In this recipe, we will write a very simple modular Hello world program to test our JDK installation. This simple example prints Hello world in XML; after all, it's the world of web services.
Compiling and running a Java application
Getting ready
You should have JDK installed and the PATH variable updated to point to the JDK installation.
How to do it...
- Let's define the model object with the relevant properties and annotations that will be serialized into XML:
@XmlRootElement @XmlAccessorType(XmlAccessType.FIELD) class Messages{ @XmlElement public final String message = "Hello World in XML"; }
In the preceding code, @XmlRootElement is used to define the root tag, @XmlAccessorType is used to define the type of source for the tag name and tag values, and @XmlElement is used to identify the sources that become the tag name and tag values in the XML.
- Let's serialize an instance of the Message class into XML using JAXB:
public class HelloWorldXml{ public static void main(String[] args) throws JAXBException{ JAXBContext jaxb = JAXBContext.newInstance(Messages.class); Marshaller marshaller = jaxb.createMarshaller(); marshaller.setProperty(Marshaller.JAXB_FRAGMENT,Boolean.TRUE); StringWriter writer = new StringWriter(); marshaller.marshal(new Messages(), writer); System.out.println(writer.toString()); }
}
- We will now create a module named com.packt. To create a module, we need to create a file named module-info.java, which contains the module definition. The module definition contains the dependencies of the module and the packages exported by the module to other modules:
module com.packt{ //depends on the java.xml.bind module requires java.xml.bind; //need this for Messages class to be available to java.xml.bind exports com.packt to java.xml.bind; }
We will explain modules in detail in Chapter 3, Modular Programming. But this example is just to give you a taste of modular programming and to test your JDK installation.
The directory structure with the preceding files is as follows:
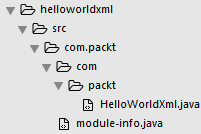
- Let's compile and run the code. From the hellowordxml directory, create a new directory in which to place your compiled class files:
mkdir -p mods/com.packt
Compile the source, HelloWorldXml.java and module-info.java, into the mods/com.packt directory:
javac -d mods/com.packt/ src/com.packt/module-info.java
src/com.packt/com/packt/HelloWorldXml.java
- Run the compiled code using java --module-path mods -m com.packt/com.packt.HelloWorldXml. You will see the following output:
<messages><message>Hello World in XML</message></messages>
Don't worry if you are not able to understand the options passed with the java or javac commands. You will learn about them in Chapter 3, Modular Programming.