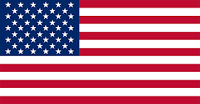

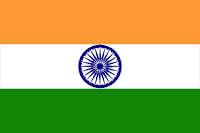
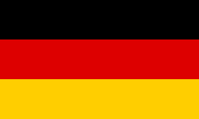
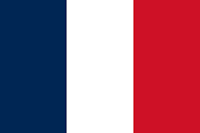

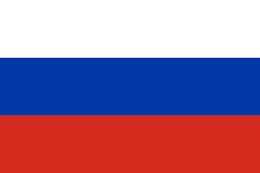
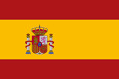



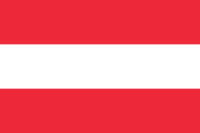

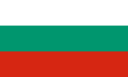
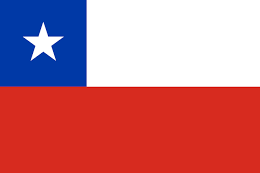

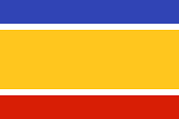
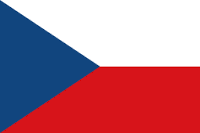
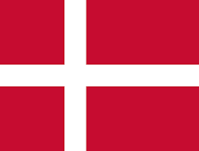
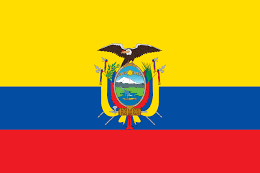
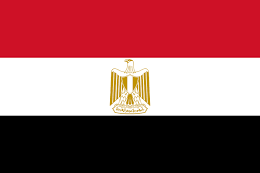

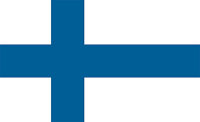
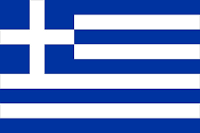
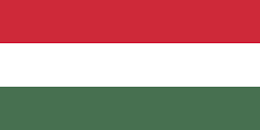
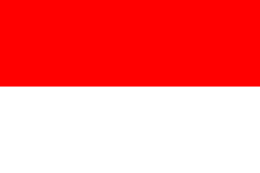

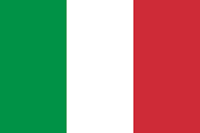

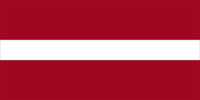
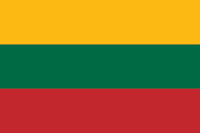
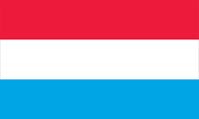
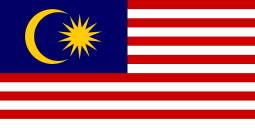

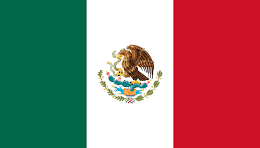

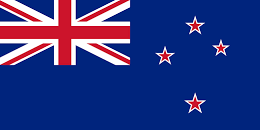



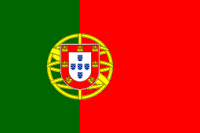
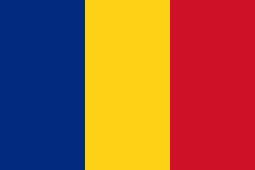


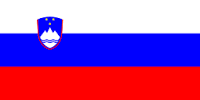
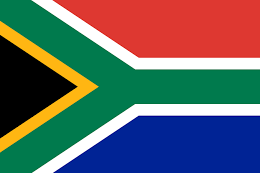

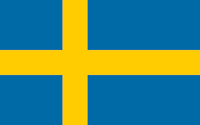

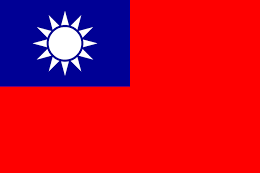
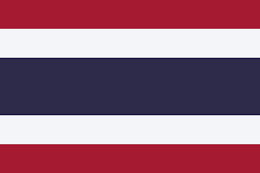
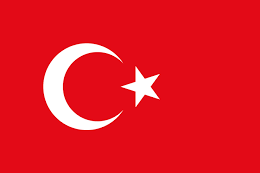
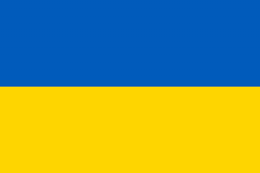
npm install body-parser --save
npm install express --save
// Two-way SMS Bot
const express = require('express')
const bodyParser = require('body-parser')
const twilio = require('twilio')
const app = express()
app.set('port', (process.env.PORT || 5000))
Chapter 5
[ 185 ]
// Process application/x-www-form-urlencoded
app.use(bodyParser.urlencoded({extended: false}))
// Process application/json
app.use(bodyParser.json())
// Spin up the server
app.listen(app.get('port'), function() {
console.log('running on port', app.get('port'))
})
// Index route
app.get('/', function (req, res) {
res.send('Hello world, I am SMS bot.')
})
//Twilio webhook
app.post('/sms/', function (req, res) {
var botSays = 'You said: ' + req.body.Body;
var twiml = new twilio.TwimlResponse();
twiml.message(botSays);
res.writeHead(200, {'Content-Type': 'text/xml'});
res.end(twiml.toString());
})
The preceding code creates a web app that looks for incoming messages from users and responds to them. The response is currently to repeat what the user has said.
git add .
git commit -m webapp
git push heroku master
Now we have a web app on the cloud at https://ms-notification-bot.herokuapp.com/sms/ that can be called when an incoming SMS message arrives. This app will generate an appropriate chatbot response to the incoming message.
Now all the inbound messages will be routed to this web app.
In Advanced settings, we can add multiple numbers for serving different geographic regions and have them respond as if the chatbot is responding over a local number.
Congratulations! You now have a two-way interactive chatbot.
This tutorial is an excerpt from the book, Hands-On Chatbots and Conversational UI Development written by Srini Janarthanam. If you found our post useful, do check out this book to get real-world examples of voice-enabled UIs for personal and home assistance.
How to build a basic server side chatbot using Go
Build a generative chatbot using recurrent neural networks (LSTM RNNs)
Top 4 chatbot development frameworks for developers