In the previous recipe, we loaded an ES module into an HTML page and executed an exported function. Now we can take a look at using multiple modules in a program. This allows us more flexibility when organizing our code.
Exporting/importing multiple modules for external use
Getting ready
Make sure you have Python installed and your browser properly configured.
How to do it...
- Create a new working directory, navigate into it with your command-line application, and start the Python SimpleHTTPServer.
- Create a file named rocket.js that exports the name of a rocket, a countdown duration, and a launch function:
export default name = "Saturn V"; export const COUNT_DOWN_DURATION = 10; export function launch () { console.log(`Launching in ${COUNT_DOWN_DURATION}`); launchSequence(); } function launchSequence () { let currCount = COUNT_DOWN_DURATION; const countDownInterval = setInterval(function () { currCount--; if (0 < currCount) { console.log(currCount); } else { console.log('LIFTOFF!!!'); clearInterval(countDownInterval); } }, 1000); }
- Create a file named main.js that imports from rocket.js, logs out details, and then calls the launch function:
import rocketName, {COUNT_DOWN_DURATION, launch } from './rocket.js'; export function main () { console.log('This is a "%s" rocket', rocketName); console.log('It will launch in "%d" seconds.', COUNT_DOWN_DURATION); launch(); }
- Next, create an index.html file that imports the main.js module and runs the main function:
<html> <head> <meta charset='UTF-8' /> </head> <body> <h1>Open your console.</h1> <script type="module"> import { main } from './main.js'; main(); </script> </body> </html>
- Open your browser and then the index.html file. You should see the following output:
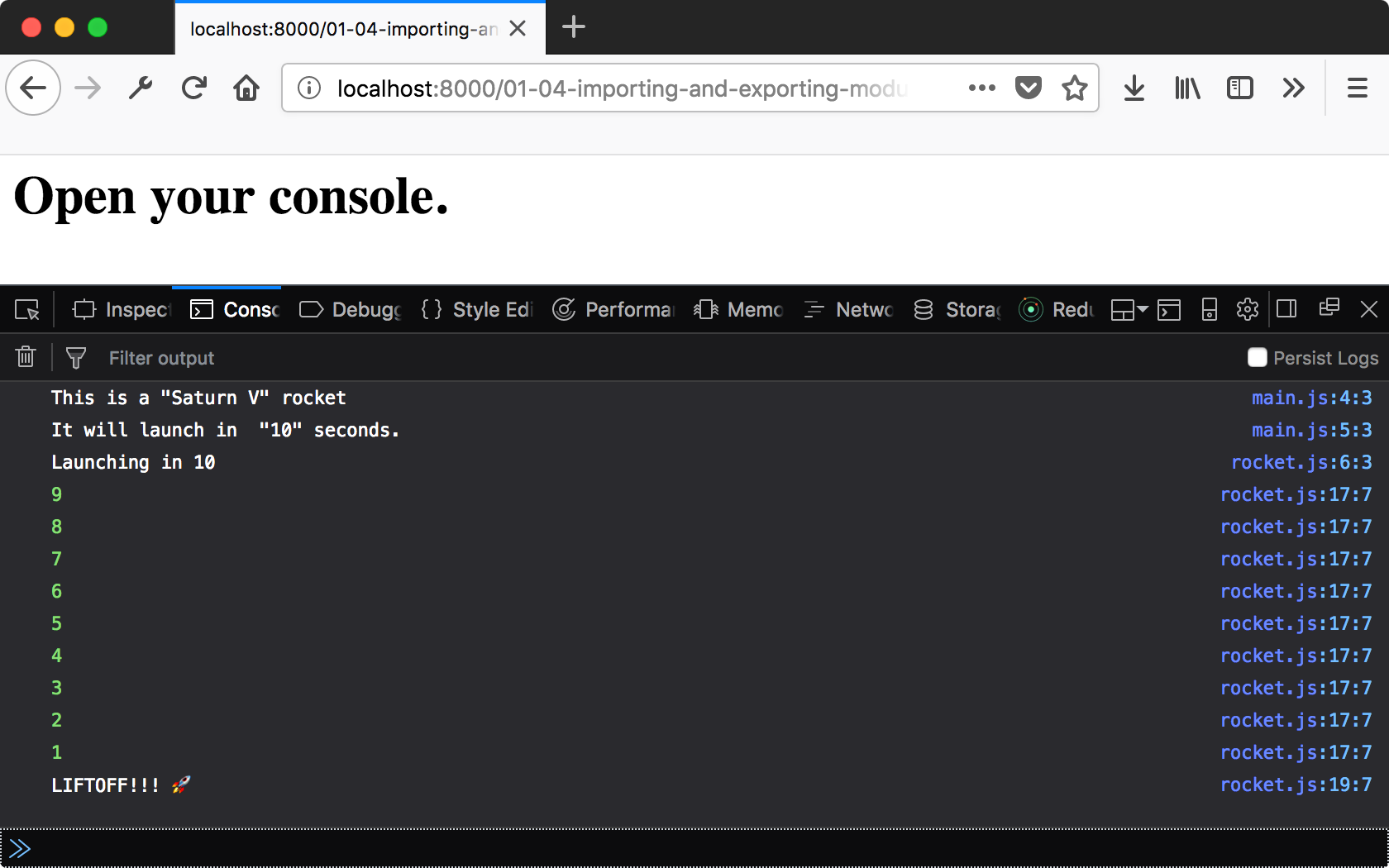
How it works...
There are two options for exporting a member from a module. It can either be exported as the default member, or as a named member. In rocket.js, we see both methods:
export default name = "Saturn V"; export const COUNT_DOWN_DURATION = 10; export function launch () { ... }
In this case, the string "Saturn V" is exported as the default member, while COUNT_DOWN_DURATION and launch are exported as named members. We can see the effect this has had when importing the module in main.js:
import rocketName, { launch, COUNT_DOWN_DURATION } from './rocket.js';
We can see the difference in how the default member and the name members are imported. The name members appear inside the curly braces, and the name they are imported with matches their name in the module source file. The default module, on the other hand, appears outside the braces, and can be assigned to any name. The unexported member launchSequence cannot be imported by another module.
See also
- Renaming imported modules
- Nesting imported modules under a single namespace