Introducing this book and its contents
Before we dive into an overview of this book, let’s set the context by understanding that this is one of three books about .NET 8 that I have written that cover almost everything a beginner to .NET needs to know.
Companion books to complete your learning journey
This book is the third of three books in a trilogy that completes your learning journey through .NET 8:
- The first book, C# 12 and .NET 8 – Modern Cross-Platform Development Fundamentals, covers the fundamentals of the C# language, the .NET libraries, and using ASP.NET Core and Blazor for web development. It is designed to be read linearly because skills and knowledge from earlier chapters build up and are needed to understand later chapters.
- The second book, Apps and Services with .NET 8, covers more specialized .NET libraries like internationalization and popular third-party packages including Serilog and Noda Time. You learn how to build native ahead-of-time (AOT)-compiled services with ASP.NET Core Minimal APIs and how to improve performance, scalability, and reliability using caching, queues, and background services. You implement more services using GraphQL, gRPC, SignalR, and Azure Functions. Finally, you learn how to build graphical user interfaces for websites, desktop, and mobile apps with Blazor and .NET MAUI.
- This third book covers important tools and skills that a professional .NET developer should have. These include design patterns and solution architecture, debugging, memory analysis, all the important types of testing, whether it be unit, integration, performance, or web user interface testing, and then topics for testing cloud-native solutions on your local computer like containerization, Docker, and .NET Aspire. Finally, we will look at how to prepare for an interview to get the .NET developer career that you want.
A summary of the .NET 8 trilogy and their most important topics is shown in Figure 1.1:
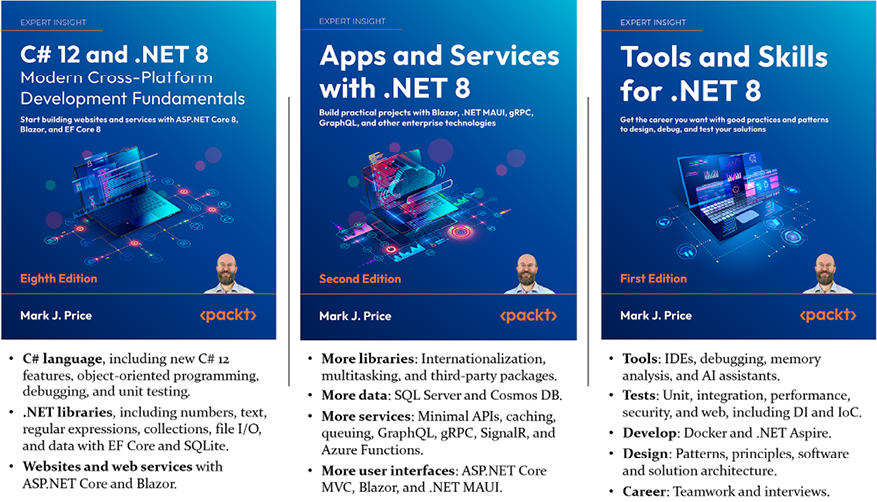
Figure 1.1: Companion books for learning .NET 8 for beginner-to-intermediate readers
We provide you with a PDF file that has color images of the screenshots and diagrams used in this book. You can download this file from https://packt.link/gbp/9781837635207.
Audiences for this book
This book caters to two audiences:
- Readers who have completed my book for learning the fundamentals of the C# language, .NET libraries, and using ASP.NET Core for web development, C# 12 and .NET 8 – Modern Cross-Platform Development Fundamentals, and now want to take their learning further.
- Readers who already have basic skills and knowledge about C# and .NET and want to acquire practical skills and knowledge of common tools to become more professional with their .NET development, and in particular join a team of .NET developers.
Let’s look at an analogy:
- First, an amateur cook might buy a book to learn fundamental skills, concepts, and terminology that any cook needs to make the most common dishes.
- Second, an amateur cook might also buy a recipe book to learn how to apply that knowledge and those skills to make complete meals.
- Third, to become a professional cook, they would also need to understand the roles in a professional kitchen, learn more specialized tools and skills that are needed when cooking meals for many more people in a professional environment, and how to work in a team of cooks.
These three scenarios are why I wrote three books about .NET.
The preface briefly introduces each chapter, but many readers skip the preface. So, let’s now review why each topic is covered in more depth.
Tools
There are many tools that a professional .NET developer should be familiar with. Some are built into most code editors like a debugger or source control integration, and some require separate applications and services like memory analysis and telemetry.
Even beginner developers know the basic tools included with a code editor like the main editing window, managing files in a project, how to set a breakpoint and start debugging, and then step through the code statement by statement, and how to run a project, so those topics will not be covered in this book.
Chapter 2, Making the Most of the Tools in your Code Editor, is about the less commonly used tools built into Visual Studio, Code, and Rider. The major tools like a debugger or memory analysis tools are covered in separate later chapters. This chapter covers topics like refactoring features and how to customize your code editor using standards like .editorconfig
.
Chapter 3, Source Code Management Using Git, covers the most common tasks that you would perform with Git to manage your source code, especially when working in a team of .NET developers. Git is a distributed source control system, so developers have a local copy of the entire repository. This enables offline work, fast branching, and merging. Git is the most popular source code control system for .NET projects, and there are tools and extensions available for seamless integration with all code editors and command-line tools. GitHub is a popular Microsoft platform for hosting Git repositories and collaborating on software projects.
Chapter 4, Debugging and Memory Troubleshooting, is about using the debugging tools in your code editor. You will learn how to use the built-in debugging features, how to decorate your own code with attributes to make it easier to see what’s happening while debugging, and how to use tools in your code editor to track the usage of memory to improve your apps and services.
Chapter 5, Logging, Tracing, and Metrics for Observability, is about how to instrument your code to enable tracing, metrics, and logging during production, and how to implement telemetry using OpenTelemetry to monitor your code as it executes to enable observability.
Skills
As well as learning how to use the tools themselves, a professional .NET developer needs skills like documenting code, leveraging dynamic code, and implementing cryptographic techniques to protect code and data. You will also build a custom chatbot that uses a model enhanced with custom functions.
Chapter 6, Documenting Code, APIs, and Services, discusses how to best document your code to help other developers maintain it in the future using comments, and how to document your services and APIs to enable other developers to call them as designed. Forcing yourself to document your code has the side benefit that you will often spot places where you could improve the design and refactor your code and APIs.
Chapter 7, Observing and Modifying Code Execution Dynamically, introduces you to some common types that are included with .NET for performing code reflection, applying and reading attributes, working with expression trees, and most usefully, creating source generators.
Chapter 8, Protecting Data and Apps Using Cryptography, is about protecting your data from being viewed by malicious users using encryption, and from being manipulated or corrupted using hashing and signing. You will also learn about using authentication and authorization to protect applications from unauthorized users.
Chapter 9, Building a Custom LLM-based Chat Service, covers how to build a custom chat service that integrates a large language model (LLM) and custom functions based on business data.
Chapter 10, Dependency Injection, Containers and Service Lifetime, is about reducing tight coupling between components, which is especially critical to performing practical testing. This also enables you to better manage changes and software complexity.
Testing
One of the most important tools and skills for a .NET developer is testing to ensure quality. Testing spans the whole life cycle of development, from testing a small unit of behavior to end-to-end testing of the user experience and system integration. The earlier in the development process that you test, the lower the cost of fixing any mistakes that you discover.
Chapter 11, Unit Testing and Mocking, introduces testing practices that will improve your code quality. Unit testing is easy to get wrong and can become useless, undermining the team’s trust. Get it right, and you will save time and money and smooth out the development process.
Chapter 12, Integration and Security Testing, introduces a higher level of testing across all components of a solution, and the types of testing needed to maintain the security of your projects.
Chapter 13, Benchmarking Performance, Load, and Stress Testing, introduces how to properly use the BenchmarkDotNet library to monitor your code to measure performance and efficiency. Then, you will see how to perform load and stress testing on your projects to predict required resources and estimate the costs of deployment in production using Bombardier and NBomber.
Chapter 14, Functional and End-to-End Testing of Websites and Services, introduces you to testing APIs and web user interfaces using Playwright for automation.
Chapter 15, Containerization Using Docker, introduces you to the concept of containerization and specifically using Docker to virtualize hosts for services in complex solution architectures.
Chapter 16, Cloud-Native Development Using .NET Aspire, introduces you to .NET Aspire, an opinionated way to manage a cloud-native development environment. It automates local deployments during development and includes integrations with tools you learned about earlier in the book like OpenTelemetry for observability, Docker containers for microservices, and so on.
Design and career development
The final part of this book is about more theoretical concepts like design patterns, principles, and software and solution architecture, and ends with a chapter that wraps everything up with preparing for an interview to get the career that you want with a team of developers and other professionals.
Chapter 17, Design Patterns and Principles, introduces you to the SOLID design patterns as well as other common patterns and principles like DRY (Don’t Repeat Yourself), KISS (Keep It Simple, Stupid), YAGNI (You Ain’t Gonna Need It), and PoLA (Principle of Least Astonishment).
Chapter 18, Software and Solution Architecture Foundations, covers software and solution architecture, including using Mermaid to create architecture diagrams.
Chapter 19, Your Career, Teamwork, and Interviews, covers what you need to get the career you aspire to. To achieve this, you must impress with your resume and at an interview. This chapter covers commonly asked questions in interviews and suggested answers that fit with your experience to help you give realistic responses.