Creating the matchmaking app
In this project, we will learn more about creating reusable controls that can be added to an Extensible Application Markup Language (XAML) page. To keep things simple, we will not be using MVVM, but bare-metal .NET MAUI without any data binding. What we aim to create is an app that allows the user to swipe images, either to the right or the left, just as most popular matchmaking applications do.
Well, let’s get started by creating the project!
Setting up the project
This project, like all the rest, is a File | New | Project...-style project. This means that we will not be importing any code at all. So, this first section is all about creating the project and setting up the basic project structure.
Let’s get started!
Creating the new project
So, let’s begin.
The first step is to create a new .NET MAUI project:
- Open Visual Studio 2022 and select Create a new project:
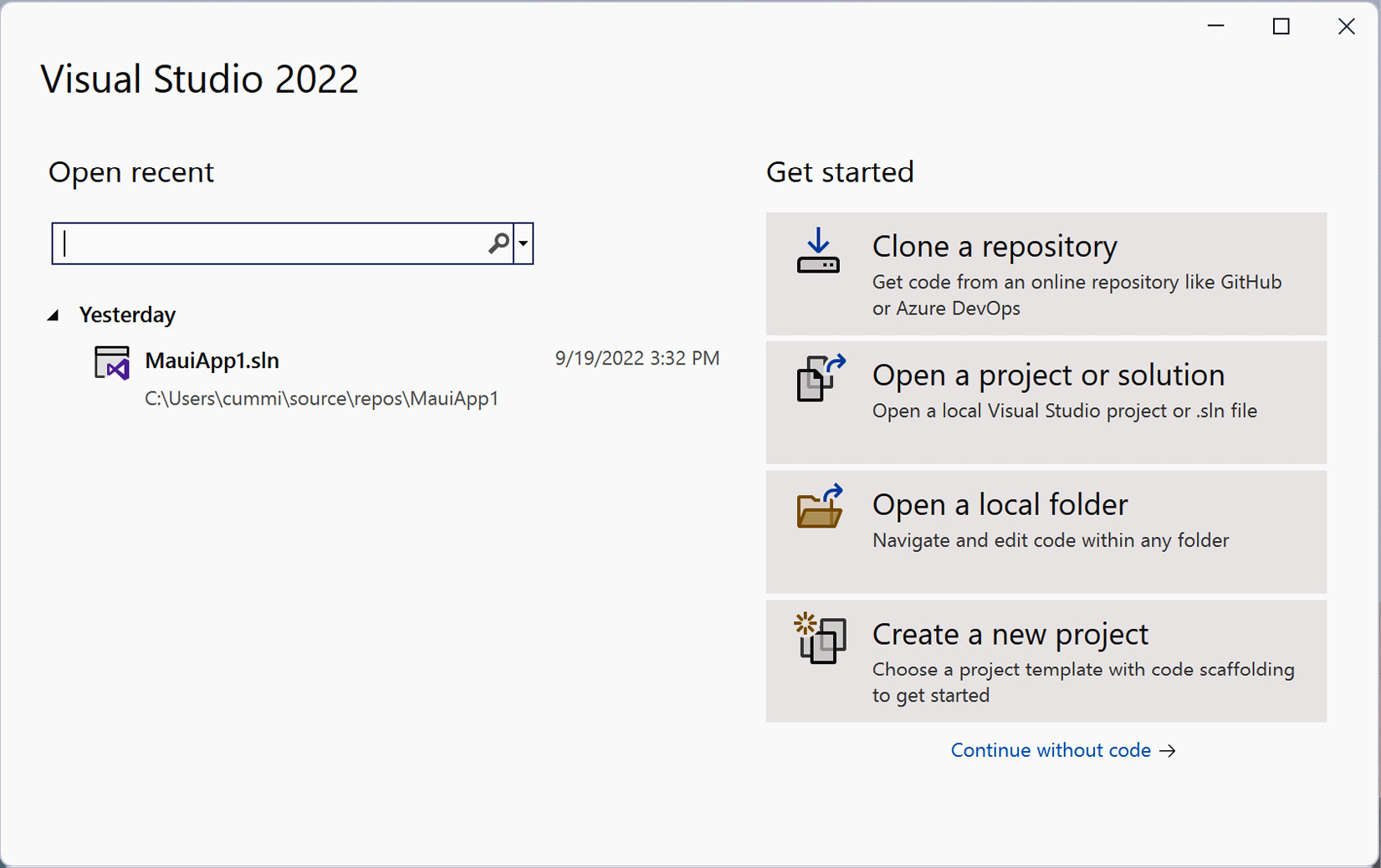
Figure 5...