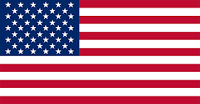

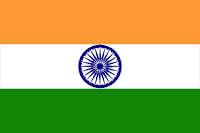
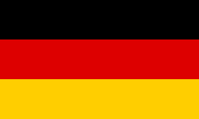
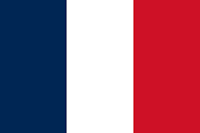

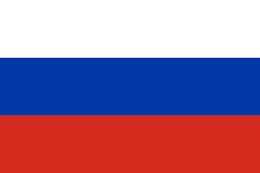
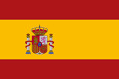



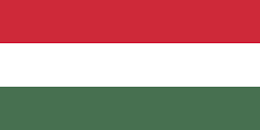

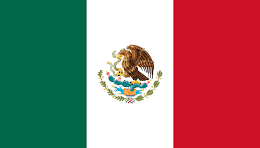
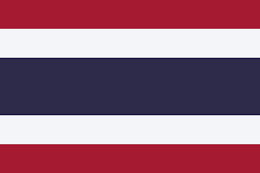
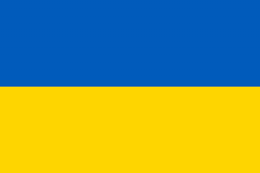
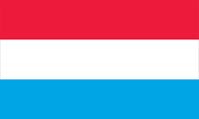

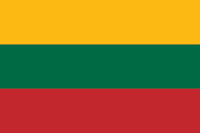

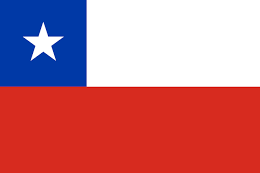

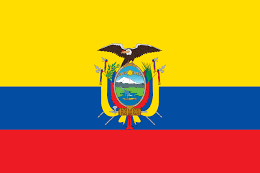

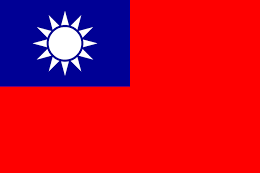

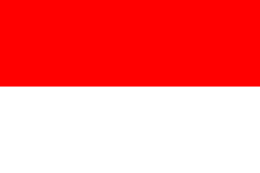
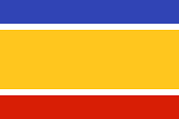
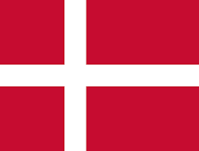
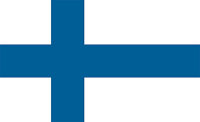


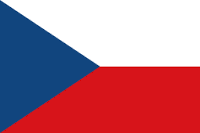
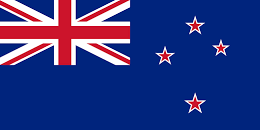
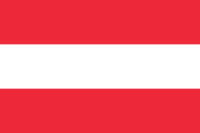
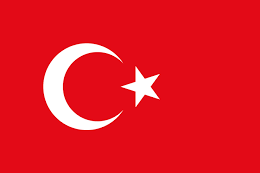
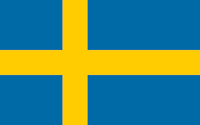
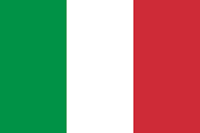
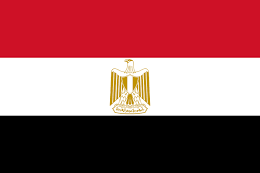

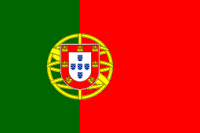
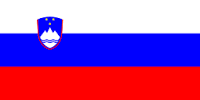

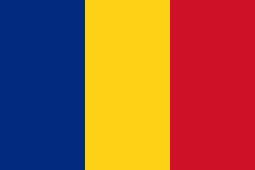
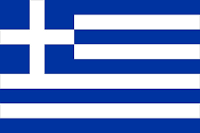

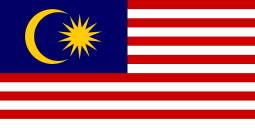
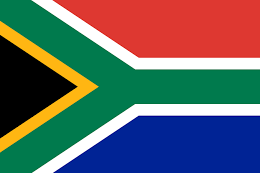

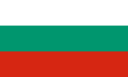
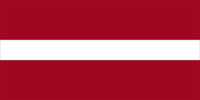


The llm-chain
is a Rust library designed to make your experience with large language models (LLMs) smoother and more powerful. In this tutorial, we'll walk you through the steps of installing Rust, setting up a new project, and getting started with the versatile capabilities of LLM-Chain.
This guide will break down the process step by step, using simple language, so you can confidently explore the potential of LLM-Chain in your projects.
Before we dive into the exciting world of LLM-Chain, let's start with the basics. To begin, you'll need to install Rust on your computer. By using the official Rust toolchain manager called rustup you can ensure you have the latest version and easily manage your installations. We recommend having Rust version 1.65.0 or higher. If you encounter errors related to unstable features or dependencies requiring a newer Rust version, simply update your Rust version. Just follow the instructions provided on the rustup website to get Rust up and running.
With Rust now installed on your machine, let's set up a new project. This step is essential to create an organized space for your work with LLM-Chain. To do this, you'll use a simple command-line instruction. Open up your terminal and run the following command:
cargo new --bin my-llm-project
By executing this command, a new directory named "my-llm-project
" will be created. This directory contains all the necessary files and folders for a Rust project.
Now that you have your Rust project folder ready, it's time to integrate the capabilities of LLM-Chain. This library simplifies your interaction with LLMs and empowers you to create remarkable applications. Adding LLM-Chain to your project is a breeze. Navigate to your project directory by using the terminal and run the following command:
cd my-llm-project
cargo add llm-chain
By running this command, LLM-Chain will become a part of your project, and the configuration will be recorded in the "Cargo.toml
" file.
LLM-Chain offers flexibility by supporting multiple drivers for different LLMs. For the purpose of simplicity and a quick start, we'll be using the OpenAI driver in this tutorial. You'll have the choice between the LLAMA driver, which runs a LLaMA LLM on your machine, and the OpenAI driver, which connects to the OpenAI API.
To choose the OpenAI driver, execute this command:
cargo add llm-chain-openai
In the next section, we'll explore generating your very first LLM output using the OpenAI driver. So, let's move on to exploring sequential chains with Rust and uncovering the possibilities they hold with LLM-Chain.
In the realm of LLM-Chain, sequential chains empower you to orchestrate a sequence of steps where the output of each step seamlessly flows into the next. This hands-on section serves as your guide to crafting a sequential chain, expanding its capabilities with additional steps, and gaining insights into best practices and tips that ensure your success.
Let's kick things off by preparing our project environment:
As we delve into creating sequential chains, one crucial prerequisite is the installation of tokio
in your project. While this tutorial uses the full tokio
package crate, remember that in production scenarios, it's recommended to be more selective about which features you install. To set the stage, run the following command in your terminal:
cargo add tokio --features full
This step ensures that your project is equipped with the necessary tools to handle the intricate tasks of sequential chains. Before we continue, ensure that you've set your OpenAI API key in the OPENAI_API_KEY
environment variable. Here's how:
export OPENAI_API_KEY="YOUR_OPEN_AI_KEY"
With your environment ready, let’s look at the full implementation code. In this case, we will be implementing the use of Chains to generate recommendations of cities to travel to, formatting them, and organizing the results throughout a series of steps:
use llm_chain::parameters;
use llm_chain::step::Step;
use llm_chain::traits::Executor as ExecutorTrait;
use llm_chain::{chains::sequential::Chain, prompt};
use llm_chain_openai::chatgpt::Executor;
#[tokio::main(flavor = "current_thread")]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
// Create a new ChatGPT executor with default settings
let exec = Executor::new()?;
// Create a chain of steps with two prompts
let chain: Chain = Chain::new(vec![
// First step: Craft a personalized birthday email
Step::for_prompt_template(
prompt!("You are a bot for travel assistance research",
"Find good places to visit in this city {{city}} in this country {{country}}. Include their name")
),
// Second step: Condense the email into a tweet. Notably, the text parameter takes the output of the previous prompt.
Step::for_prompt_template(
prompt!(
"You are an assistant for managing social media accounts for a travel company",
"Format the information into 5 bullet points for the most relevant places. \\\\n--\\\\n{{text}}")
),
// Third step: Summarize the email into a LinkedIn post for the company page, and sprinkle in some emojis for flair.
Step::for_prompt_template(
prompt!(
"You are an assistant for managing social media accounts for a travel company",
"Summarize this email into a LinkedIn post for the company page, and feel free to use emojis! \\\\n--\\\\n{{text}}")
)
]);
// Execute the chain with provided parameters
let result = chain
.run(
// Create a Parameters object with key-value pairs for the placeholders
parameters!("city" => "Rome", "country" => "Italy"),
&exec,
)
.await
.unwrap();
// Display the result on the console
println!("{}", result.to_immediate().await?.as_content());
Ok(())
}
The provided code initiates a multi-step process using the llm_chain
and llm_chain_openai
libraries. First, it sets up a ChatGPT executor with default configurations. Next, it creates a chain of sequential steps, each designed to produce specific text outputs. The first step involves crafting a personalized travel recommendation, which includes information about places to visit in a particular city and country, with a Parameters object containing key-value pairs for placeholders like {{city}}
and {{country}}
. The second step condenses this email into a tweet, formatting the information into five bullet points and utilizing the text output from the previous step. Lastly, the third step summarizes the email into a LinkedIn post for a travel company's page, adding emojis for extra appeal.
The chain is executed with specified parameters, creating a Parameters object with key-value pairs for placeholders like "city" (set to "Rome") and "country" (set to "Italy"). The generated content is then displayed on the console. This code represents a structured workflow for generating travel-related content using ChatGPT.
Now, it's time to compile the code and run the code. Execute the following command in your terminal:
cargo run
As the code executes, the sequential chain orchestrates the different prompts, generating content that flows through each step.
We can see the results of the model as a bulleted list of travel recommendations.
The llm-chain
Rust library serves as your gateway to accessing large language models (LLMs) within the Rust programming language. This tutorial has been your guide to uncovering the fundamental steps necessary to harness the versatile capabilities of LLM-Chain.
We began with the foundational elements, guiding you through the process of installing Rust and integrating llm-chain
into your project using Cargo. We then delved into the practical application of LLM-Chain by configuring it with the OpenAI driver, emphasizing the use of sequential chains. This approach empowers you to construct sequences of steps, where each step's output seamlessly feeds into the next. As a practical example, we demonstrated how to create a travel recommendation engine capable of generating concise posts for various destinations, suitable for sharing on LinkedIn.
It's important to note that LLM-Chain offers even more possibilities for exploration. You can extend its capabilities by incorporating CPP models like Llama, or you can venture into the realm of map-reduce chains. With this powerful tool at your disposal, the potential for creative and practical applications is virtually limitless. Feel free to continue your exploration and unlock the full potential of LLM-Chain in your projects. See you in the next article.
Alan Bernardo Palacio is a data scientist and an engineer with vast experience in different engineering fields. His focus has been the development and application of state-of-the-art data products and algorithms in several industries. He has worked for companies such as Ernst and Young, and Globant, and now holds a data engineer position at Ebiquity Media helping the company to create a scalable data pipeline. Alan graduated with a Mechanical Engineering degree from the National University of Tucuman in 2015, participated as the founder of startups, and later on earned a Master's degree from the faculty of Mathematics at the Autonomous University of Barcelona in 2017. Originally from Argentina, he now works and resides in the Netherlands.