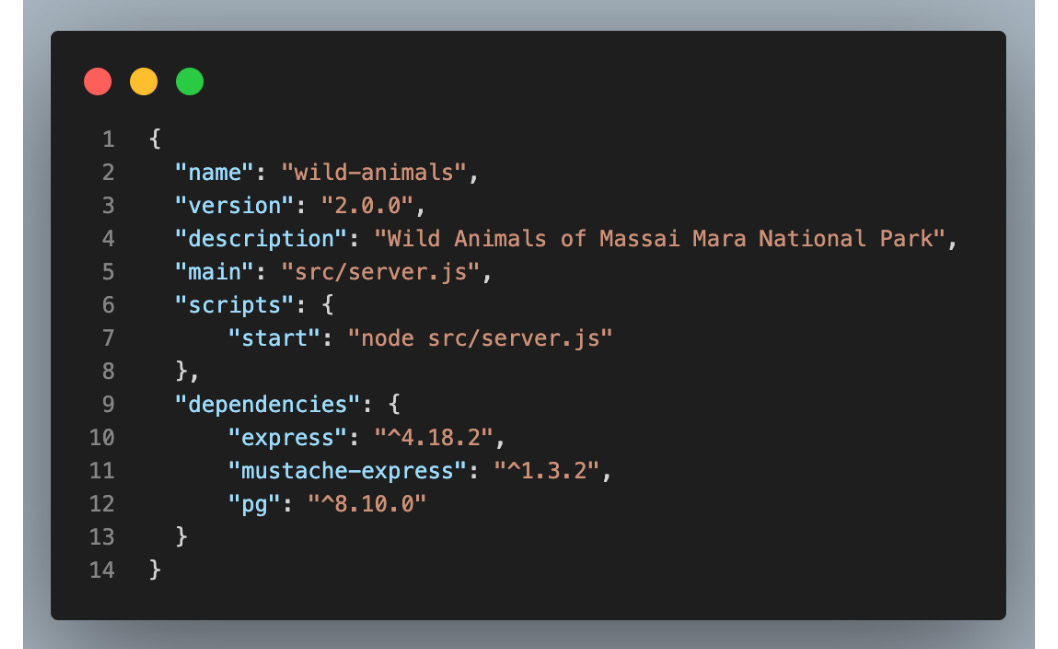
Figure 11.8 – The package.json file of the sample web application
Note
If you prefer not to type yourself, you can always download the files from the sample solution: https://github.com/PacktPublishing/The-Ultimate-Docker-Container-Book/tree/main/sample-solutions/ch11/step2.
- Create...