Writing Python scripts
We are now familiar with the basic concepts of Python. Now we will write an actual program or script in Python.
Ask for the input of a country name, and check whether the last character of the country is a vowel:
countryname=input("Enter country name:") countryname=countryname.lower() lastcharacter=countryname.strip()[-1] if 'a' in lastcharacter: print ("Vowel found") elif 'e' in lastcharacter: print ("Vowel found") elif 'i' in lastcharacter: print ("Vowel found") elif 'o' in lastcharacter: print ("Vowel found") elif 'u' in lastcharacter: print ("Vowel found") else: print ("No vowel found")
Output of the preceding code is as follows:
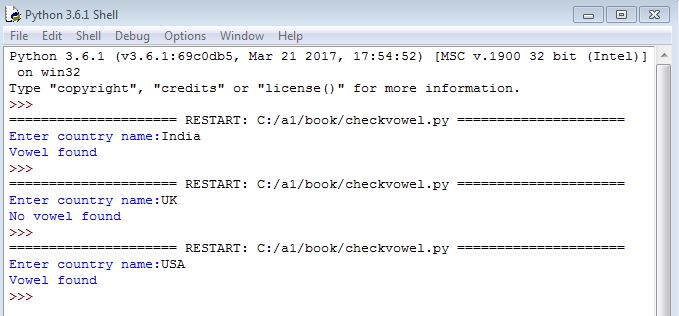
- We ask for the input of a country name. The
input()
method is used to get an input from the user. The value entered is in the string format, and in our case thecountryname
variable has been assigned the input value. - In the next line,
countryname.lower()
specifies that the input that we receive needs to converted into...