Developing weather display applications
Now that we are experienced IoT application developers, we are ready to take our skills to the next level and create more intricate projects. In this section, we will leverage the capabilities of Raspberry Pi and Sense HAT to create a weather display application and a weather-dependent GO-NO-GO decision-making application.
In Figure 2.13, we see a diagram depicting a call to the OpenWeather API from our Raspberry Pi and Sense HAT, enclosed within its custom case. For our weather display application, we will follow a similar approach to the scrolling stock ticker:
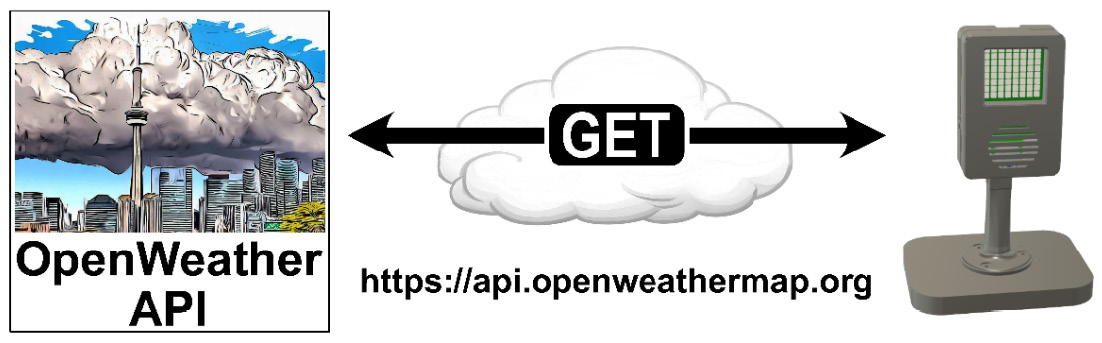
Figure 2.13 – Using the OpenWeather API to get the current weather conditions
We will first acquire an API key from OpenWeather and verify the API call by printing the response to the Shell for testing purposes. We will then utilize the Sense HAT to create a ticker-style display that displays the current weather conditions.
Finally, we will...