Component-based architecture
We have reached the final section of our introductory discussion and we are almost ready to start coding. This section is going to introduce the concept of component-based architecture. Even if you are already familiar with this topic, I suggest you continue reading this chapter as it will support some of the decisions we will make later in the course of the book.
In this section, we are going to cover how web development worked before this concept was introduced and we will then discuss how component-based architecture has shaped the web development industry as we know it.
One page at a time
If you have been a developer for as long as I have been, you have probably worked with languages and frameworks that were not flexible in the way the pages were defined and developed. Using .NET and PHP a few years ago would have meant that each web page was created using a single file (disclaimer: some languages had the definition of “partials”.
This worked well until JS started to be used in the frontend and shook the ecosystem. JS changed sites from static pages to very dynamic entities and in doing so pushed for something more dynamic that would not work with the previous development tools.
Let’s take into consideration a standard website homepage, such as the following one:
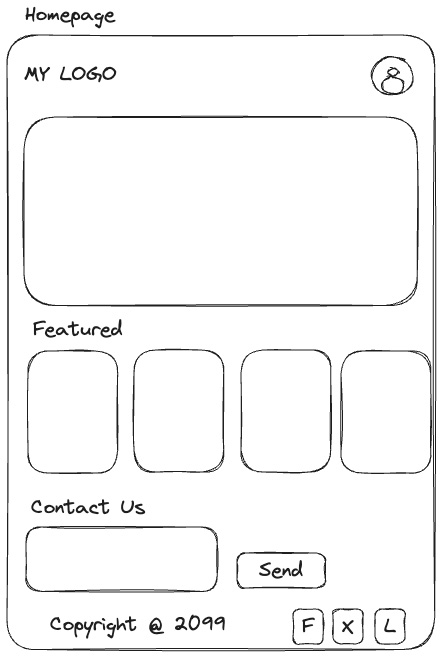
Figure 1.2: Wireframe of a standard homepage
This site follows a standard layout with a header and a footer, a banner, some featured content, and a Contact Us form. Imagine having all this in one single HTML file. Back in the day, this was probably all held in one single HTML file with a shared stylesheet, for example a CSS (Cascading Style Sheet) file. As mentioned previously, things started to change in the industry and JS started to be used more and more.
In the preceding scenario, JS was probably just used to add some basic interactivity like slideshow animation in the banner, some fancy scrolling i products list or to handle form submission in the Contact Us form.
Long story short, this change slowly shaped the industry toward frontend libraries and frameworks. These libraries and frameworks aimed to help manage and simplify the hundreds of lines of code produced in JS and they did so by introducing component-based architecture.
Breaking things down into small units was not something new in the industry, as the backend framework already had this notion with the use of object-oriented programming, but it was an innovation in the frontend side of the industry.
From one page to many components
The term component-based development (CBD) is a pattern in which the UI of a given application is broken down into multiple “components.” Breaking down big pages into small individual units reduces the complexity of the application and helps the developer focus on the individual scope and responsibility of each section.
All of today’s frontend frameworks are built on top of this pattern and today’s frontend development is driven by architecture based on CBD.
Let’s look at the previous example of the home page and see how we could split this into small isolated components.
The home page would be broken down into the following components:
- Header: A component that will include the logo and the logic used to display account information such as the avatar
- The slideshow: A reusable component used to display slideshow images
- Featured: A component used to display featured articles
- Contact Us: A component including all the logic required to validate and submit our form
- Footer: A static component that will include some copy and social links

Figure 1.3: Wireframe of a dashboard divided into different sections, such as header, slideshow, featured, Contact Us, and footer
As we will see in a few minutes, the components displayed in Figure 1.3 are just an example, as a fully defined CBD will actually break things even further all the way to the single HTML element. What this means is that not only the page is made of components, but components are made up of smaller components too.
Breaking down things into smaller units has many benefits. Let’s analyze some of these characteristics:
- Reusability: CBD provides you with the possibility to create components that can be reused within your application. (In our example we could reuse the header, footer, slideshow, and even the featured component.)
- Encapsulation: Encapsulation is defined as the ability for each component to be “self-contained.” All styles, HTML, and JS logic are “encapsulated” within the scope of a given component.
- Independence: Due to encapsulation, each component is independent and does not share (or is not supposed to share) responsibility with other components. This allows components to be used in different contexts (for example, the ability to use the feature component on a different page of the site).
- Extensibility: Due to the component being “self-contained” and independent, we are able to extend it with limited risk.
- Replaceability: The component can easily be swapped out with other components or be removed without risk.
It is clear from the preceding list that using CBD brings many benefits to the hands of a frontend developer. As we will experience in the course of this book, the ability to break an application down into small units is extremely beneficial for new developers as it allows the individual topics to be broken down and really focuses our attention on what matters the most.
Vue.js implements component-based architecture with the use of a Single-File Component (SFC). These files are denoted by the .vue
extension and encapsulate styles, HTML, and logic (JS or Typescript) in the same file. SFC will be clearly introduced later in the book.
Atomic design
In this last section, we are going to understand how we will structure our components during the course of the book.
The folder structure of components is something that has not been standardized yet around the industry and this can differ from developer to developer.
In our case, we are going to follow what is known in the industry as “atomic design.” This is described as:
The Atomic Design methodology created by Brad Frost (https://bradfrost.com/) is a design methodology for crafting robust design systems with an explicit order and hierarchy - blog.kamathrohan.com.
The atomic design pattern follows the same concept described in chemistry and the composition of matter. If you want to go into more detail on this subject, I suggest you read the following article: https://blog.kamathrohan.com/atomic-design-methodology-for-building-design-systems-f912cf714f53.
In this book, we are going to follow the hierarchy proposed in this methodology by breaking down our applications into “sub-atomic,” atoms, molecules, organisms, templates and pages.
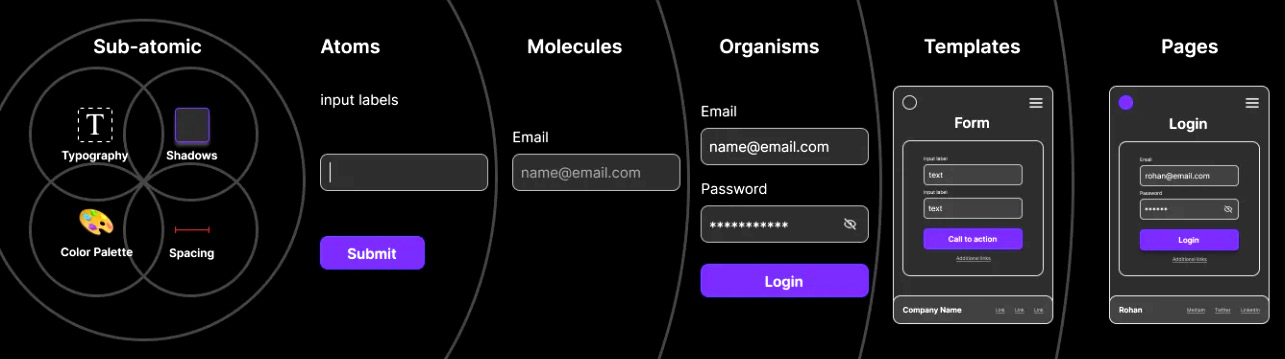
Figure 1.4: Visual explanation of the different levels offered by atomic design (source: https://blog.kamathrohan.com/atomic-design-methodology-for-building-design-systems-f912cf714f53)
Atomic design layers are as follows:
- Sub-atomic: The sub-atomic layers include all the variables and settings that will be used within the application. These are not going to be “components,” but just CSS variables that will be shared globally within our application. In the sub-atomic layer, we find colors, typography, and spacing.
- Atoms: These are components that will define individual HTML elements, so, for example, a button, an icon, and an input text are all part of atoms.
- Molecules: Molecules are made up of two or more atoms or plain HTML elements. For example, an input field with a label and an error is a molecule.
- Organism: There are UI components that make up a standalone section that can be used on the site. For example, a login form is an organism, a slideshow is an organism, and so is a footer.
- Templates: These are commonly called layouts within the frontend ecosystem and are used to define a reusable structure used by multiple pages. An example could be a template with a hero image, a sidebar, main content area and a footer. This template would be used by many pages within the application and abstracting it into its own template reduces duplication.
- Pages: Lastly, we have pages. These are used to define our web application page or subpage. A page is going to be the place in which our data is loaded, and it will include HTML elements, organisms, molecules, and atoms.
Even if this separation may seem complicated to understand from the preceding description, we will touch base on this topic multiple times during the book and this will help you understand the main difference between the layers available.
Spend some time going through the folder structure of the application and read the components’ names to try and understand how we will break up our application.
Separation of concern
So far, we have learned that the modern framework offers the ability to break up the application into small chunks called components and that there is a hierarchy within the component itself.
In this section, we are going to quickly touch base on why this hierarchy was introduced in the first place and understand how this is going to help our development.
Atomic design not only supports us in breaking up components by their visual complexity, but it also helps us to break up the application logic to create highly performance and scalable applications.
As the component definitions get more complex, so does the logic expected to be attached to it.
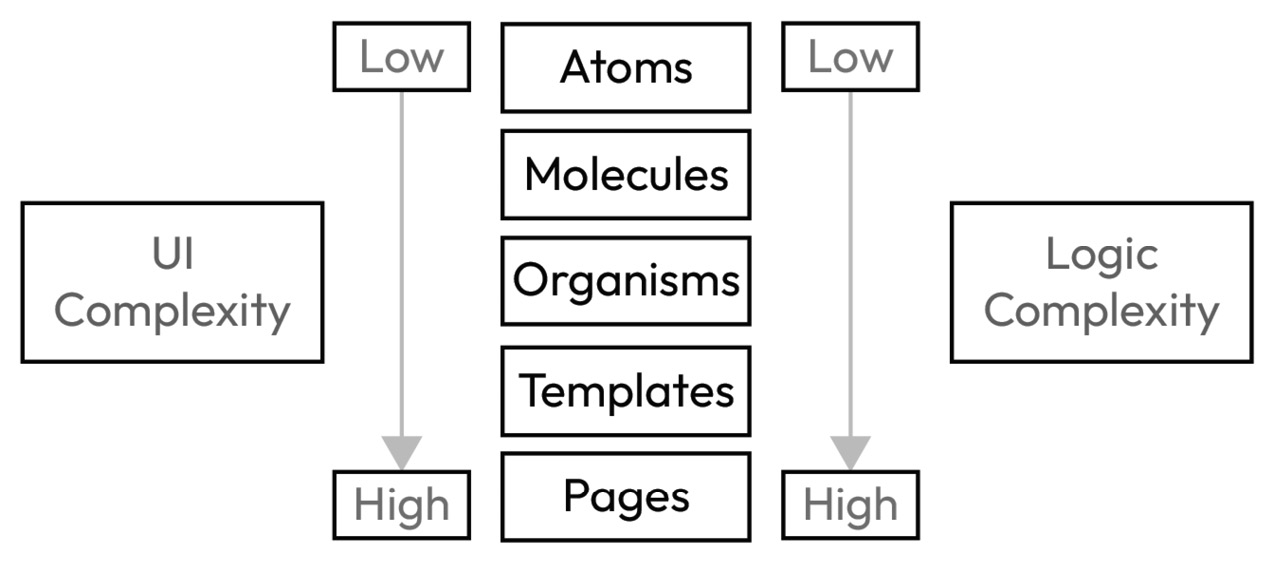
Figure 1.5: Illustration of the level of UI and Logic complexity for each layer
What do we mean by logic complexity? Logic complexity can be described as the amount of JS required for the component to function correctly.
For example, a component with low logic complexity such as a button will have very limited JS, while a more complex component such as an input field will need to handle field validation and error placement; furthermore, a page will have to take ownership of loading the data from the API, format the data to ensure that it is in the right format, and handle the visibility of its children.
In this section, we have introduced how an application is structured using the component-based architecture. We introduced the different layers that make up a component library and finally defined the advantages that this methodology when used in conjunction with a frontend framework such as Vue.js. Let’s now recap the chapter in the Summary section.