Dealing with strings in Python is very simple: you can search, replace, change character case, and perform other manipulations with ease:
To search for a string, you can use the find method like this:
#!/usr/bin/python3
str = "Welcome to Python scripting world"
print(str.find("scripting"))

The string count in Python starts from zero too, so the position of the word scripting is at 18.
You can get a specific substring using square brackets like this:
#!/usr/bin/python3
str = "Welcome to Python scripting world"
print(str[:2]) # Get the first 2 letters (zero based)
print(str[2:]) # Start from the second letter
print(str[3:5]) # from the third to fifth letter
print(str[-1]) # -1 means the last letter if you don't know the length
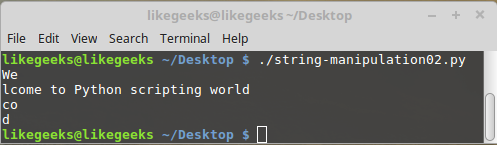
To replace a string, you can use the replace method like this:
#!/usr/bin/python3
str = "Welcome to...