Let's start by looking at some concurrency bugs. Here is a simple example of incrementing a counter:
public class Counter {
private int counter;
public int incrementAndGet() {
++counter;
return counter;
}
This code is not thread-safe. If two threads are running using the same object concurrently, the sequence of counter values each gets is essentially unpredictable. The reason for this is the ++counter operation. This simple-looking statement is actually made up of three distinct operations, namely read the new value, modify the new value, and store the new value:
   Â
  Â
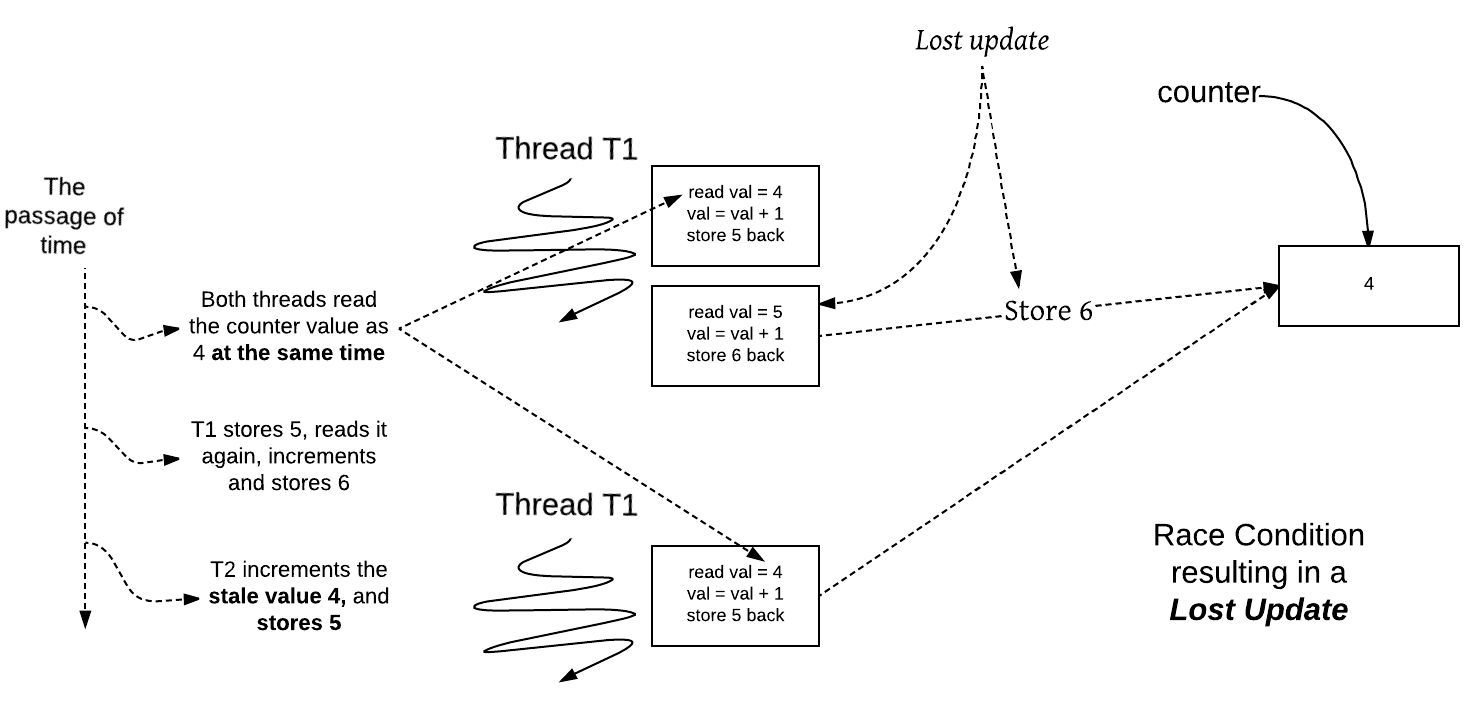
As shown in the preceding diagram, the thread executions are oblivious of each other, and hence interfere unknowingly, thereby introducing a lost update.Â
The following code illustrates the ...