Operations in a Computational Graph
Now that we can put objects into our computational graph, we will introduce operations that act on such objects.
Getting ready
To start a graph, we load TensorFlow and create a session, as follows:
import tensorflow as tf sess = tf.Session()
How to do it…
In this example, we will combine what we have learned and feed in each number in a list to an operation in a graph and print the output:
First we declare our tensors and placeholders. Here we will create a
numpy
array to feed into our operation:import numpy as np x_vals = np.array([1., 3., 5., 7., 9.]) x_data = tf.placeholder(tf.float32) m_const = tf.constant(3.) my_product = tf.mul(x_data, m_const) for x_val in x_vals: print(sess.run(my_product, feed_dict={x_data: x_val})) 3.0 9.0 15.0 21.0 27.0
How it works…
Steps 1 and 2 create the data and operations on the computational graph. Then, in step 3, we feed the data through the graph and print the output. Here is what the computational graph looks like:
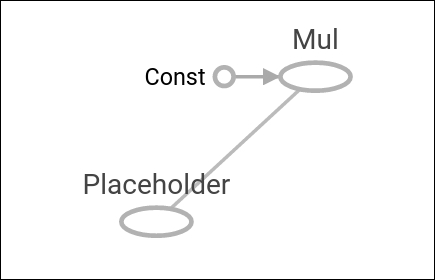
Figure...