27. Collecting all prime factors of a given number
A prime number is a number divisible by itself and 1 (for instance, 2, 3, and 5 are prime numbers). Having a given number, we can extract its prime factors, as in the following figure:
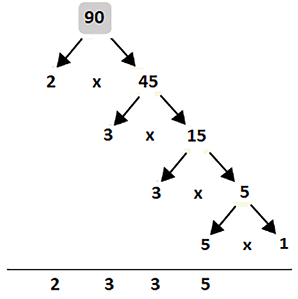
Figure 1.22: Prime factors of 90 are 2, 3, 3, and 5
The prime factors of 90 are 2, 3, 3, and 5. Based on Figure 1.22, we can create an algorithm to solve this problem, as follows:
- Define a
List
to collect prime factors of the givenv
. - Initialize a variable
s
with 2 (the smallest prime number). - If
v % s
is 0, collects
as a prime factor and compute the newv
asv / s
. - If
v % s
is not 0, then increases
by 1. - Repeat step 3 as long as
v
is greater than 1.
In code lines, this O(n) algorithm (O(log n) for composite numbers) can be expressed as follows:
public static List<Integer> factors(int v) {
List<Integer> factorsList = new ArrayList<>();
int s = 2;
while (v > 1)...