Generating passwords with Terraform
When provisioning infrastructure with Terraform, there are some resources that require passwords in their properties, such as account credentials, VMs, and database connection strings.
To ensure better security by not writing passwords as plaintext in the configuration, you can use a random
Terraform provider, which allows you to generate a random string that can be used as a password.
In this recipe, we will discuss how to generate a password with Terraform and assign it to a resource.
Getting ready
In this recipe, we need to provision a VM in Azure that will be provisioned with an administrator password generated dynamically by Terraform.
To do this, we will use an already existing Terraform configuration that provisions a VM in Azure.
The source code for this recipe is available at https://github.com/PacktPublishing/Terraform-Cookbook-Second-Edition/tree/main/CHAP02/password.
How to do it…
Perform the following steps:
- In the Terraform configuration file for the VM, add the following code:
resource "random_password" "password" { length = 16 special = true override_special = "_%@" }
- Then, in the code of the resource itself, modify the
password
property with the following code:resource "azurerm_linux_virtual_machine" "myterraformvm" { name = "myVM" location = "westeurope" resource_group_name = azurerm_resource_group.myterraformgroup.name computer_name = "vmdemo" admin_username = "uservm" admin_password = random_password.password.result .... }
How it works…
In Step 1, we added the Terraform random_password
resource from the random
provider, which allows us to generate strings according to the properties provided. These will be sensitive
, meaning that they’re treated as sensitive in the CLI by Terraform, so they will not be displayed to the user.
Then, in Step 2, we used its result (with the result
property) in the password
property of the VM.
The result of executing the terraform plan
command on this code can be seen in the following screenshot:
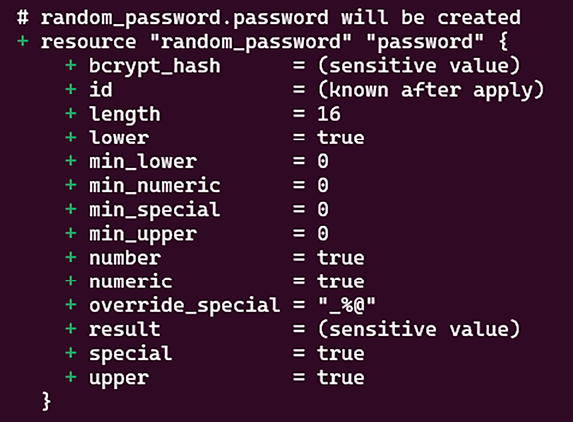
Figure 2.16: Generating a password with Terraform
As we can see, the result is (sensitive value)
.
Please note that the fact a property is sensitive in Terraform means that it cannot be displayed when using the Terraform plan
and apply
commands in the console output display.
On the other hand, it will be present in clear text in the Terraform state file.
See also
- To find out more about the
random
provider, read the following documentation: https://registry.terraform.io/providers/hashicorp/random/. - Documentation regarding sensitive data in Terraform state files is available at https://www.terraform.io/docs/state/sensitive-data.html.