Using callables with signals
In this recipe, we will see how callables can be used with signals. We will also look at the call
and bind
callable methods. Callables can be held in variables and passed into functions. As such, you can use them in arrays and in dictionaries as the key or the value.
Getting ready
For this recipe, create a new scene by clicking + to the right of the current Scene tab and adding Node2D. Select Save Scene As and name it Callables
.
How to do it…
Let’s start by creating a Button
node and referencing it to the button
variable:
- Add a script named
Callables
to Node2D and delete all of the default lines except line 1 and the_ready()
function. - In the new scene named Callables that you created, add a
Button
node and make it big enough to see. - Let’s use
@onready
and create a variable calledbutton
to reference our Button node:1 extends Node2D 2 3 @onready var button = $Button
- On line 8, we create a function called
signal_callable()
:8 func signal_callable(): 9 print("This method was called by the button pressed signal.")
- On line 5, in the
_ready()
function, we connect a callable signal to thesignal_callable()
function:5 func _ready(): 6 button.pressed.connect(signal_callable)
- Now click the Run the current scene button or hit the F6 key.
- Let’s use the
.bind
method when we connect the signal. On line 6, add.bind
aftersignal_callable
:6 button.pressed.connect(signal_callable.bind("binding_"))
- We need to add a parameter to the
signal_callable
function:8 func signal_callable(param): 9 print(param, "This method was called by the button pressed signal.")
- Now click the Run the current scene button or hit the F6 key.
- Let’s add a new function on line 11 called
player_text
:10 func player_text(param: String): 11 print(param)
- Go to line 7, hit the Tab key, and add more code to the
ready
function. - Let’s create a variable called
pt
equal toplayer_text
:7 var pt = player_text 8 pt.call("Hello, NPC!")
- Now click the Run the current scene button or hit the F6 key.
How it works…
We added a script called Callables
to Node2D and deleted everything in the script except line 1 and the _ready()
function. Then we created a Button
node in the Scene tab. In the Callables
script, we used @onready
to declare a variable called button
to the Button
node.
We created a function that we want to run when the button pressed
signal is true
. Notice that we don’t have to connect the signal in the editor. We can use any method that we want.
In the _ready()
function, we used the button reference to connect the signal_callable
function when the signal pressed is true
, which happens when the button is pressed.
We ran the current scene. In the console, we saw This method was called by the button pressed signal. after we clicked the button.
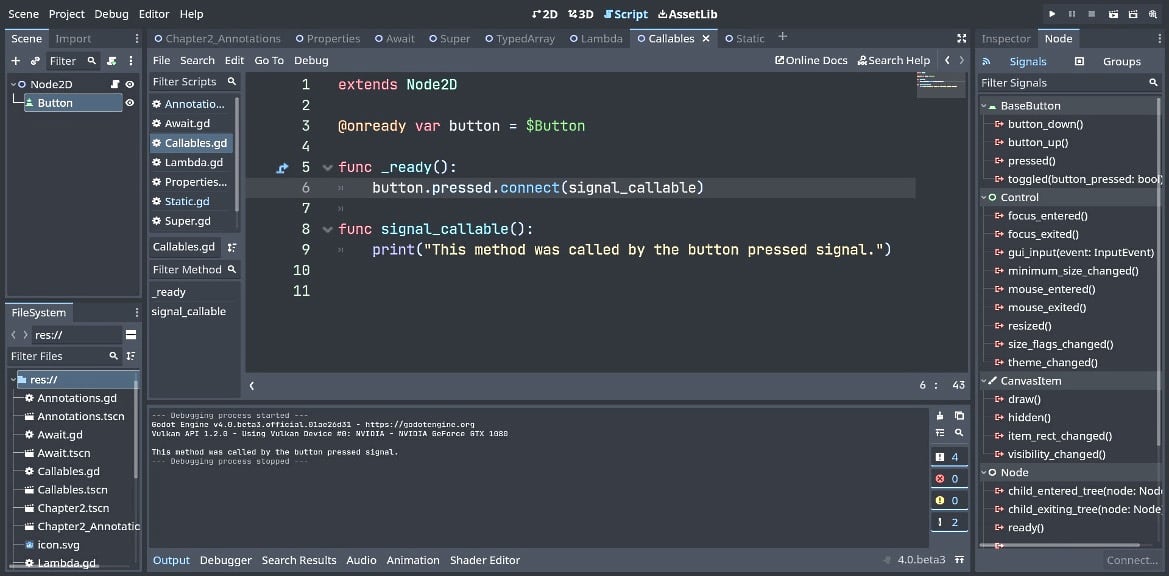
Figure 2.11 – Using callables with signals (code for steps 4–6)
We used the callable bind
method. In line 6, we added .bind
like this:
button.pressed.connect(signal_callable.bind("binding_")).
We needed to add a parameter to the function and inside of the print
statement to see what we added with bind
.
We ran the current scene. In the console, we saw binding_This method was called by the button pressed signal. printed after we clicked the button.
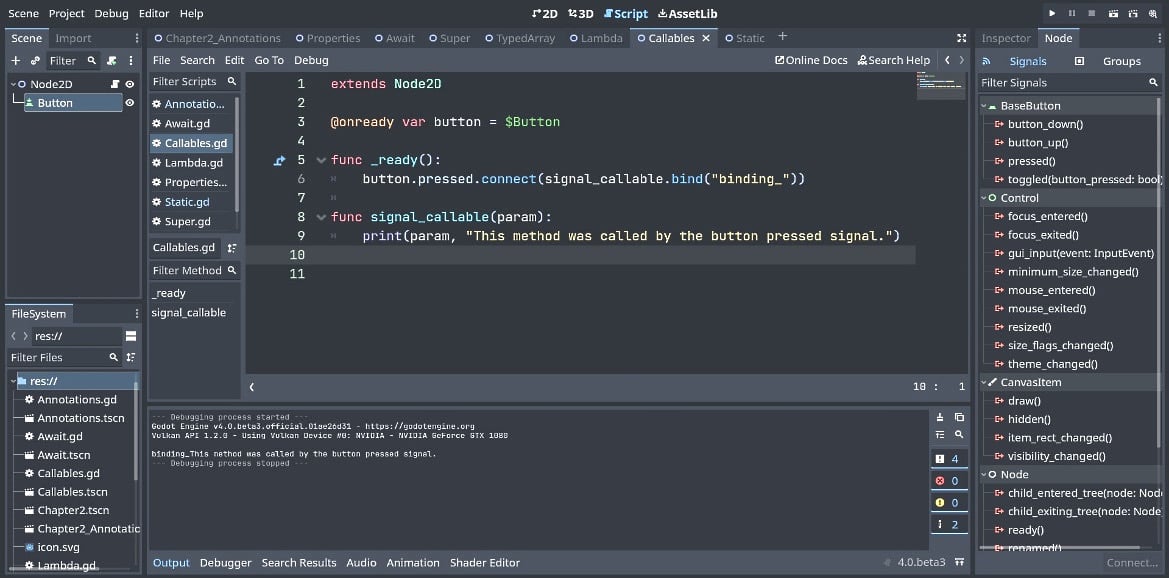
Figure 2.12 – Using callable with the bind method (code for steps 7–9)
We added a function called player_text
, which takes a parameter called param
that only accepts strings. It prints out the parameter that will be passed in when we use .call
.
We created a variable for the player_text()
function When you enter var pt = player_text
, autocomplete wants to add the ()
at the end – make sure you delete it. We converted the function into a variable, so now we can use the function in arrays, dictionaries, or in any other way you can use a variable.
We ran it to see Hello, NPC! printed in the console.
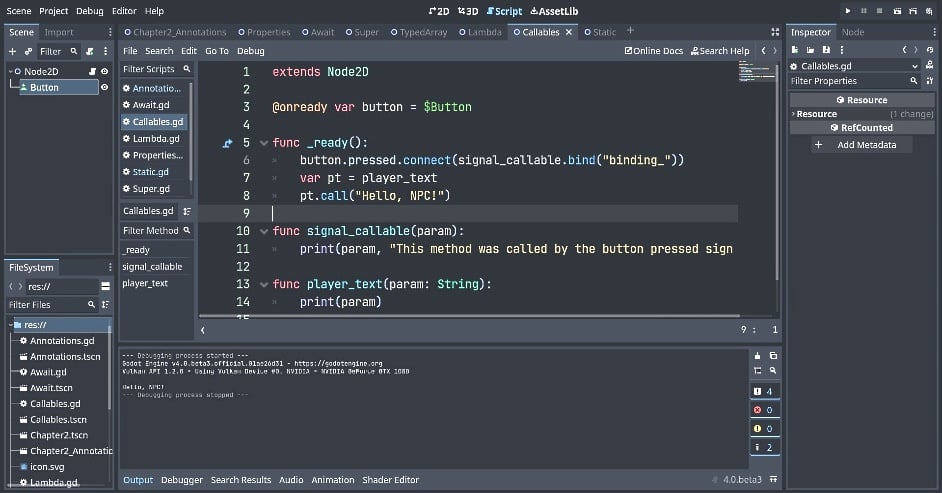
Figure 2.13 – Using .call() with a variable of the function (code for steps 10–13)